TL;DR – HTML form elements let the developer collect user input of various types.
Contents
Using HTML <form> Tags
To create an HTML form, you need to use <form> tags:
<form>
<br>First name:<br>
<input type="text" name="first name" placeholder="firstname">
<br>Last name:<br>
<input type="text" name="lastname" placeholder="lastname">
</form>
However, the tags don't create the form itself. The <form>
element acts as a container for the form elements that create and define the functionalities you expect from your HTML form. Input types and other information can also be defined using attributes.
New Form Elements in HTML5
The <datalist> element defines a list of options for the <input>
element. The user will see an HTML drop down list and will be able to select an option:
<input list="names">
<datalist id="names">
<option value="Tom">
<option value="Sam">
<option value="Jack">
<option value="Annie">
<option value="Rich">
</datalist>
</input>
Note: the id attribute of the <datalist> element must match the list attribute of the <input> element.
The <output> element defines a result of a calculation. If you include two or more numeric inputs, this element will be able to generate the result:
<form oninput="result.value=parseInt(x.value)+parseInt(y.value)">
0<input type="range" id="x" value="75">100
+<input type="number" id="y" value="72">
=<output name="result" for="x y"></output>
</form>
The <keygen> element generated a private and public key pair to be used while submitting a form. However, this element is no longer supported in HTML5:
<form action="login" method="POST">
Username: <input type="text" name="user_name">
Password: <input type="password" name="user_password">
Keygen Strength: <keygen name="login_form">
<input type="submit" value="Login">
</form>
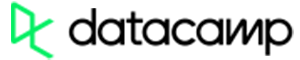
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
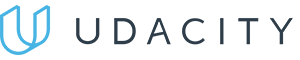
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
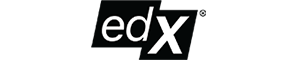
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Common Native Form Elements
The <input> element is arguably the most important of all the form elements. It defines a place for the user input, which can come it various formats specified by the type
attribute. In the example below, you can see type="text"
, so the user will be shown a box in which they have to type using their keyboard:
Email: <input type="text" name="email"><br>
Name: <input type="text" name="name"><br>
<input type="submit" value="Submit">
See all the available HTML5 input types in the table below:
button | checkbox | color | date | datetime-local | |
file | hidden | image | month | number | password |
radio | range | reset | search | submit | tel |
text | time | url | week |
The <textarea>
element comes in handy when we need textual data but a single line that a text field offers is not enough. It provides a multiline area for the user input:
<textarea rows="2" cols="50">
I am writing multiline text here. Some more text. Text. Text. Text. Text.
</textarea>
The <label> tag sets a name for the <input>
element. It gives the user a clear indication on what information they have to type in. It also improves user experience by increasing the clickable area:
<form>
<input type="radio" name="day" value="monday" checked>Monday<br>
<input type="radio" name="day" value="tuesday">Tuesday<br>
<input type="radio" name="day" value="wednesday">Wednesday
</form>
The <fieldset> element groups related elements which are then displayed in one separated box. It is usually used for elements that make no sense being used alone, e.g., radio buttons:
<form>
<fieldset>
<legend>Student:</legend>
Name: <input type="text"><br>
Surname: <input type="text"><br>
Class: <input type="text"><br>
Favourite food: <input type="text">
</fieldset>
</form>
Tip: if you want your user to be able to select multiple options in your HTML form, a group of checkboxes can be a great option.
The <legend> element creates a title for the <fieldset>
element:
<form>
<fieldset>
<legend>Student:</legend>
Name: <input type="text"><br>
Surname: <input type="text"><br>
Favourite class: <input type="text"><br>
</fieldset>
</form>
The <select> element generates an HTML drop down list:
<select name="housepets">
<option value="cat">Cat</option>
<option value="dog">Dog</option>
<option value="llama">Llama</option>
<option value="rabbit">Rabbit</option>
<option value="animal">Animal</option>
</select>
You must include at least one option for the HTML drop down list to work properly. For that, you will have to use the <option> tags. One pair of tags define a single option, but you can add as many as you need:
<p>Which pet would you like to keep at home?</p>
<select>
<option value="cat">Cat</option>
<option value="wolf">Wolf</option>
<option value="llama">Llama</option>
</select>
You can also group related options in an HTML drop down list using <optgroup> tags:
<select>
<optgroup label="Sports">
<option value="baseball">Baseball</option>
<option value="basketball">Basketball</option>
</optgroup>
<optgroup label="Shopping">
<option value="food">Food</option>
<option value="gift">Gift</option>
</optgroup>
</select>
HTML Form Elements: Useful Tips
- Don't forget to include the submit button at the end of your form so the user can send the data to the server. You can do that using the <input> element with the
type
attribute and its value defined assubmit
. - Defining the name for a certain form control using the
name
attribute will help the server identify the inputted value.