TL;DR – HTML5 server-sent events (SSE) allow a web page to receive a constant stream of updates from the server without having to send XMLHttpRequests.
Contents
What are HTML5 Server-Sent Events?
To have information on your website constantly updated, you need to establish a connection with the web server. One way to do it is using XMLHttpRequests – however, they have to be re-sent to receive a response for each update.
By using HTML5 server-sent events, you can create a connection that lasts longer and receives the updates in a constant stream. Newsfeeds, social networks, sports and weather updates are all great examples of who can benefit from using HTML5 server-sent events.
Receiving and Sending SSE
To receive HTML5 updates, you need to use the EventSource
object:
<script>
if(typeof(EventSource) !== "undefined") {
var source = new EventSource("sse_demo.php");
source.onmessage = function(event) {
document.getElementById("myresult").innerHTML += event.data + "<br>";
};
} else {
document.getElementById("myresult").innerHTML = "Sorry, server-sent events are not supported in your browser...";
}
</script>
Note: in the parentheses, a PHP file called sse_demo.php is defined. A file written in a dynamic programming language is necessary to send HTML5 updates to the browser.
EventSource
opens a long-lasting connection which will only finish when you call EventSource.close()
. This connection allows the browser to receive the HTML5 server-sent events in text/event-stream media type. However, it is one-way only: the browser cannot send any data to the server.
These are all the events relevant to the EventSource
object:
Event | Description |
---|---|
open | A connection to the server is enabled |
error | A connection to the server fails |
message | A message is collected |
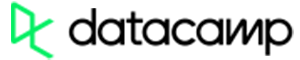
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
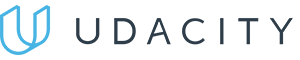
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
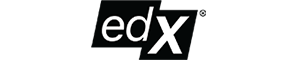
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Server Side: PHP Code Example
A server can send HTML5 updates through ASP or PHP if it is necessary. The syntax is not complicated if you have at least some basic knowledge in those languages:
<?php
header('Content-Type: text/event-stream');
header('Cache-Control: no-cache');
$time = date('r');
echo "data: The server time is: {$time}\n\n";
flush();
?>
Note: don't forget to set the Content-Type header to text/event-stream.
HTML5 Server-Sent Events: Useful Tips
- HTML5 server-sent events are a bit similar to WebSockets. However, WebSockets are more complicated to use and require a unique protocol, while SEE relies on HTTP.
- Make sure to use UTF-8 character encoding for the text data stream.
- HTML5 notifications for updates can be redirected just like any HTTP request.
Browser support
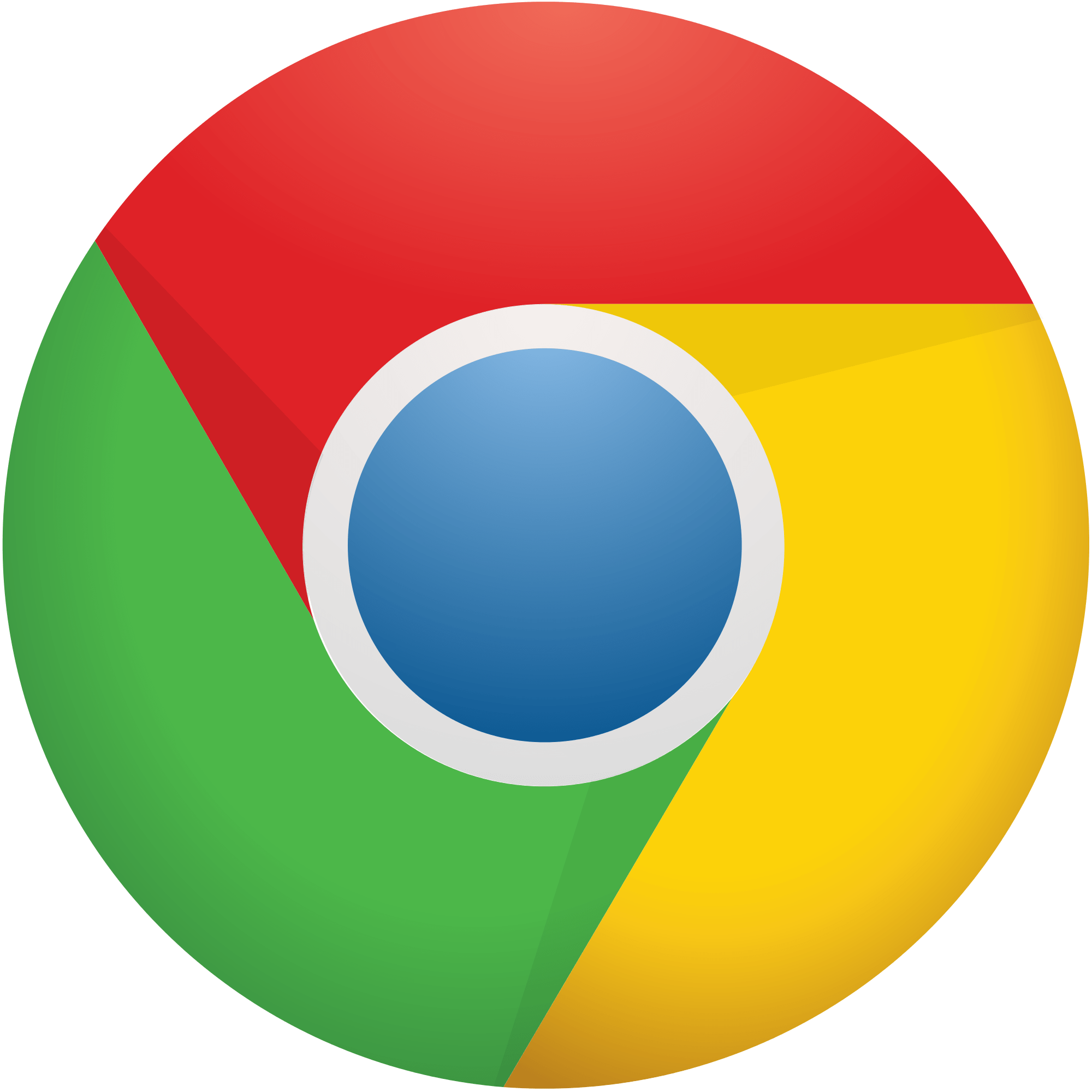
Chrome
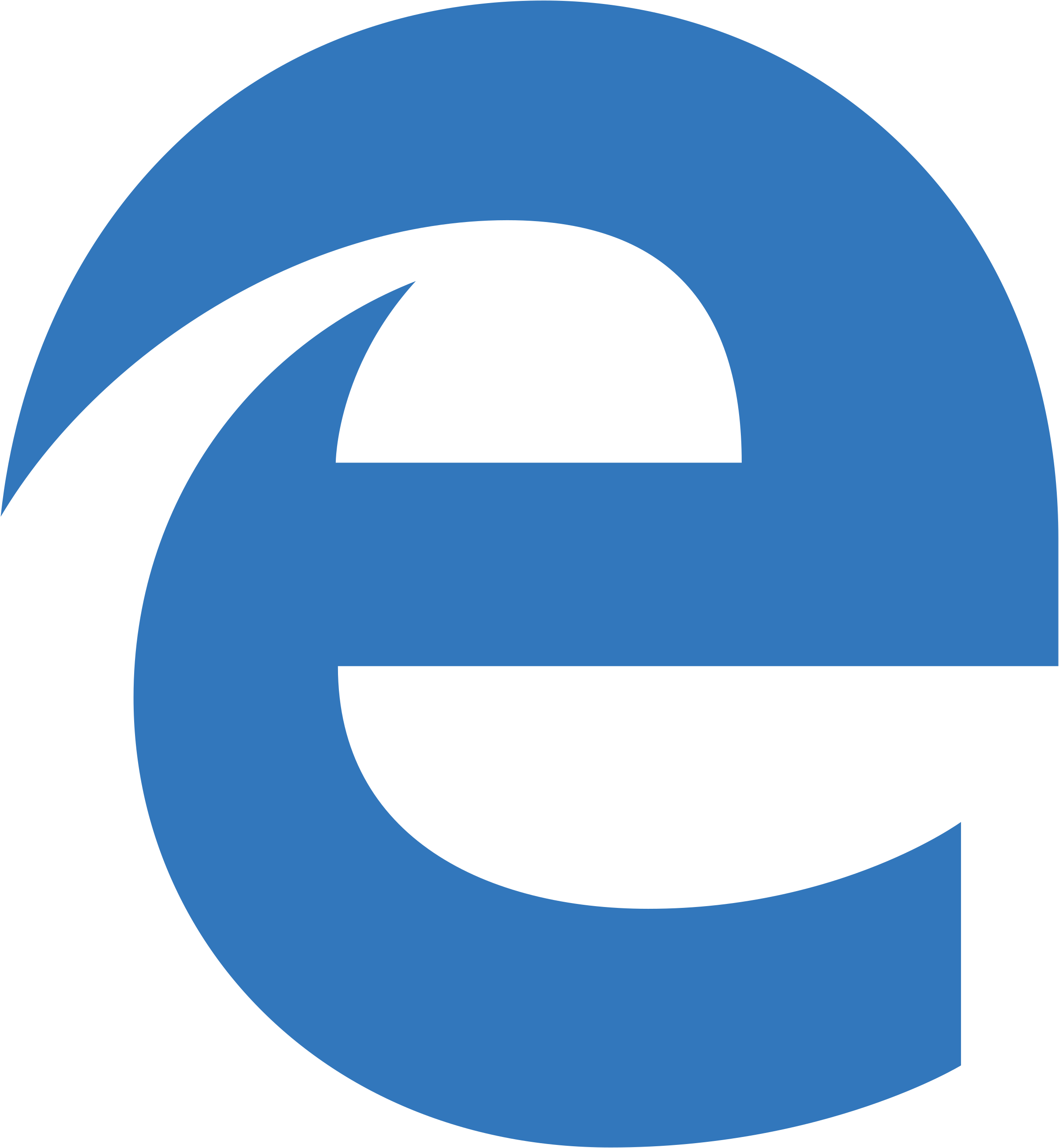
Edge
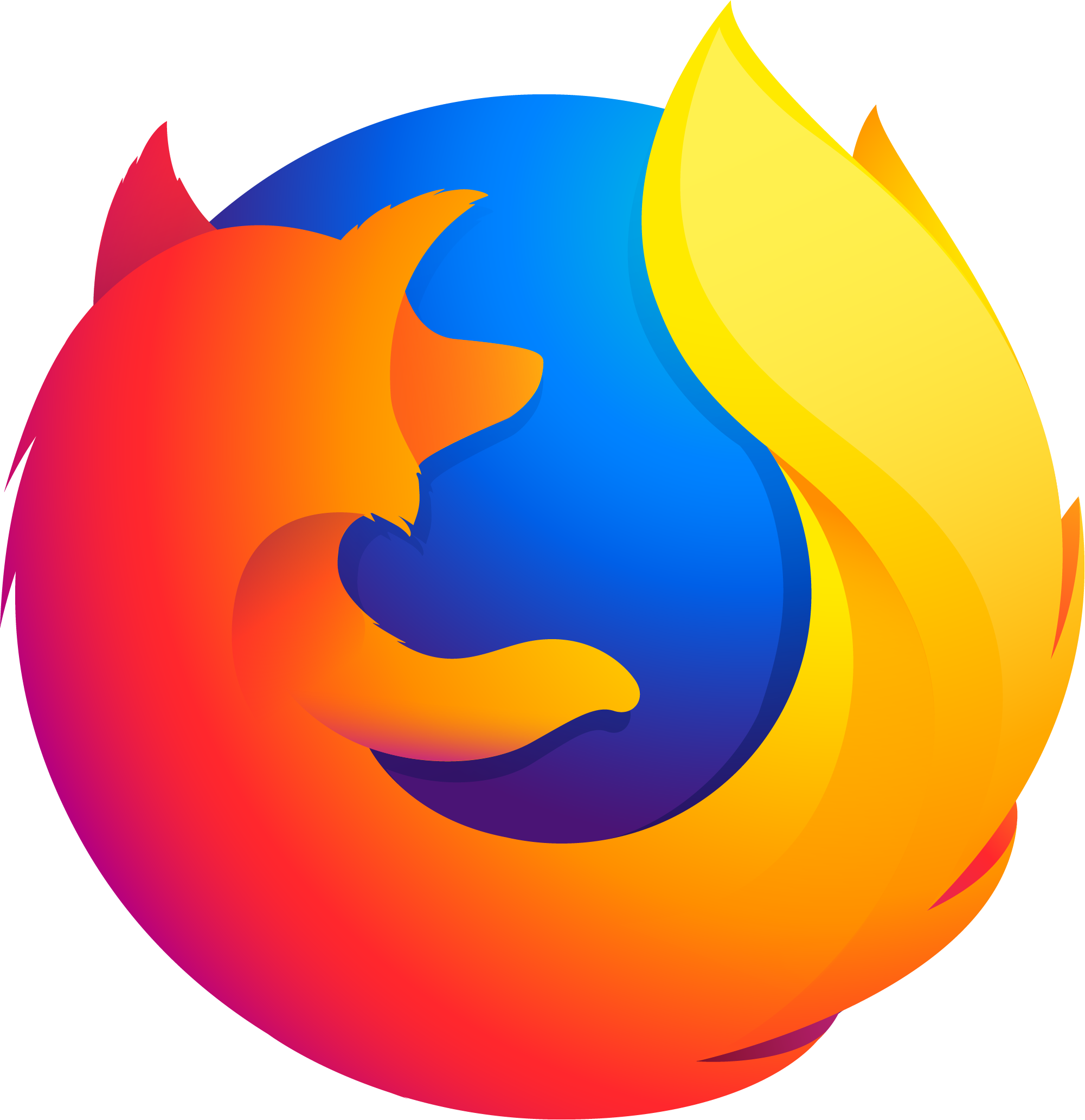
Firefox
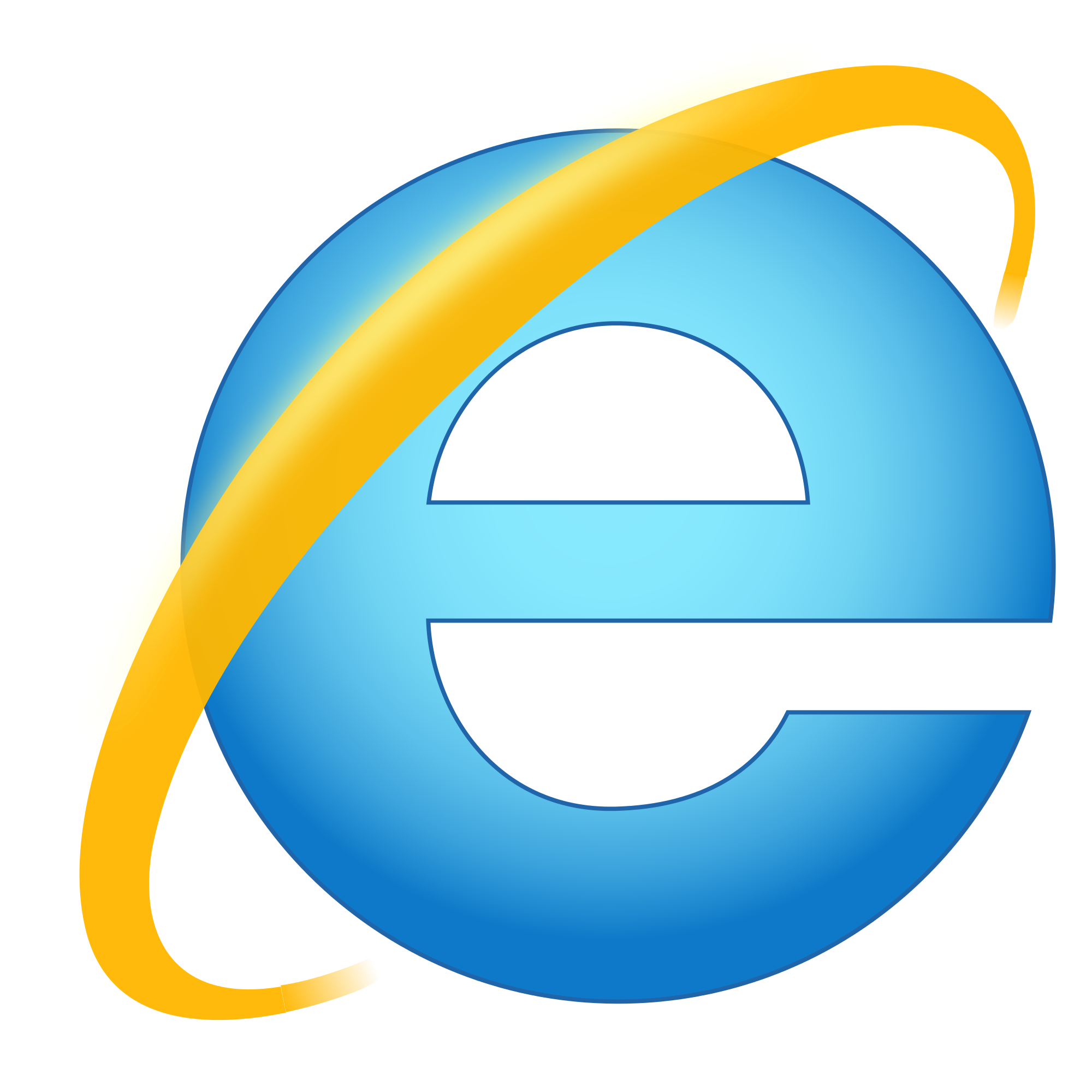
IE
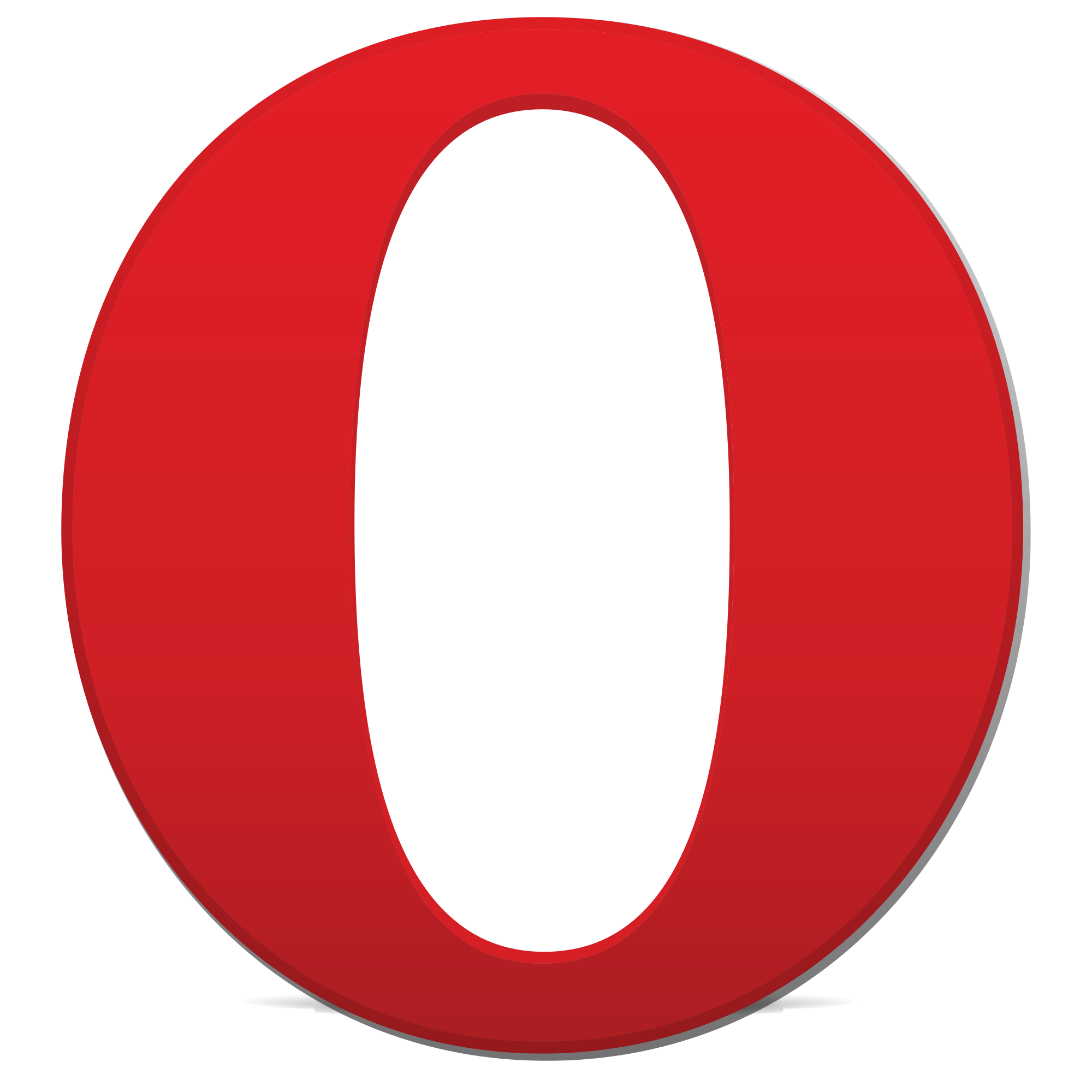
Opera
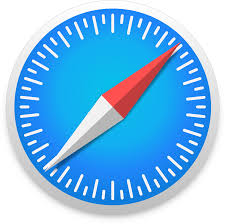
Safari
Mobile browser support
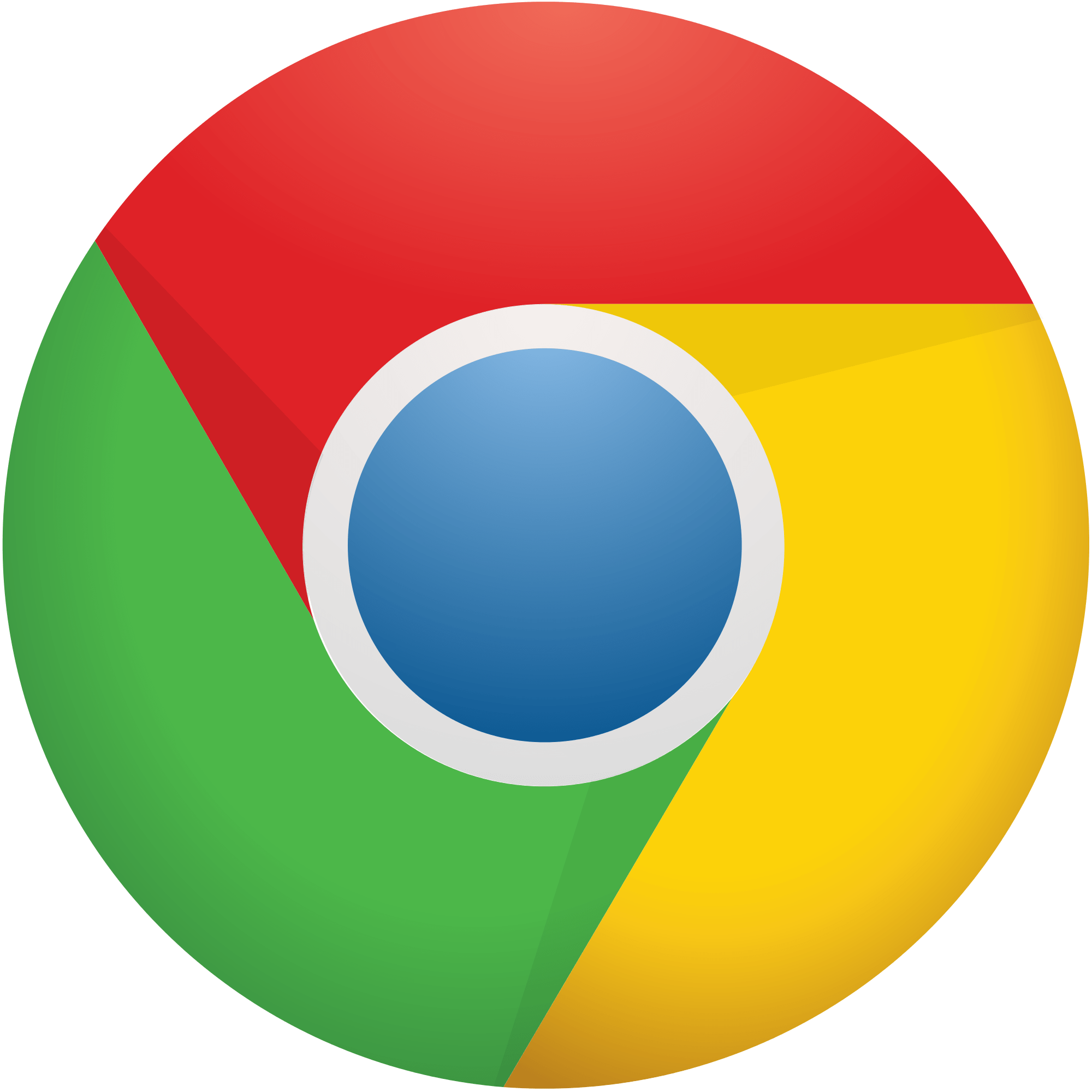
Chrome
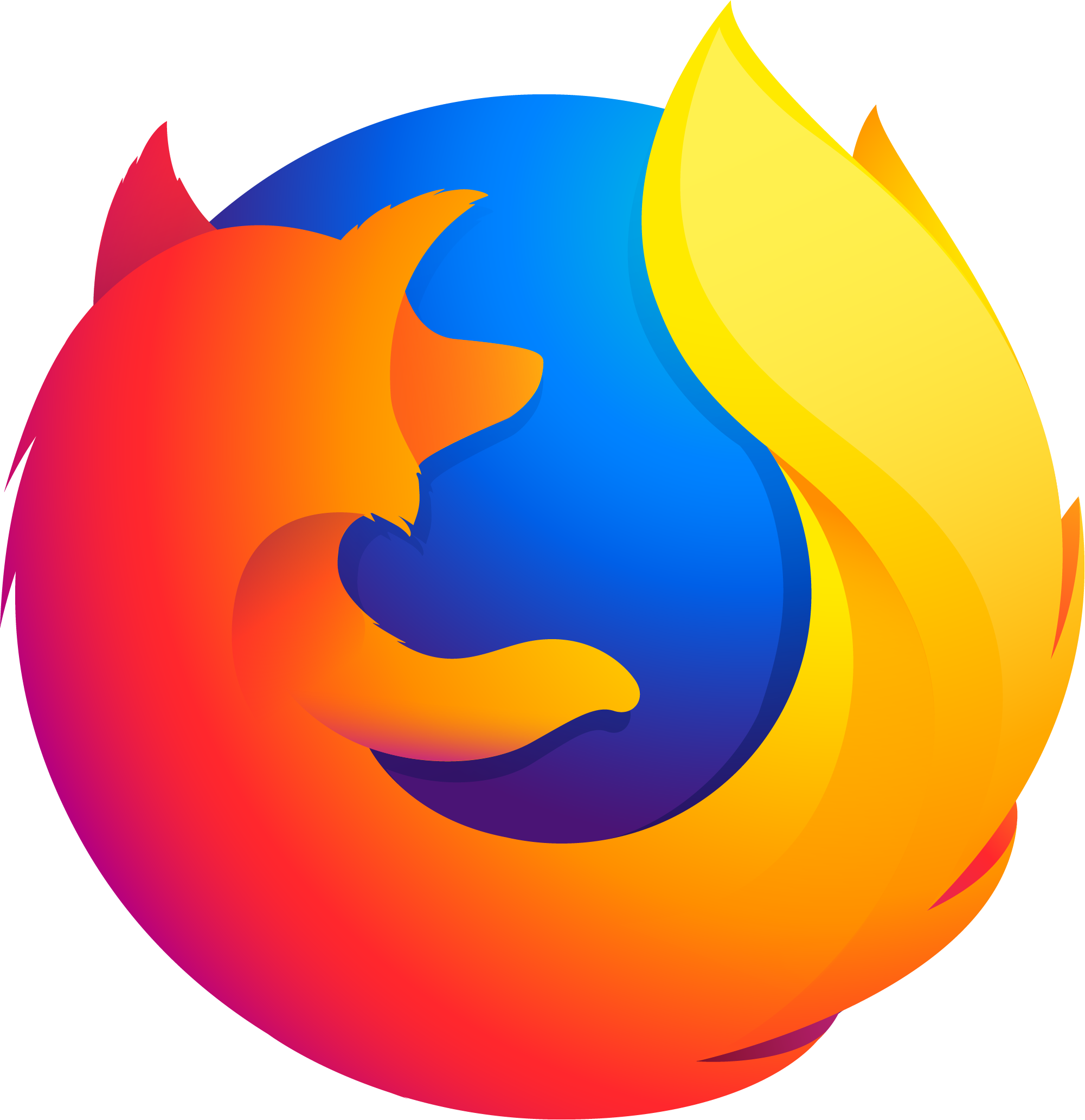
Firefox
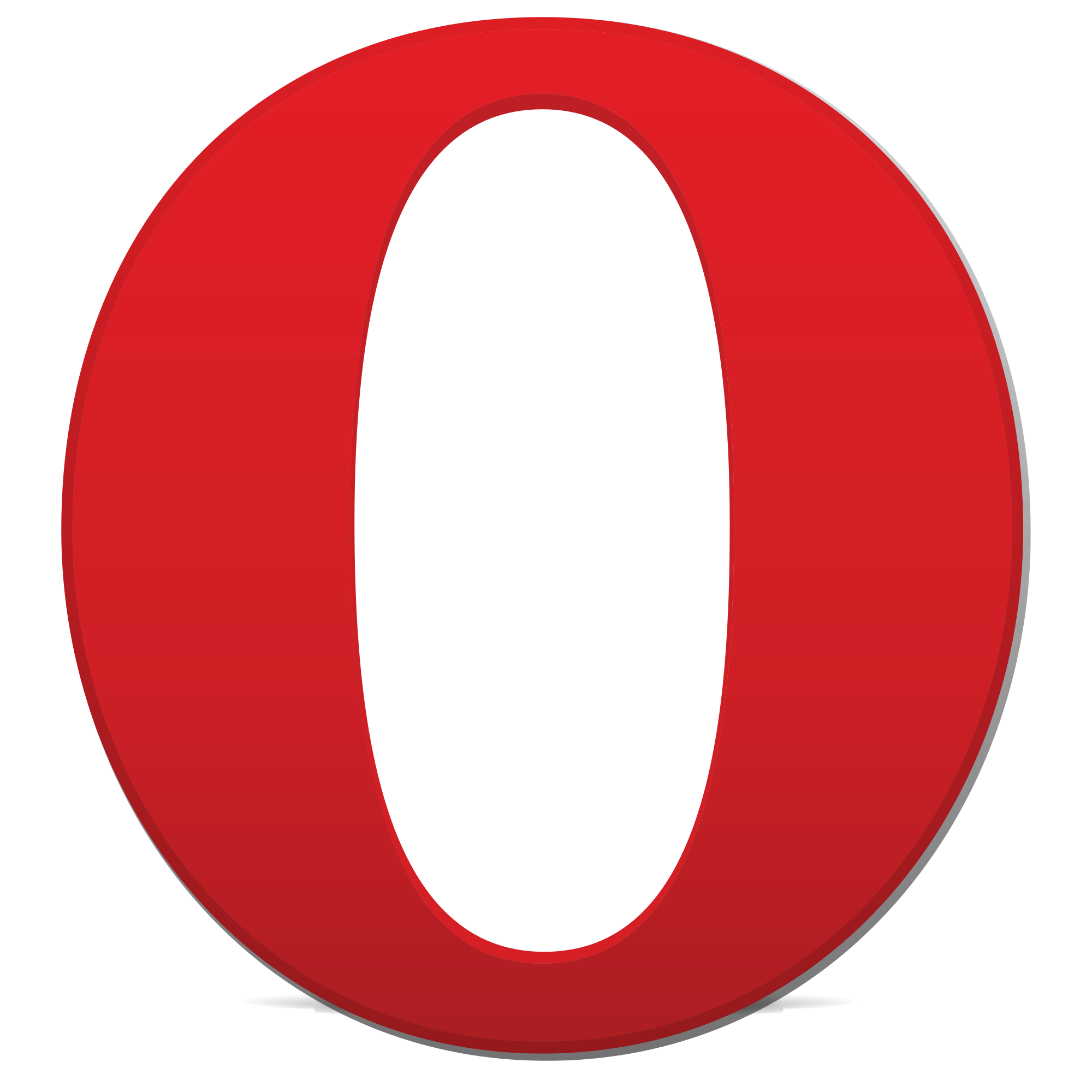
Opera
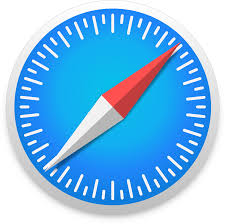