TL;DR – HTML5 Geolocation API lets the user share their location data with a web application by giving a special permission.
Contents
HTML Geolocation API Explained
Introduced in HTML5, the Geolocation API allows you to receive information on the user's current geographical location. It can help you choose the relevant data to provide (e.g., weather or traffic information):
<!DOCTYPE html>
<html>
<head>
<script>
const demo = document.getElementById('demo');
function error(err) {
demo.innerHTML = `Failed to locate. Error: ${err.message}`;
}
function success(pos) {
demo.innerHTML = 'Located.';
alert(`${pos.coords.latitude}, ${pos.coords.longitude}`);
}
function getGeolocation() {
if (navigator.geolocation) {
demo.innerHTML = 'Locating…';
navigator.geolocation.getCurrentPosition(success, error);
} else {
demo.innerHTML = 'Geolocation is not supported by this browser.';
}
}
</script>
</head>
<body>
<button onclick="getGeolocation()">
Get my coordinates!
</button>
<div id="demo"></div>
</body>
</html>
The browser explicitly asks the user to give their permission, usually in the form of a pop-up alert or a dialog box. If they wish to keep their privacy, they can easily disagree with collecting such information.
Depending on the program, the user's location can be defined in coordinates, displayed on a map or a navigation route.
How Does Geolocation Work
Identifying the geographical location of the user relies on multiple methods. Computer browsers use the IP address, and mobile devices use GPS and cell triangulation methods. Both also use WiFi when possible.
To receive information about the current location of the user, you have to use the navigator.geolocation.getCurrentPosition()
method:
function success(pos) {
alert(`latitude: ${pos.coords.latitude}
\n longitude: ${pos.coords.longitude}
\n accuracy: ${pos.coords.accuracy}`);
}
function getGeolocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(success);
}
}
getGeolocation();
If the user agrees to share the data, you will receive a position object that contains a timestamp and an object called coords
. See the values it defines:
Property | Meaning | Units |
---|---|---|
Latitude and longitude | Geographical coordinates | Degrees |
Accuracy | Accuracy of the latitude and longitude data | Meters |
Altitude | Height in relation to sea level | Meters |
AltitudeAccuracy | Accuracy of the altitude data | Meters |
Heading | The direction of movement | Degrees |
Speed | The speed of movement | Meters per second |
Note: the first three values are returned every time, no matter the location identifying method. The other four can only be returned if available.
To keep receiving HTML geolocation data in regular intervals, use the watchPosition()
method. It returns the updated position of a user when it changes or when a more accurate location technique can be used:
function success(pos) {
alert(`latitude: ${pos.coords.latitude}
\n longitude: ${pos.coords.longitude}
\n accuracy: ${pos.coords.accuracy}`);
}
function watchPosition() {
if (navigator.geolocation) {
navigator.geolocation.watchPosition(success);
}
}
watchPosition();
Note: the watchPosition() method provides a unique ID that can be used to identify the position watcher.
To stop the watchPosition()
method, use clearWatch()
:
<script>
var watchId = -1;
function error(err) {
console.warn(err.message);
}
function success(pos) {
alert(`latitude: ${pos.coords.latitude}
\n longitude: ${pos.coords.longitude}
\n accuracy: ${pos.coords.accuracy}`);
}
function watchPosition() {
if (navigator.geolocation) {
watchId = navigator.geolocation.watchPosition(success);
}
}
function stopWatching() {
if (navigator.geolocation) {
navigator.geolocation.clearWatch(watchId);
}
}
</script>
<body>
<button onclick="watchPosition()">
Watch My Position!
</button>
<button onclick="stopWatching()">
Stop Watching
</button>
</body>
Using the Google Geolocation API
You can display the coordinates returned by the HTML5 Geolocation API methods on a map. To do that, you need a map service (e.g., Google Maps). The following example uses the Google Geolocation API to display the current user location.
First, you must define an HTML element as the map canvas and invoke the Google Geolocation API. Then, use the HTML5 geolocation methods to get the user location. It will be sent to a function that uses Google Geolocation API and shown on the map:
<body>
<div id="canvas" style="width: 100%; height: 400px;"></div>
<script>
function getGeolocation() {
navigator.geolocation.getCurrentPosition(drawMap);
}
function drawMap(geoPos) {
geolocate = new google.maps.LatLng(geoPos.coords.latitude, geoPos.coords.longitude);
let mapProp = {
center: geolocate,
zoom:5,
};
let map=new google.maps.Map(document.getElementById('canvas'),mapProp);
let infowindow = new google.maps.InfoWindow({
map: map,
position: geolocate,
content:
`Location from HTML5 Geolocation:
<br>Latitude: ${geoPos.coords.latitude}
<br>Longitude: ${geoPos.coords.longitude}`
});
}
getGeolocation();
</script>
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY"></script>
If this seems a bit complex, we can analyze the example above in more detail:
<div id="canvas" style="width:100%;height:400px;"></div>
sets an HTML element to be used as a map canvas.<script src="https://maps.googleapis.com/maps/api/js"></script>
invokes the Google Geolocation API.getGeolocation()
is the first function to be executed.navigator.geolocation.getCurrentPosition(drawMap);
gets the user location and sends it to thedrawMap()
function.drawMap(geoPos)
uses Google Maps API to show the user location on the map.geolocate = new google.maps.LatLng(geoPos.coords.latitude, geoPos.coords.longitude);
creates a Google MapsLatLng
object, based on the HTML geolocation coordinates.var map=new google.maps.Map(document.getElementById("canvas"),mapProp);
creates a Google map inside the given HTML element.var infowindow = new google.maps.InfoWindow({...})
creates a Google Mapsinfowindow
that shows the user latitude and longitude.
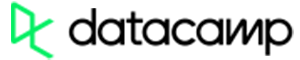
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
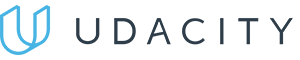
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
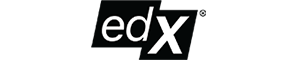
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Error Handling
If some method fails on getting the user's position, they will return error codes:
Error code | Meaning |
---|---|
error.PERMISSION_DENIED | The user rejected the request. |
error.POSITION_UNAVAILABLE | The HTML5 geolocation information is unavailable. |
error.TIMEOUT | The request for the location information has timed out. |
error.UNKNOWN_ERROR | The occurred error cannot be specified. |
In most cases, the errors can be easily handled by using an error callback function:
function error(err) {
console.error(`ERROR(${error.code}): ${err.message}`);
}
function success(pos) {
alert(`latitude: ${pos.coords.latitude}
\n longitude: ${pos.coords.longitude}
\n accuracy: ${pos.coords.accuracy}`);
}
function getGeolocation() {
navigator.geolocation.getCurrentPosition(success, error);
}
HTML5 Geolocation: Useful Tips
- Keep in mind that lower accuracy is not always a bad thing – it all depends on the goal. It might ruin route navigation, but still be great for looking up local businesses or finding out weather data.
- While HTML5 Geolocation API is not supported in older browsers, you can change that by using wrapper libraries.
Browser support
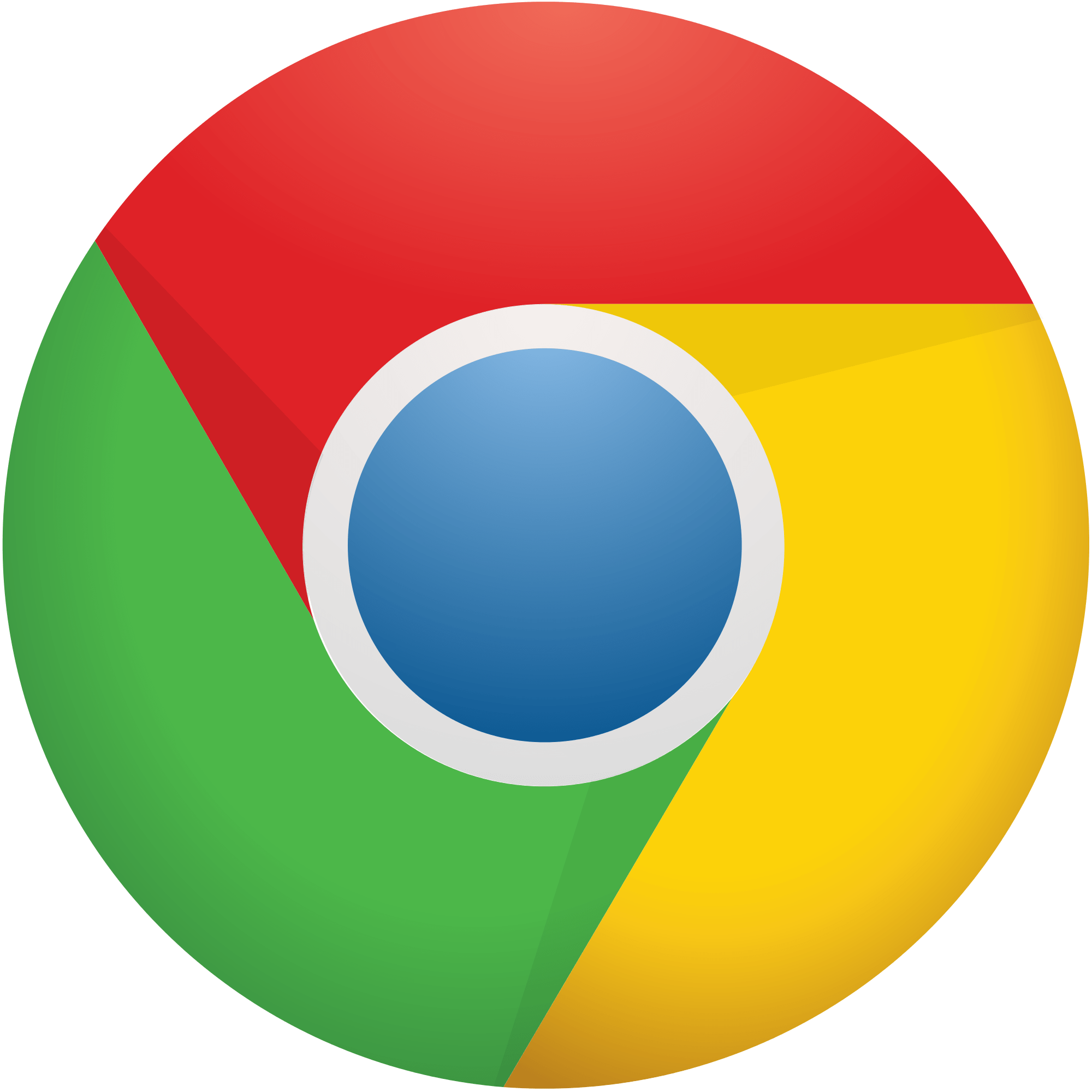
Chrome
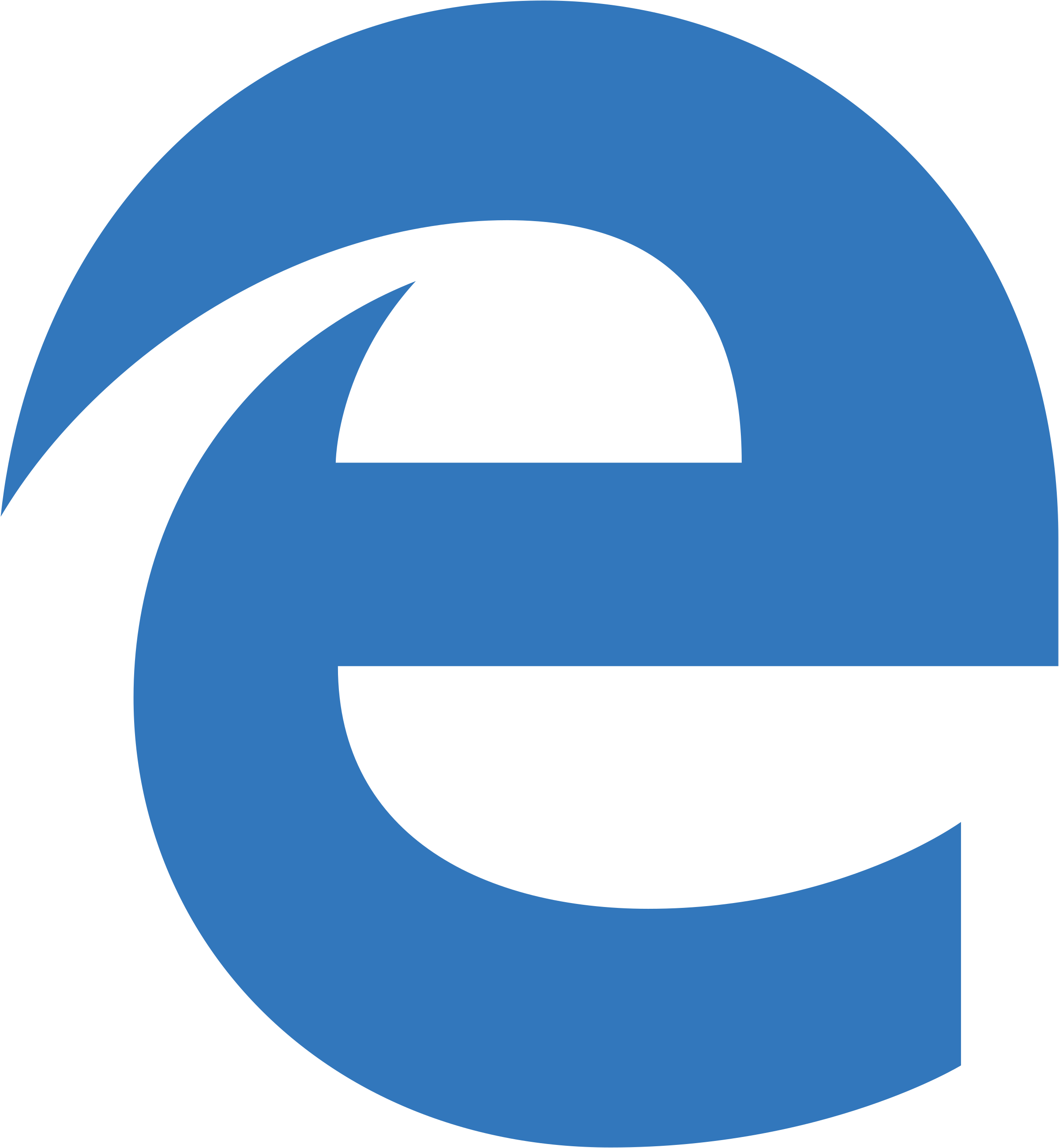
Edge
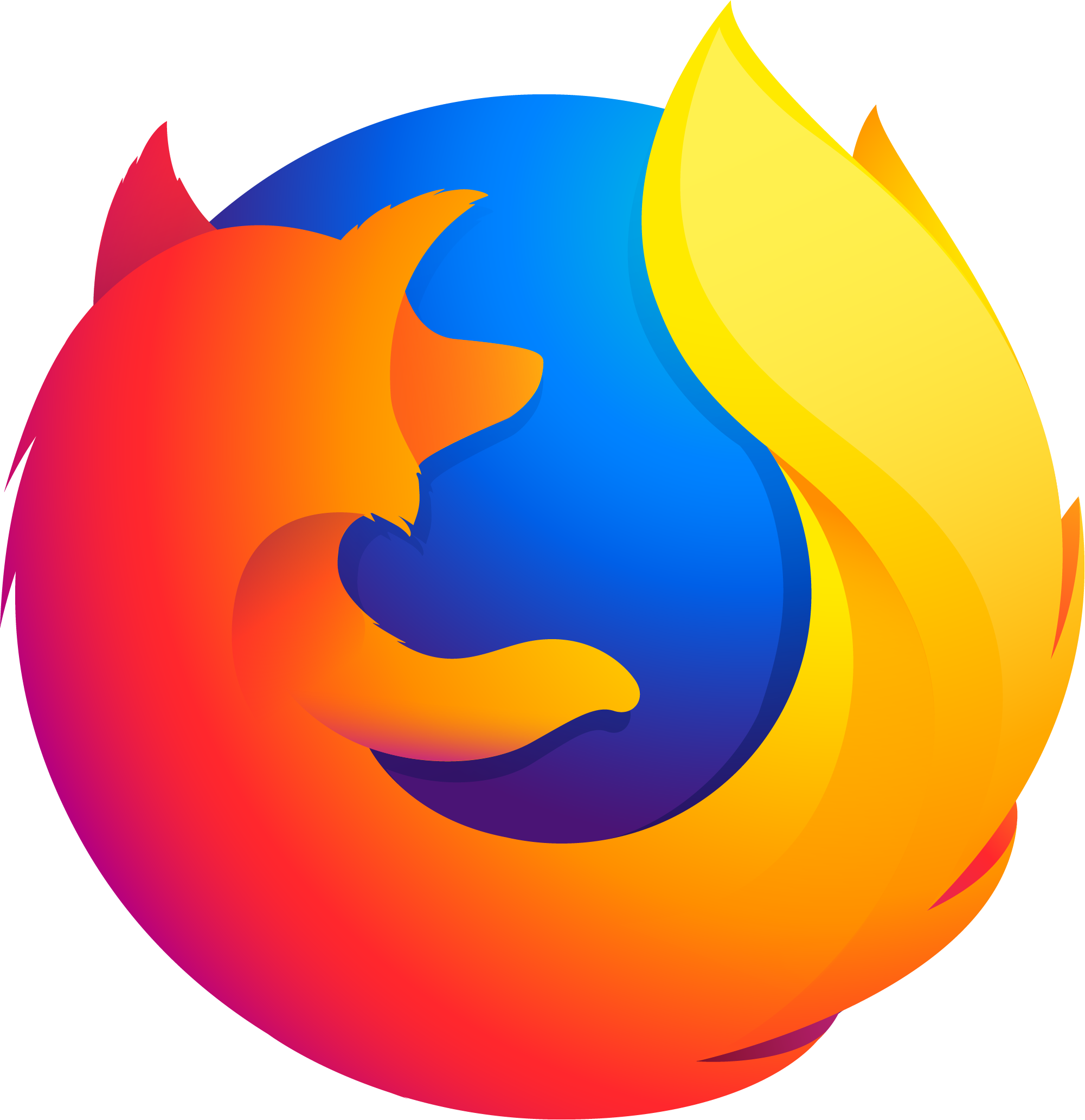
Firefox
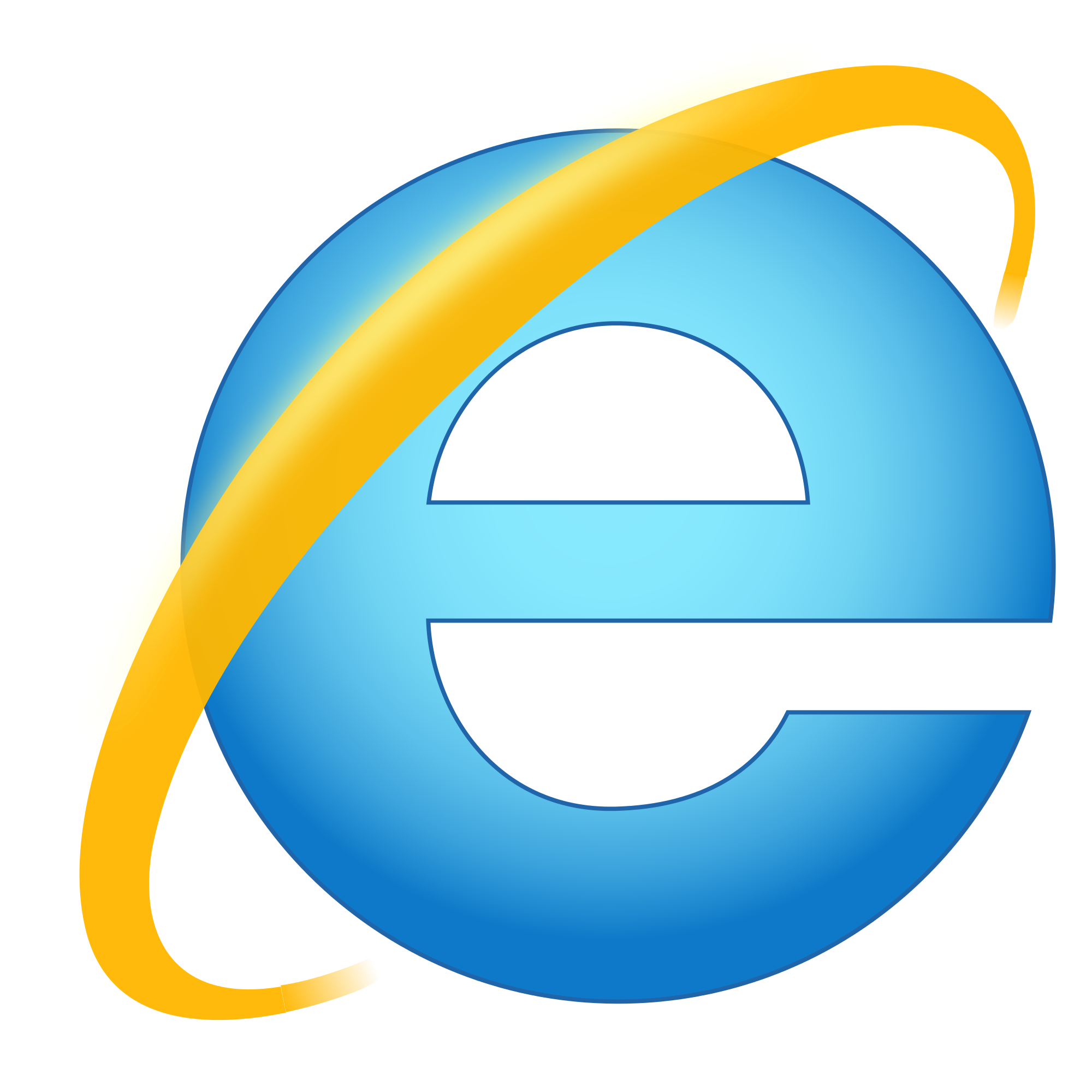
IE
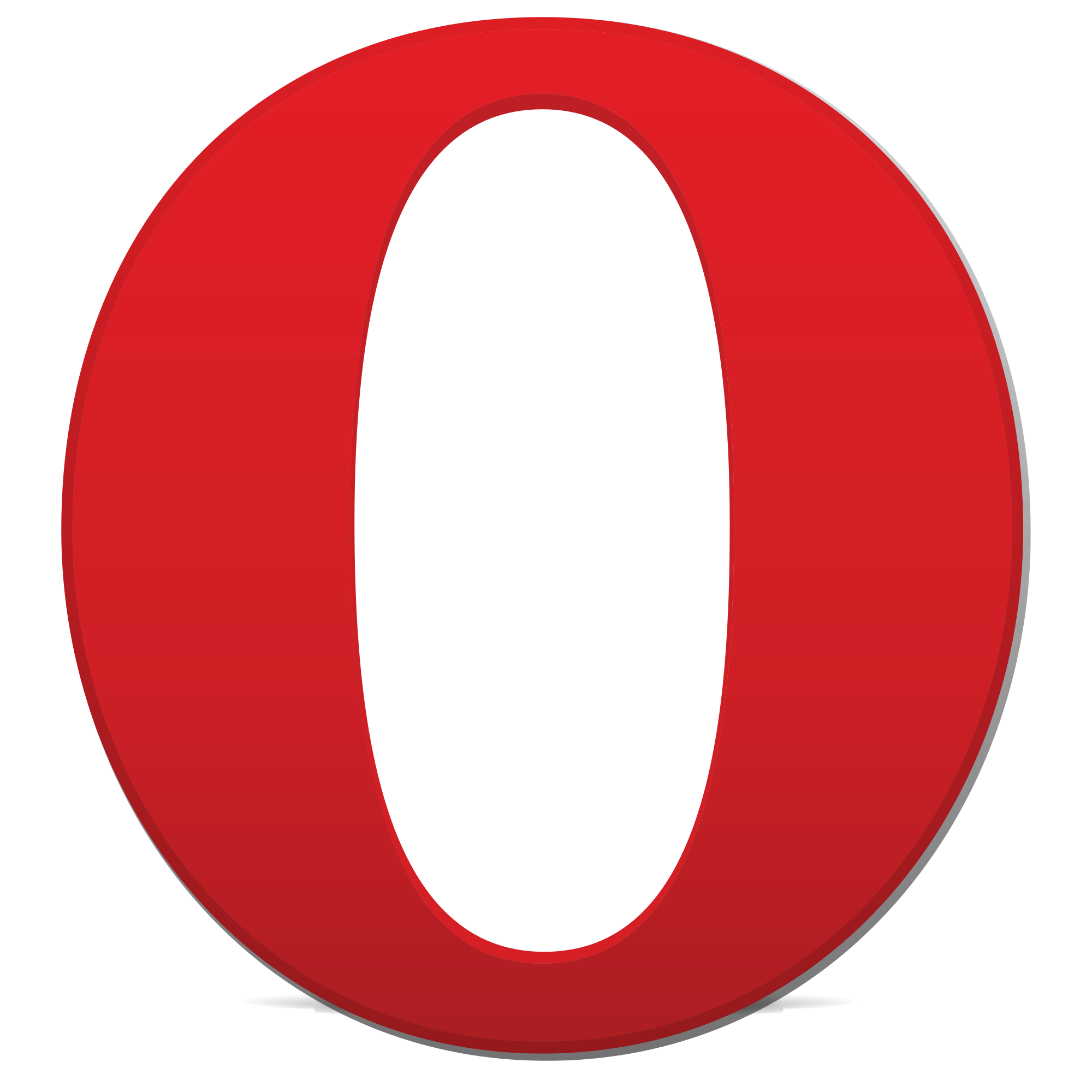
Opera
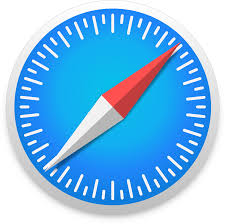
Safari
Mobile browser support
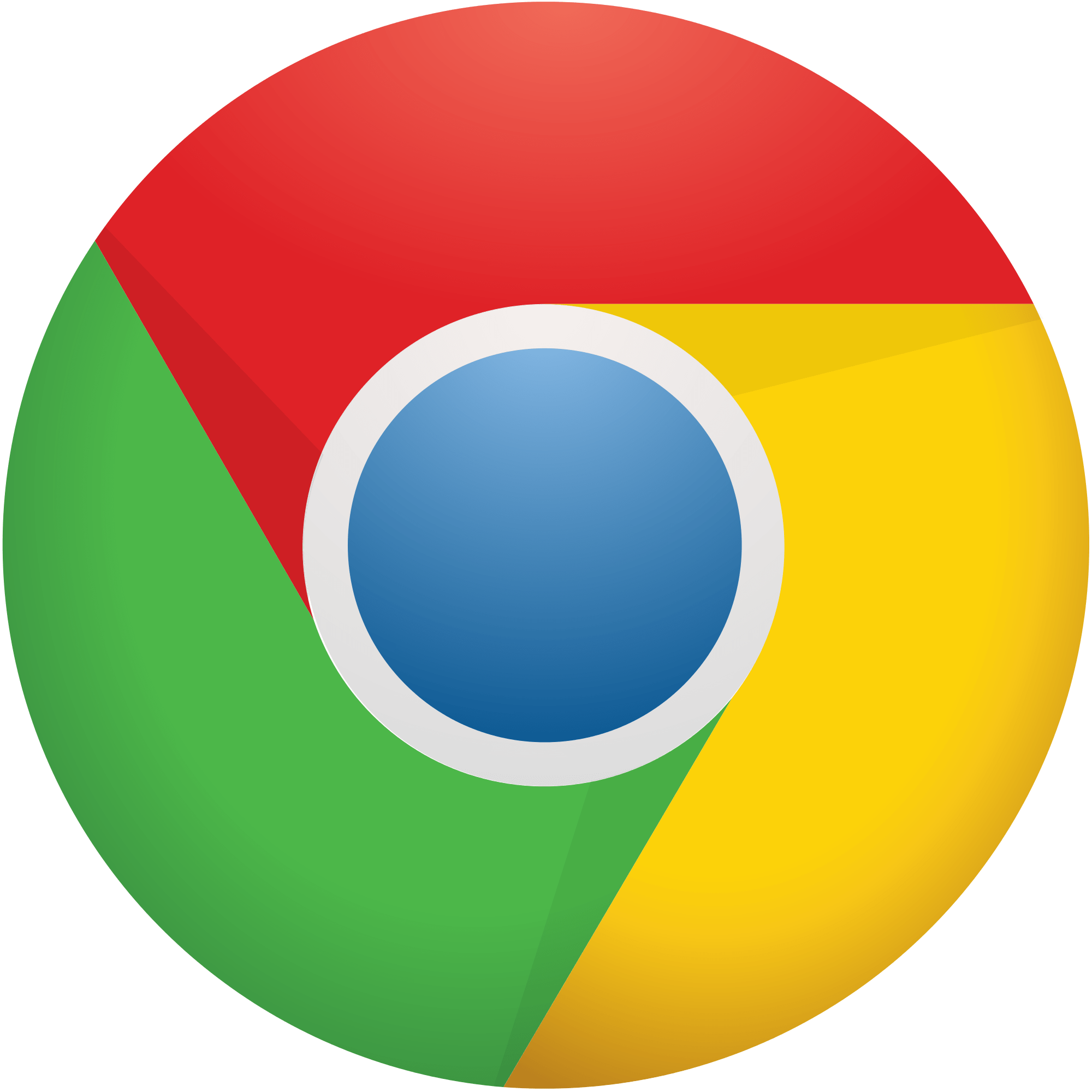
Chrome
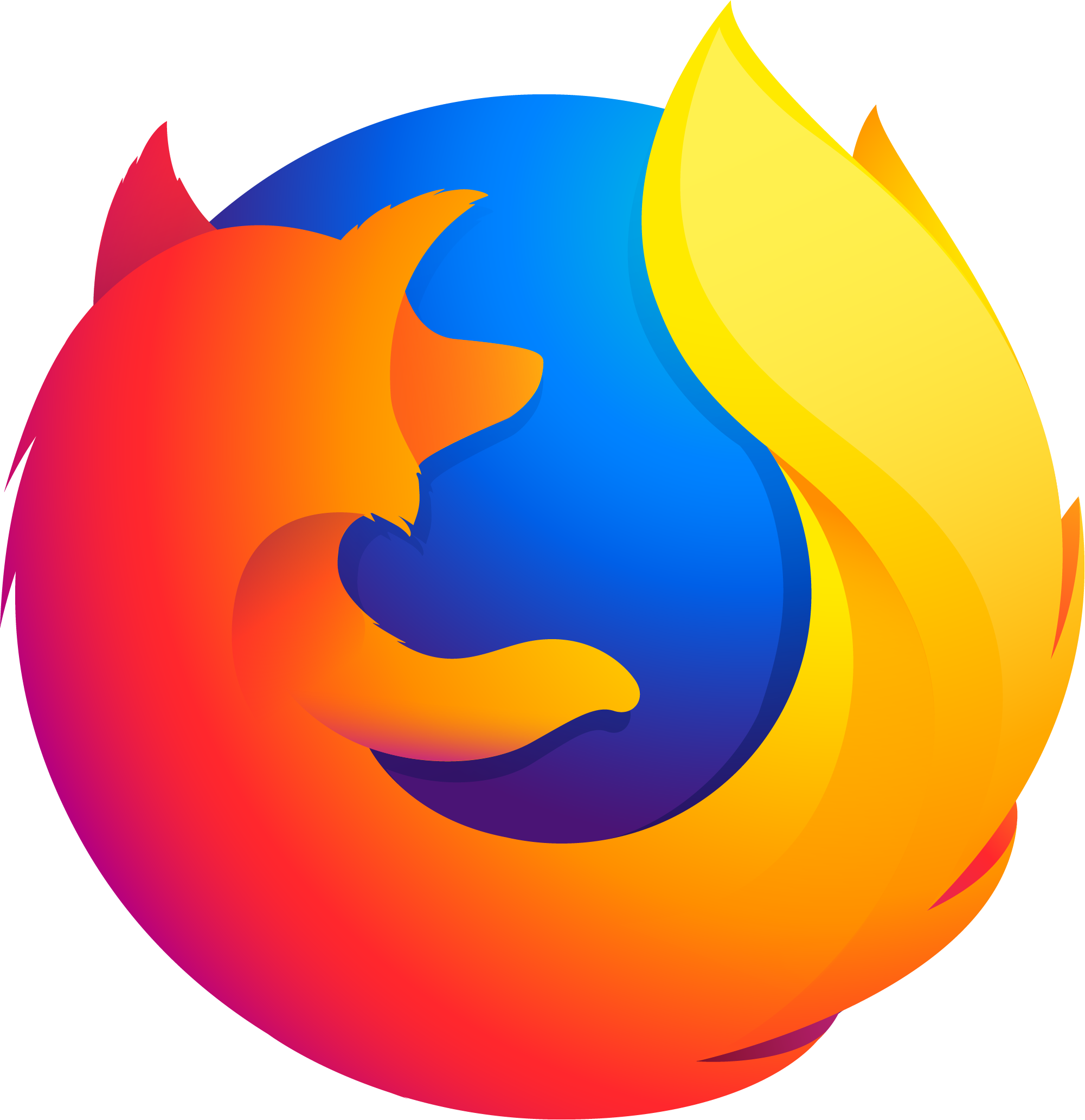
Firefox
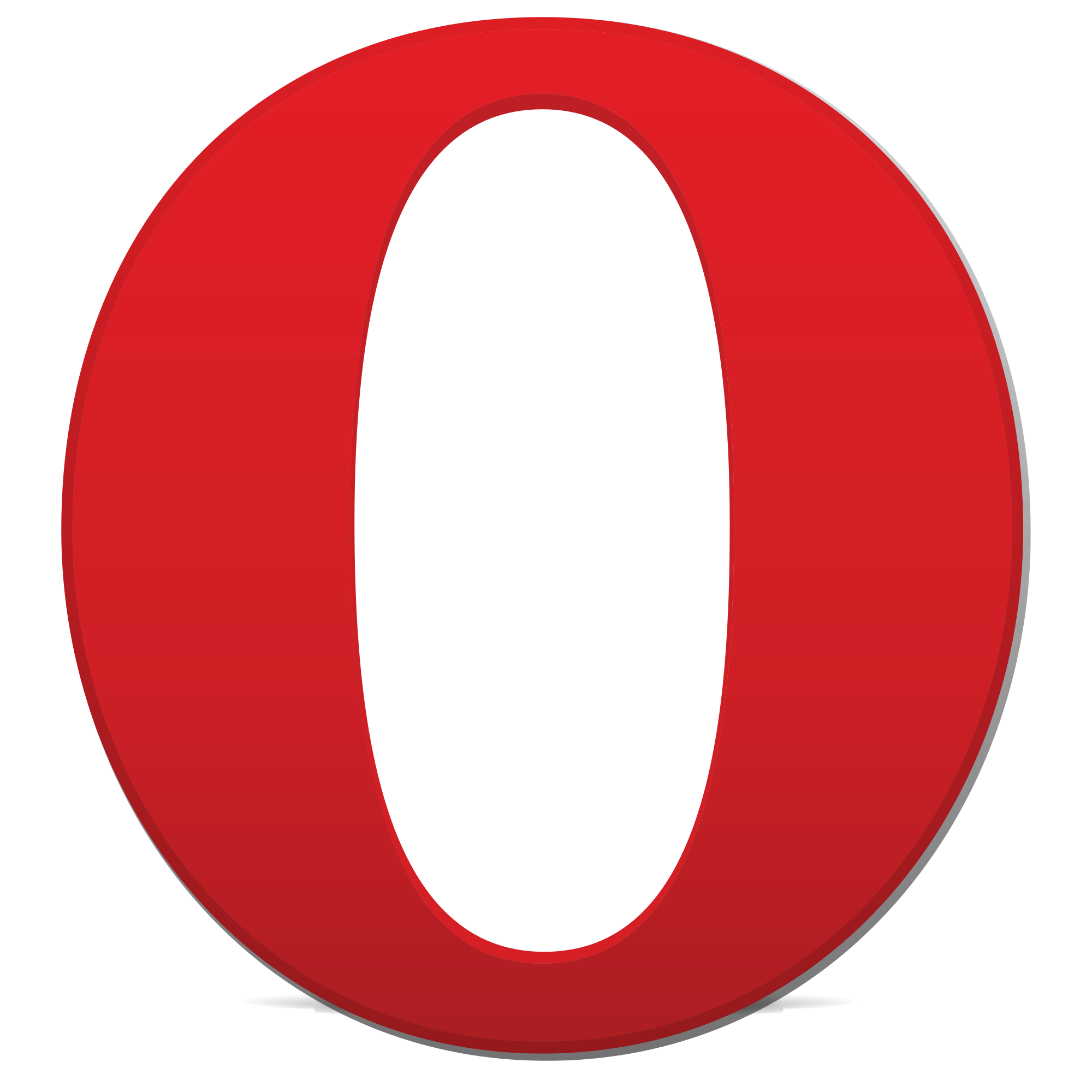
Opera
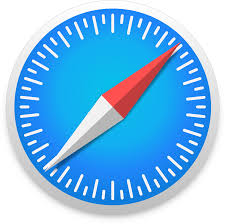