TL;DR – Web workers are JavaScript codes running in the background without disturbing web page performance.
Contents
Web Workers Explained
Using web workers in HTML5 allows you to prevent the execution of bigger tasks from freezing up your web page. A web worker performs the job in the background, independent of other scripts and thus not affecting their performance. The process is also called threading, i.e., separating the tasks into multiple parallel threads. During the time, the user can browse normally, as the page stays fully responsive.
In the example below, you can see a simple script for a web worker made for counting numbers:
var i=0;
function timedCount() {
i=i+1;
postMessage(i);
setTimeout("timedCount()", 500);
}
timedCount();
The script contains a postMessage()
method which allows posting a message (i.e., the numbers) to an HTML document. However, web workers are not usually used from such simple tasks as they wouldn't use a lot of CPU power and disturb website performance anyway.
A Full Web Worker Code
The code for web workers in HTML5 has to be stored in an external JavaScript file. However, for them to work properly, you also have to include some code in your HTML document:
<!DOCTYPE html>
<html>
<body>
<p>Count numbers: <output id="res"></output></p>
<button onclick="startCounting()">Start Counting</button>
<button onclick="stopWorker()">Stop Counting</button>
<p><strong>Note:</strong>All Internet Explorer versions before 9, do not support the Web Workers.</p>
<script>
var l;
function startCounting() {
console.log("Worker started");
if(typeof(Worker) !== "undefined") {
if(typeof(l) == "undefined") {
l = new Worker("demo_workers.js");
}
l.onmessage = function(event) {
document.getElementById("res").innerHTML = event.data;
};
} else {
document.getElementById("res").innerHTML = "Browser does not support Workers";
}
}
function stopWorker() {
console.log("Worker terminated");
l.terminate();
l = undefined;
}
</script>
</body>
</html>
We'll explain the most crucial parts of the code in the table below.
Code section | Meaning |
---|---|
var l; function startCounting() { |
Sets up a global variable |
if(typeof(l) == "undefined") { l = new Worker("demo_workers.js"); } |
Initializes a web worker |
l.onmessage = function(event) { document.getElementById("res").innerHTML = event.data; }; |
Allows listening to messages and updating the page accordingly |
} else { document.getElementById("res").innerHTML = "Browser does not support Workers"; } } |
Informs the user if their browser doesn't support web workers |
function stopWorker() { console.log("Worker terminated"); l.terminate(); l = undefined; } |
Terminates the web worker |
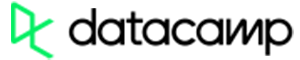
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
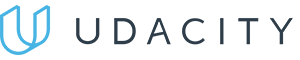
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
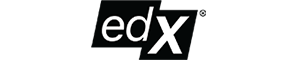
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Web Workers: Useful Tips
- By using setInterval() and setTimeout() JavaScript functions, you can make web workers perform periodic tasks.
- You cannot access DOM elements using web workers, so don't include any in their scripts.
- Not sure when to use web workers? The most common tasks include spell checking, syntax highlighting, prefetching and caching data.
Browser support
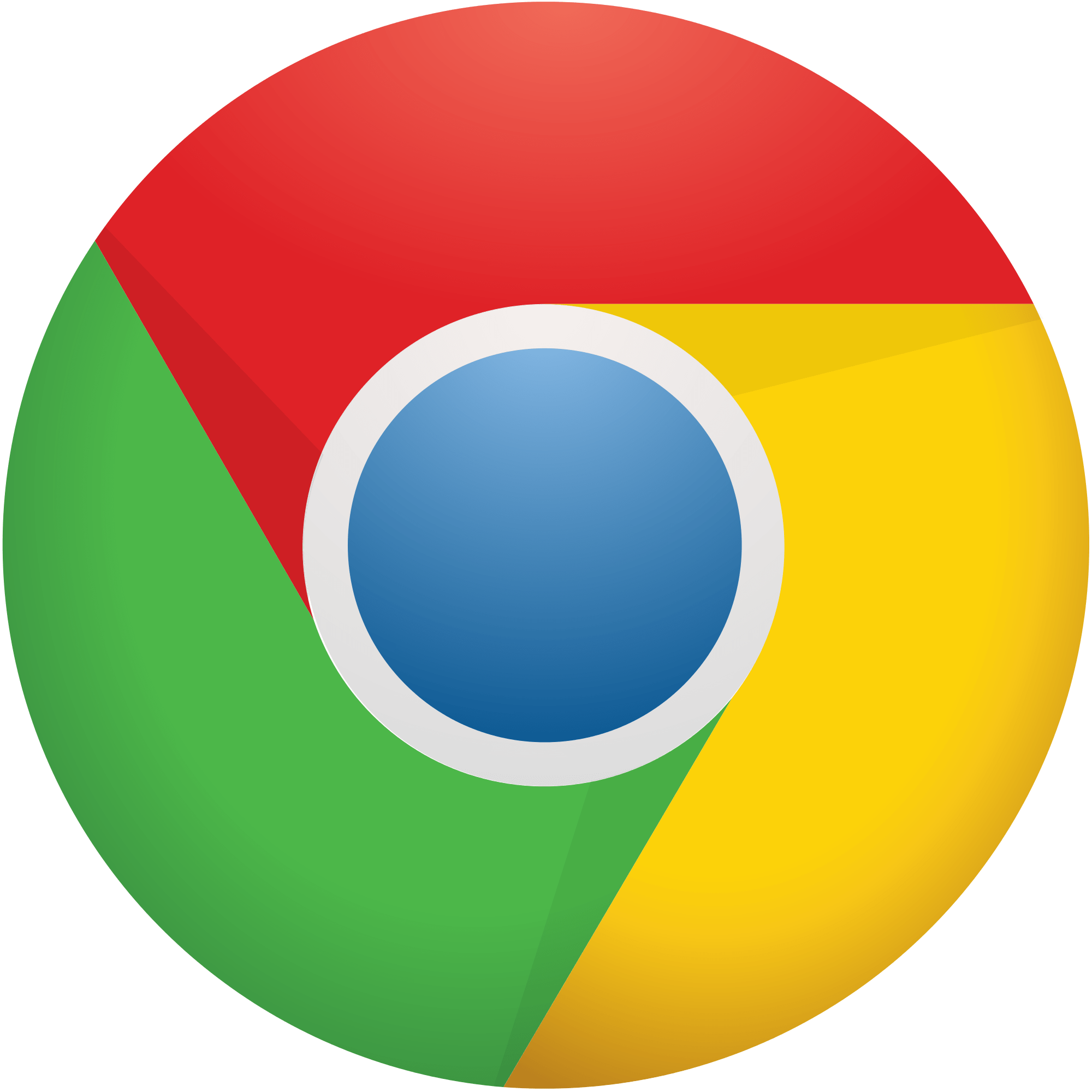
Chrome
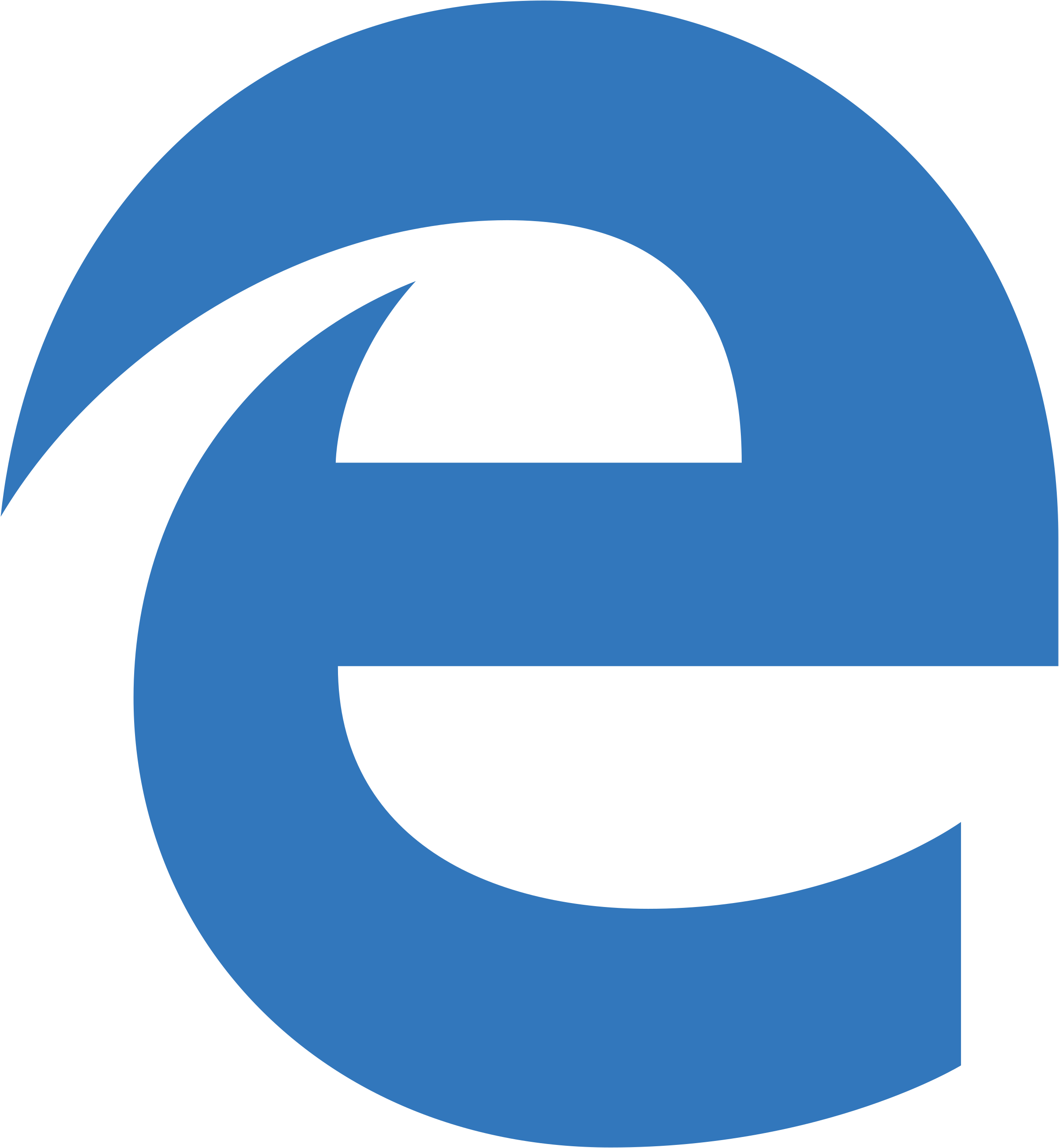
Edge
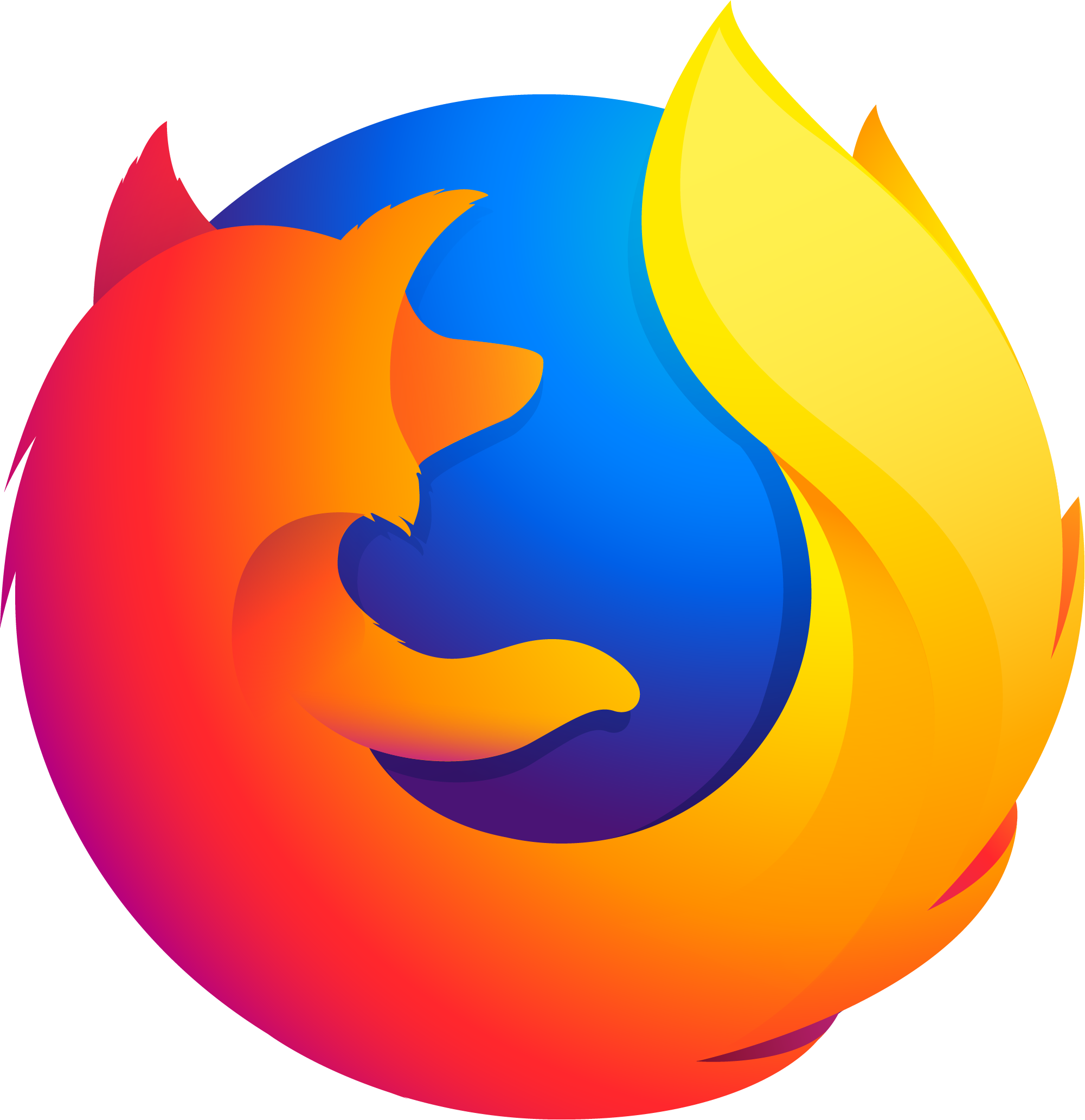
Firefox
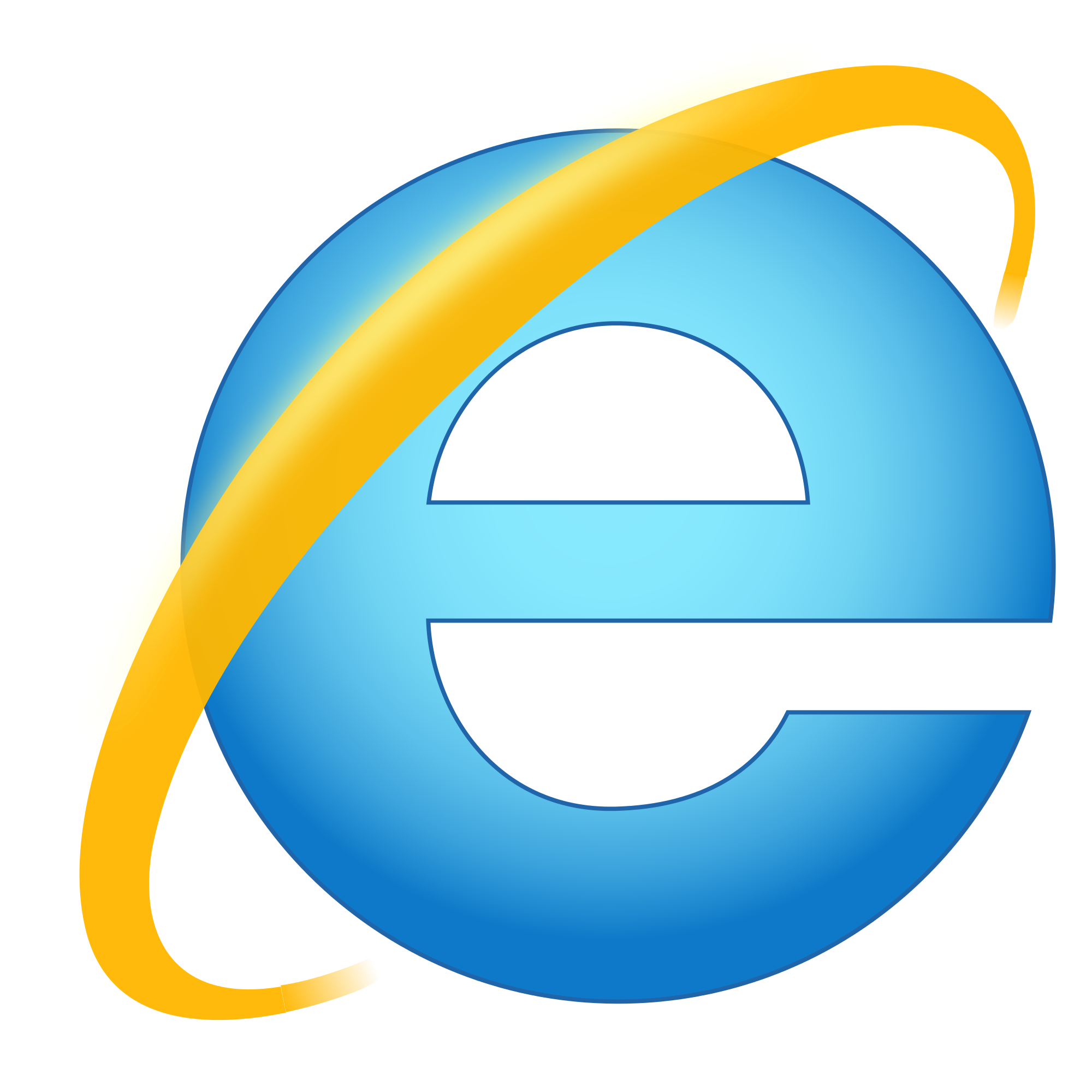
IE
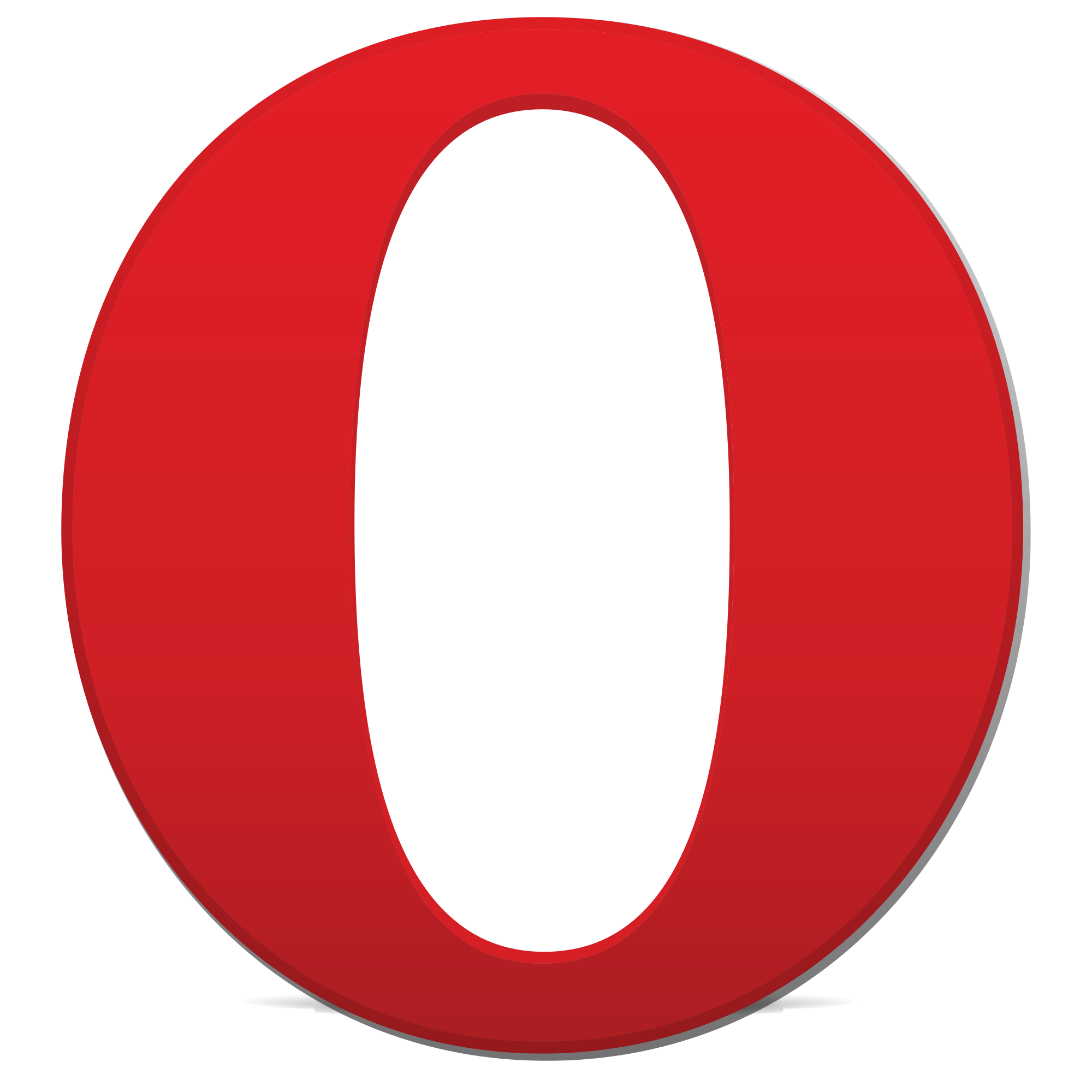
Opera
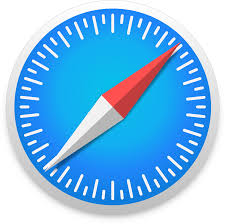
Safari
Mobile browser support
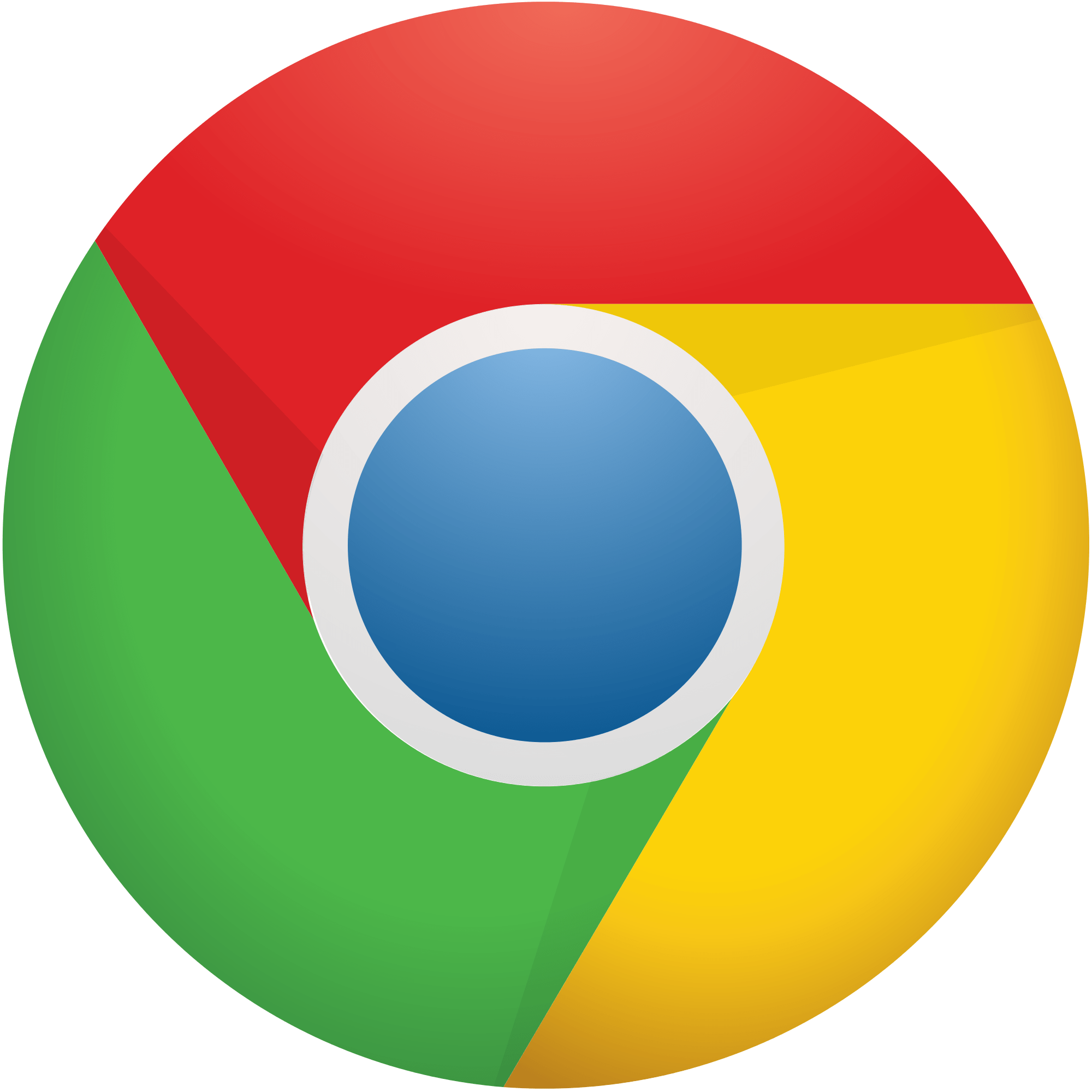
Chrome
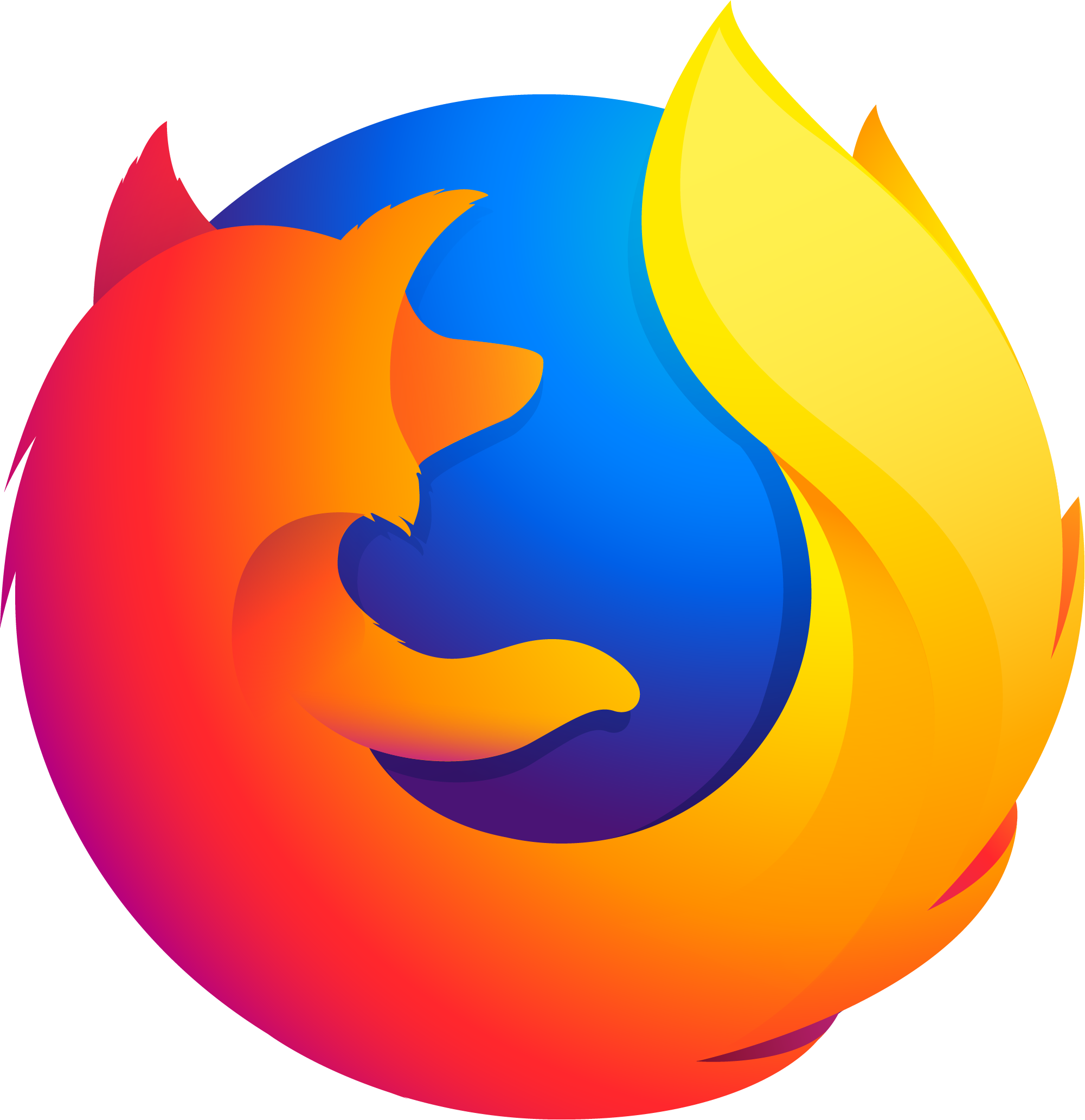
Firefox
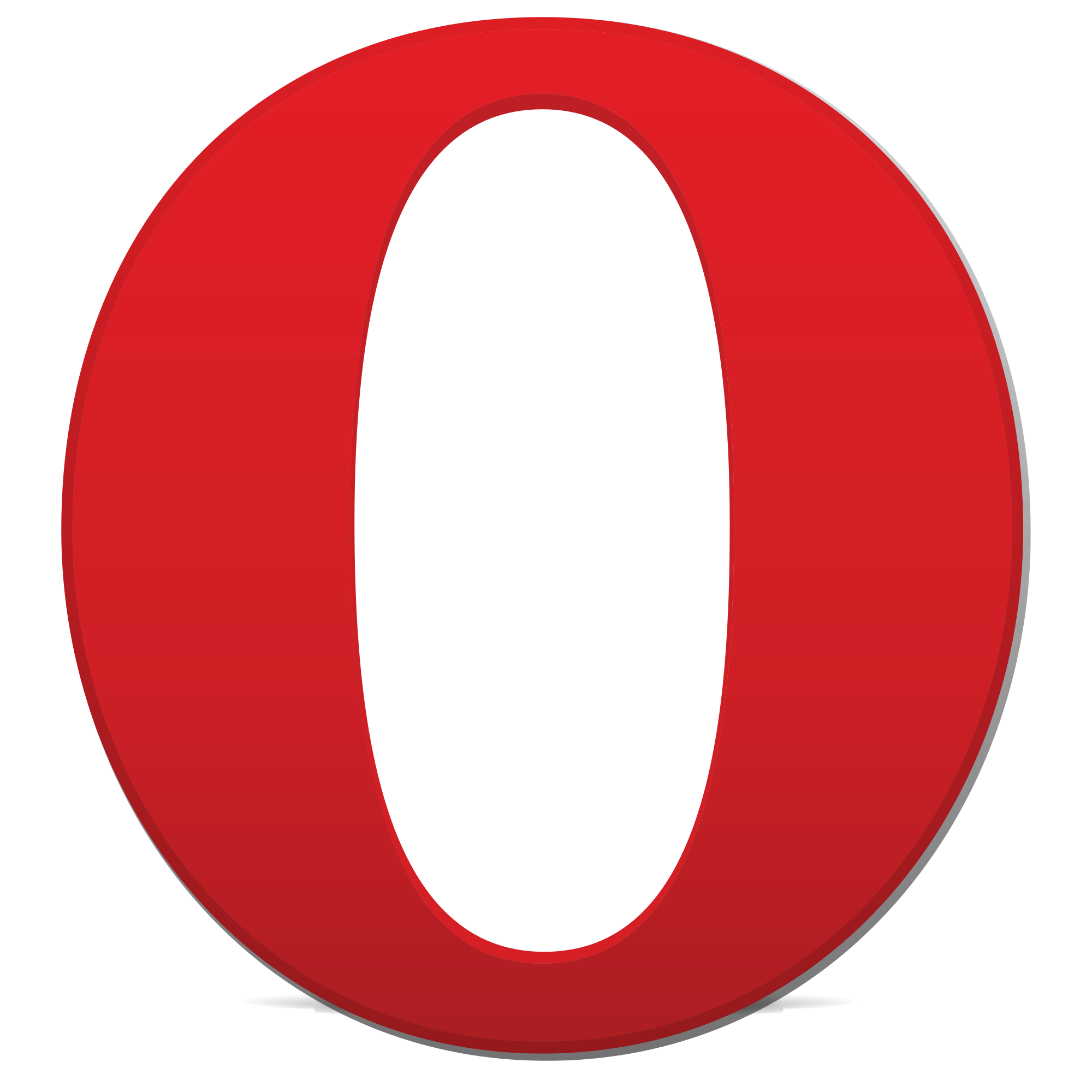
Opera
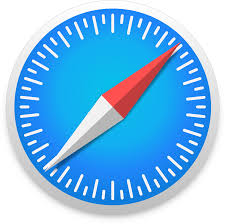