TL;DR – HTML5 Drag and Drop API allows you to grab an HTML object with your cursor and drag it to another place.
Contents
What Can You Drag and Drop in HTML5?
HTML5 allows you to make any element draggable, allowing you to pick it up with your cursor and drag it to a different location which is defined as a droppable element. In the example below, try to drag an image to a square:
function allowDropThis(i) {
i.preventDefault();
}
function dragThis(i) {
i.dataTransfer.setData("doggo", i.target.id);
}
function dropThis(i) {
i.preventDefault();
var data = i.dataTransfer.getData("doggo");
i.target.appendChild(document.getElementById(data));
}
This functionality simplifies user experience and even allows creating simple online games (e.g., you can drag and drop virtual chess pieces). When you drag an element, its transparent replica moves along with your cursor until you drop it by releasing the mouse button.
Technically, Drag and Drop is a JavaScript API added to HTML5. Therefore, it is important to have at least basic scripting knowledge to be able to work with this functionality.
Events for the HTML5 Drag and Drop
In the table below, you will see all the events that are relevant to the Drag and Drop functionality:
Event | Explanation |
---|---|
drag | The cursor moves while dragging an element |
drop | The dragging process finishes |
dragstart | The cursor picks up the element and starts dragging |
dragend | The mouse button is released, dropping the element |
dragenter | The cursor enters the target element |
dragleave | The cursor leaves the target element |
dragover | The cursor moves over the target element |
Step by Step Explanation of Drag and Drop
HTML5 drag and drop coding seems quite complicated at first sight as it includes scripting in JS. However, let's study each part of the process to make it easier to understand.
Draggable Elements
First, you have to make sure that the element you wish to drag has an HTML5 draggable
attribute applied and its value set to true
:
<img draggable="true">
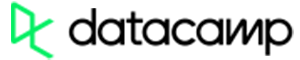
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
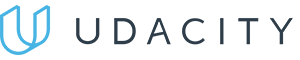
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
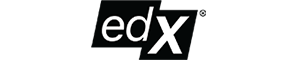
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Dragging
Then, define what should happen after you drag an object. You can do it by calling an event handler for a dragstart
event and defining a function to be executed.
The value and data type of the dragged data have to be set by the dataTransfer.setData()
method:
function dragThis(i) {
i.dataTransfer.setData("doggo", i.target.id);
}
Dropping
The HTML5 draggable data is specified by attaching an event handler to the dragover
event.
Elements and data cannot be dropped in other elements by default. This means you have to stop the element's default behavior using the event.preventDefault()
method:
event.preventDefault();
A drop
event occurs when the HTML5 draggable data is dropped. It is called by the ondrop
event handler:
function drop(i) {
i.preventDefault();
var data = i.dataTransfer.getData("doggo");
i.target.appendChild(document.getElementById(data));
}
The Final Code: an Example
Basically, you need your code to be able to perform four steps:
- Prevent the web browser's default action related with the data by calling
preventDefault()
- Get the data with the
dataTransfer.getData()
method - Affix the dragged element on the drop element
Let's see how it works in the example below:
<!DOCTYPE HTML>
<html>
<head>
<script>
function allowDropThis(i) {
i.preventDefault();
}
function dragThis(i) {
i.dataTransfer.setData("doggo", i.target.id);
}
function dropThis(i) {
i.preventDefault();
var data = i.dataTransfer.getData("doggo");
i.target.appendChild(document.getElementById(data));
}
</script>
</head>
<body>
<h2>Drag and Drop</h2>
<p>Drag Doggo between these the two elements.</p>
<div id="fig1" ondrop="dropThis(event)" ondragover="allowDropThis(event)">
<img src="https://cdn.bitdegree.org/learn/pom-laptop.png?raw=true" draggable="true" ondragstart="dragThis(event)" id="drag1" width="220" height="220">
</div>
<div id="fig2" ondrop="dropThis(event)" ondragover="allowDropThis(event)"></div>
</body>
</html>
HTML5 Drag and Drop: Useful Tips
- You can drag various types of elements, including but not limited to files, links, images and text.
- You may also drag multiple elements at once. However, it's recommended to have them all in the same format for consistency.
- Form elements that accept user input can be droppable areas by default.
Browser support
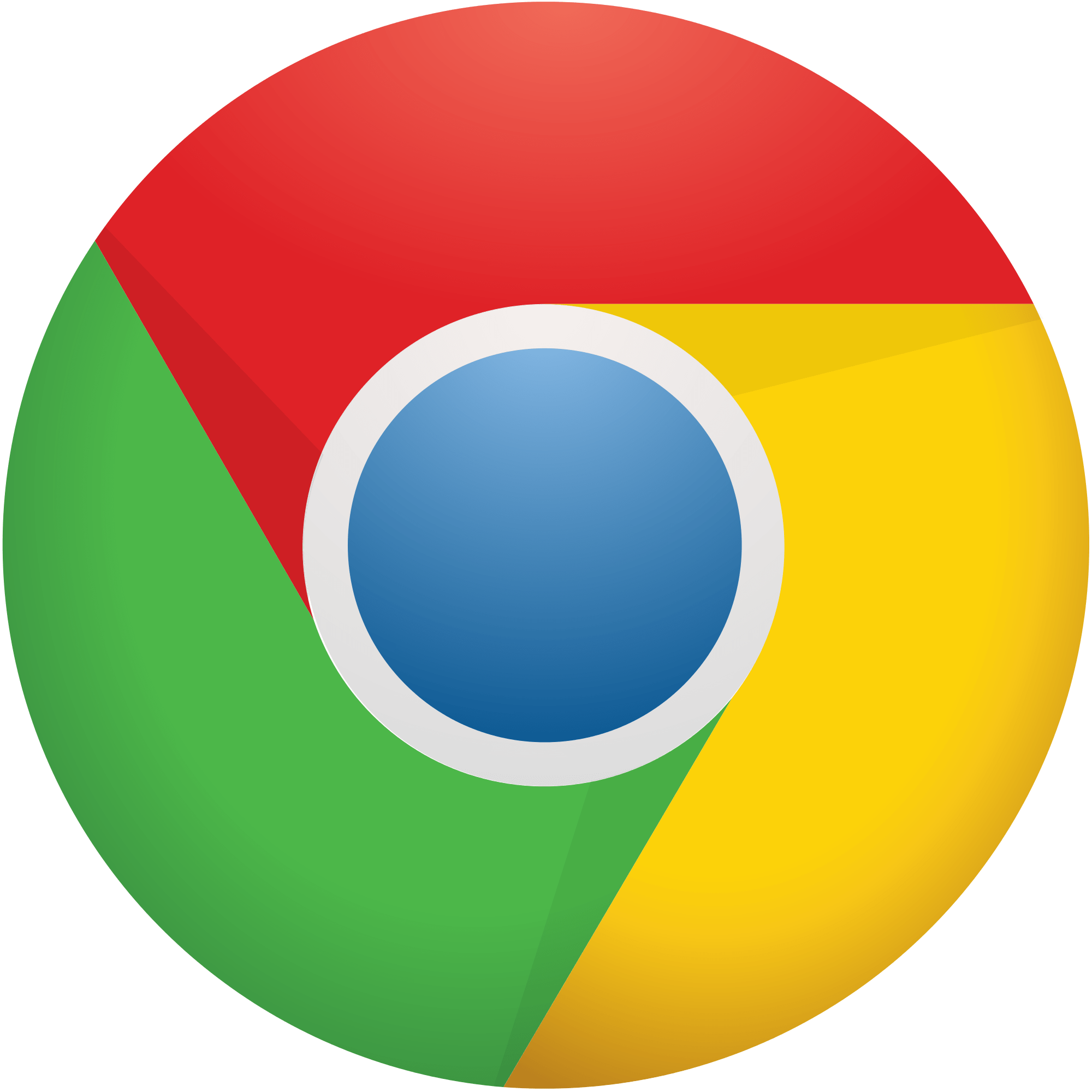
Chrome
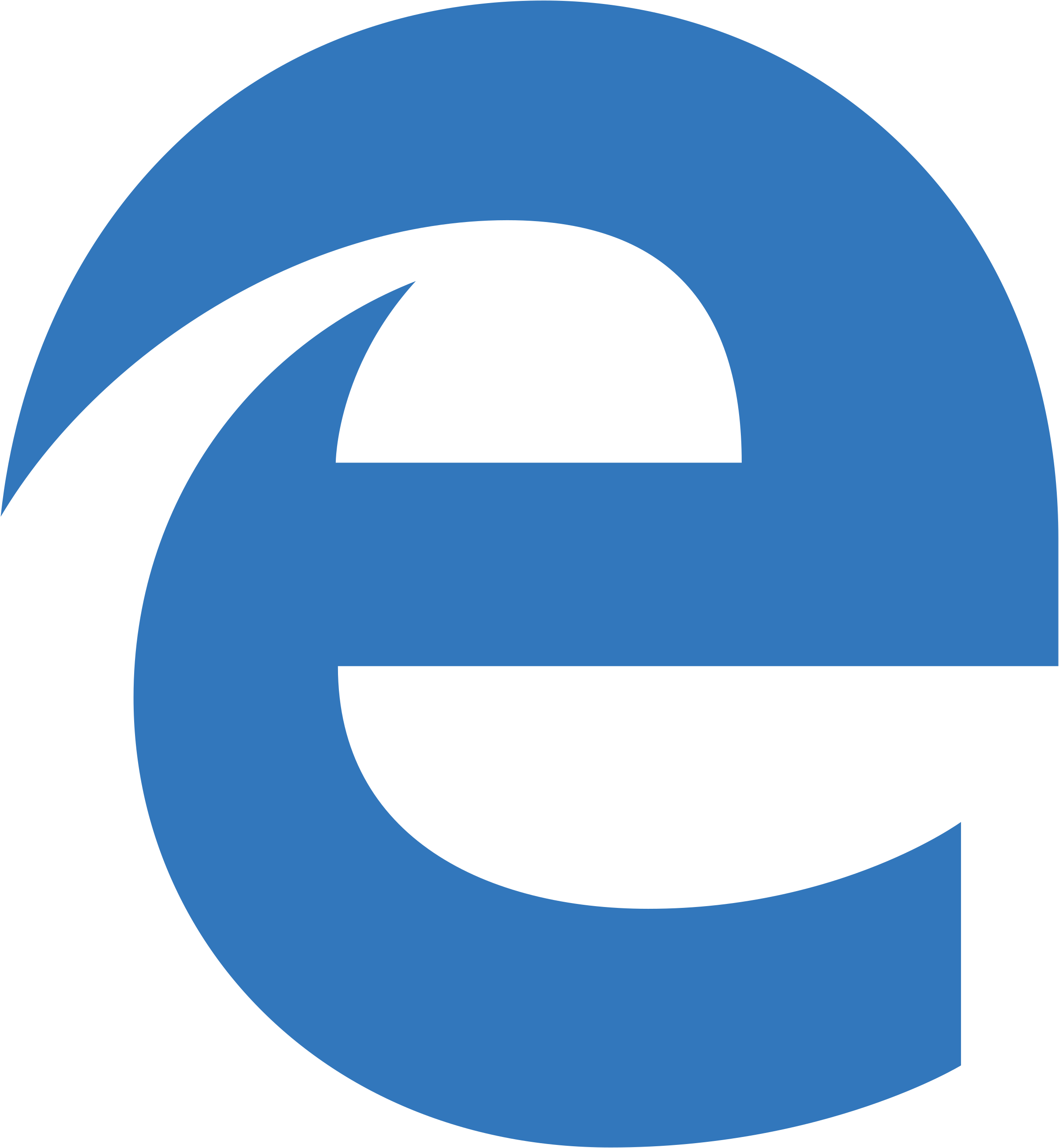
Edge
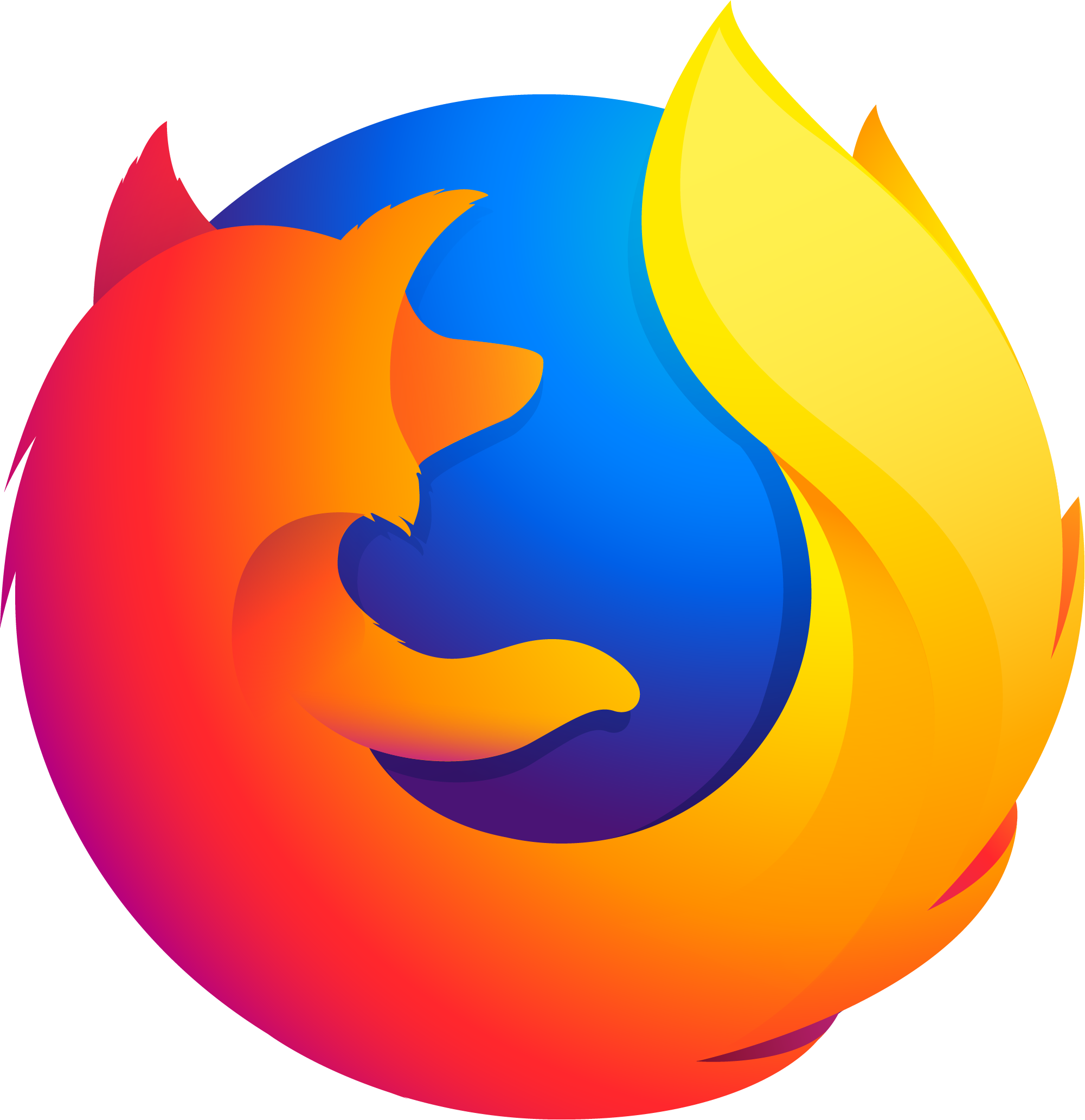
Firefox
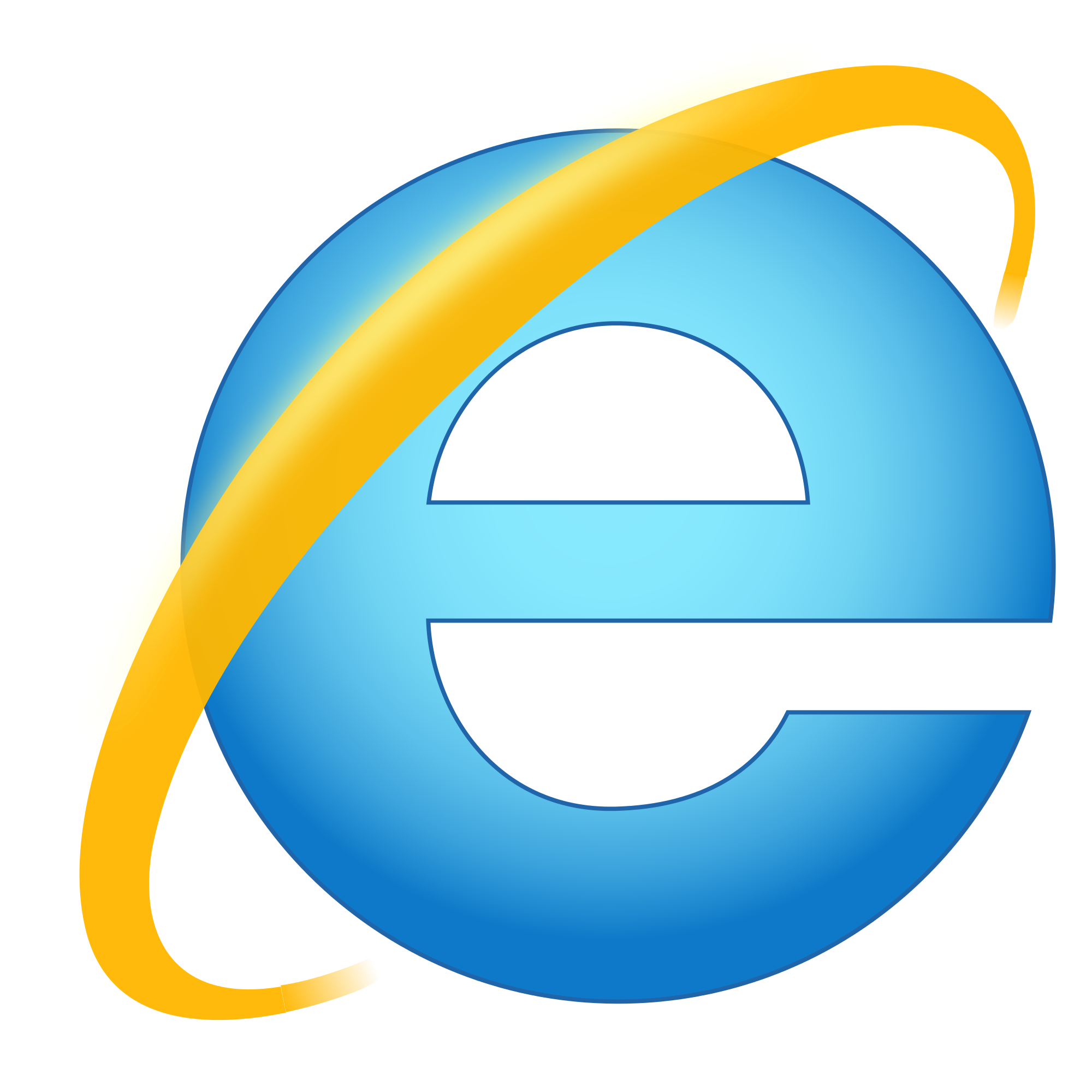
IE
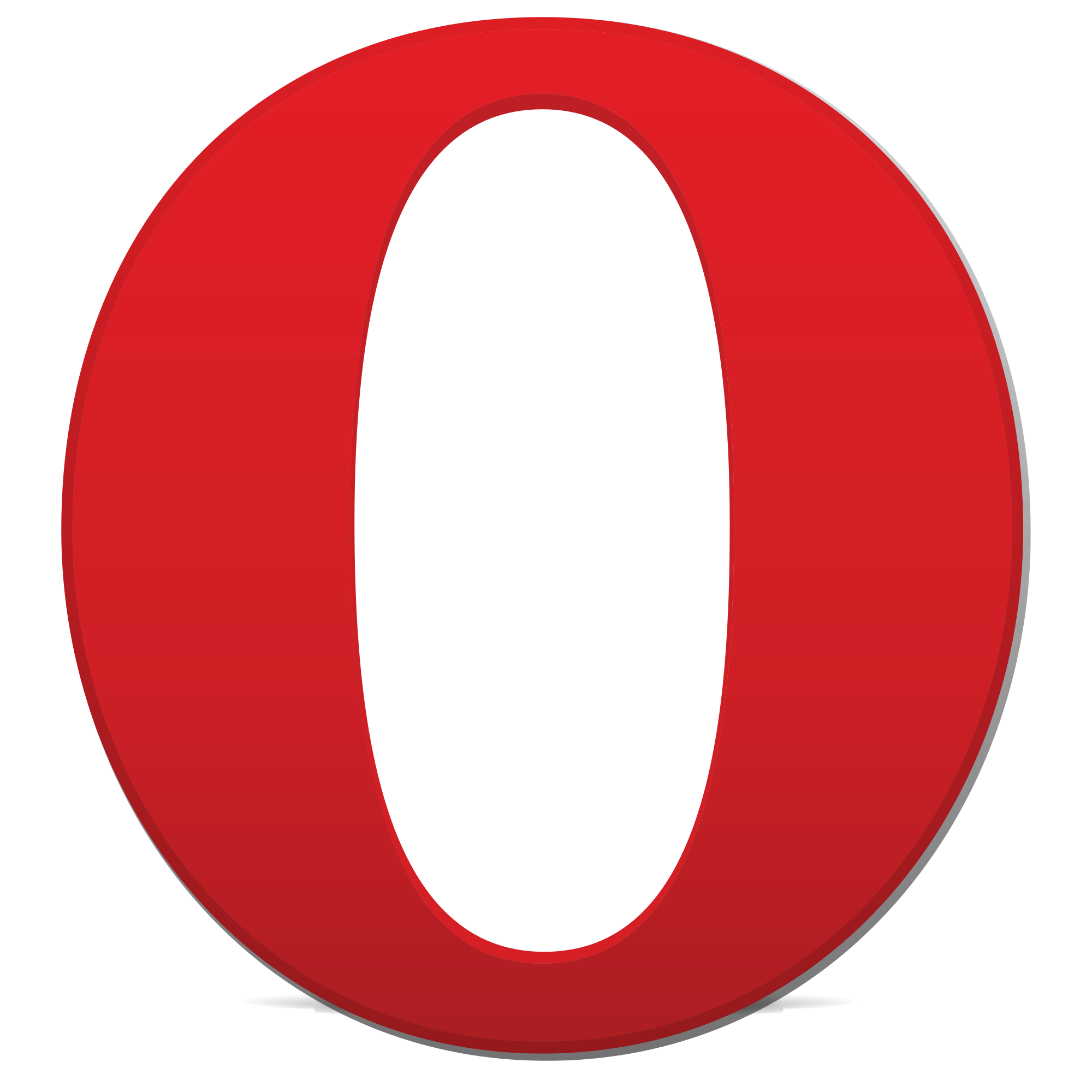
Opera
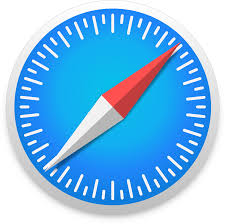
Safari
Mobile browser support
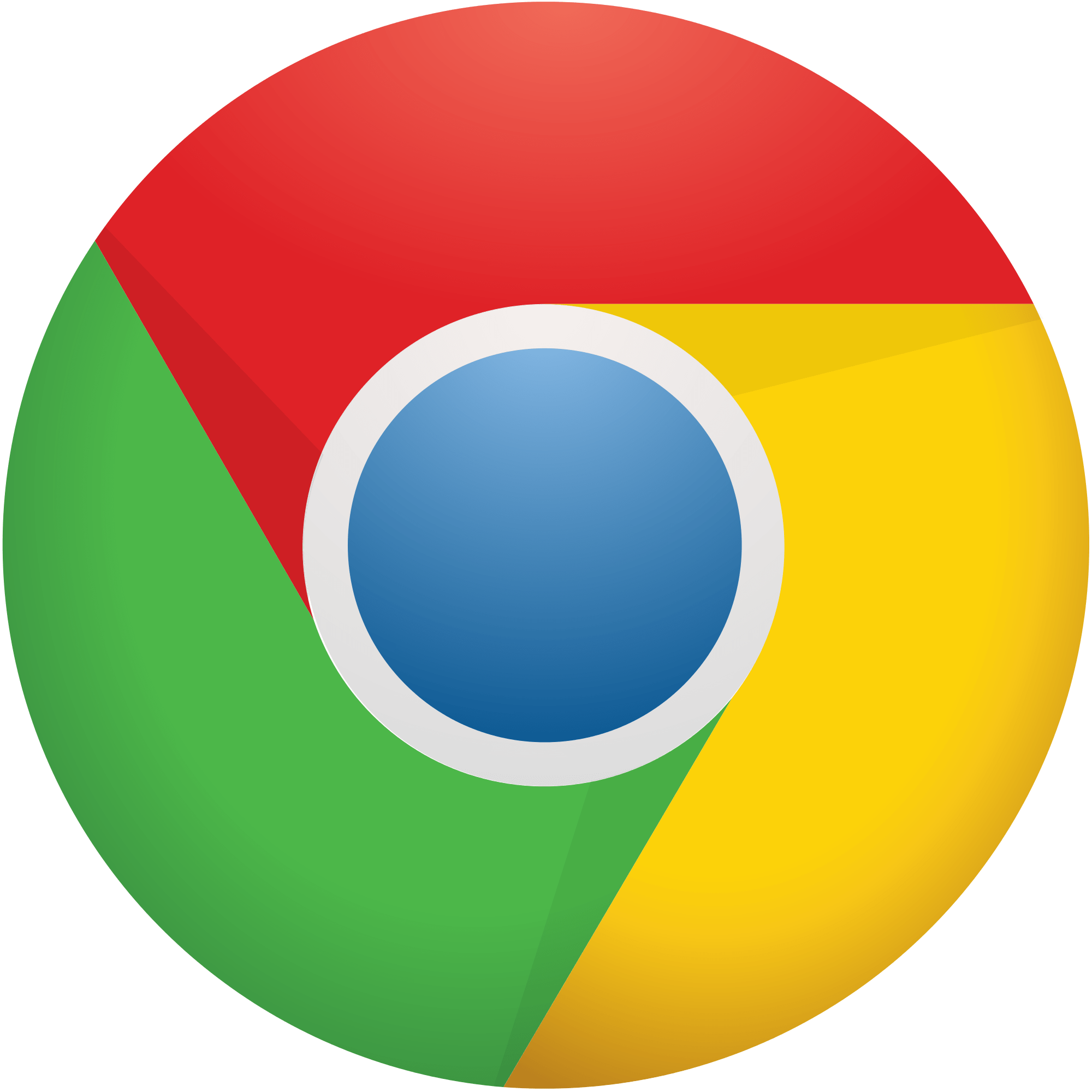
Chrome
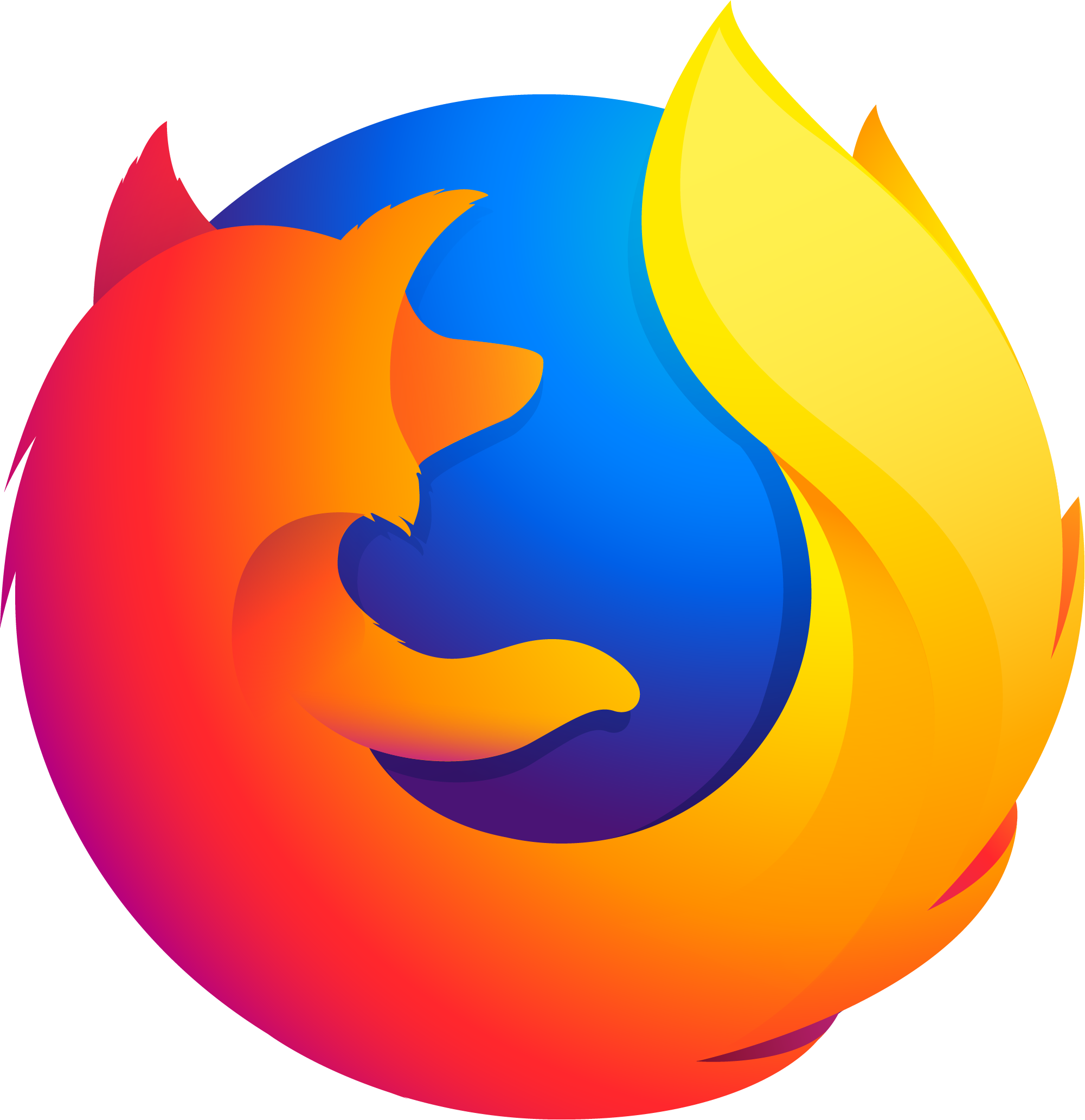
Firefox
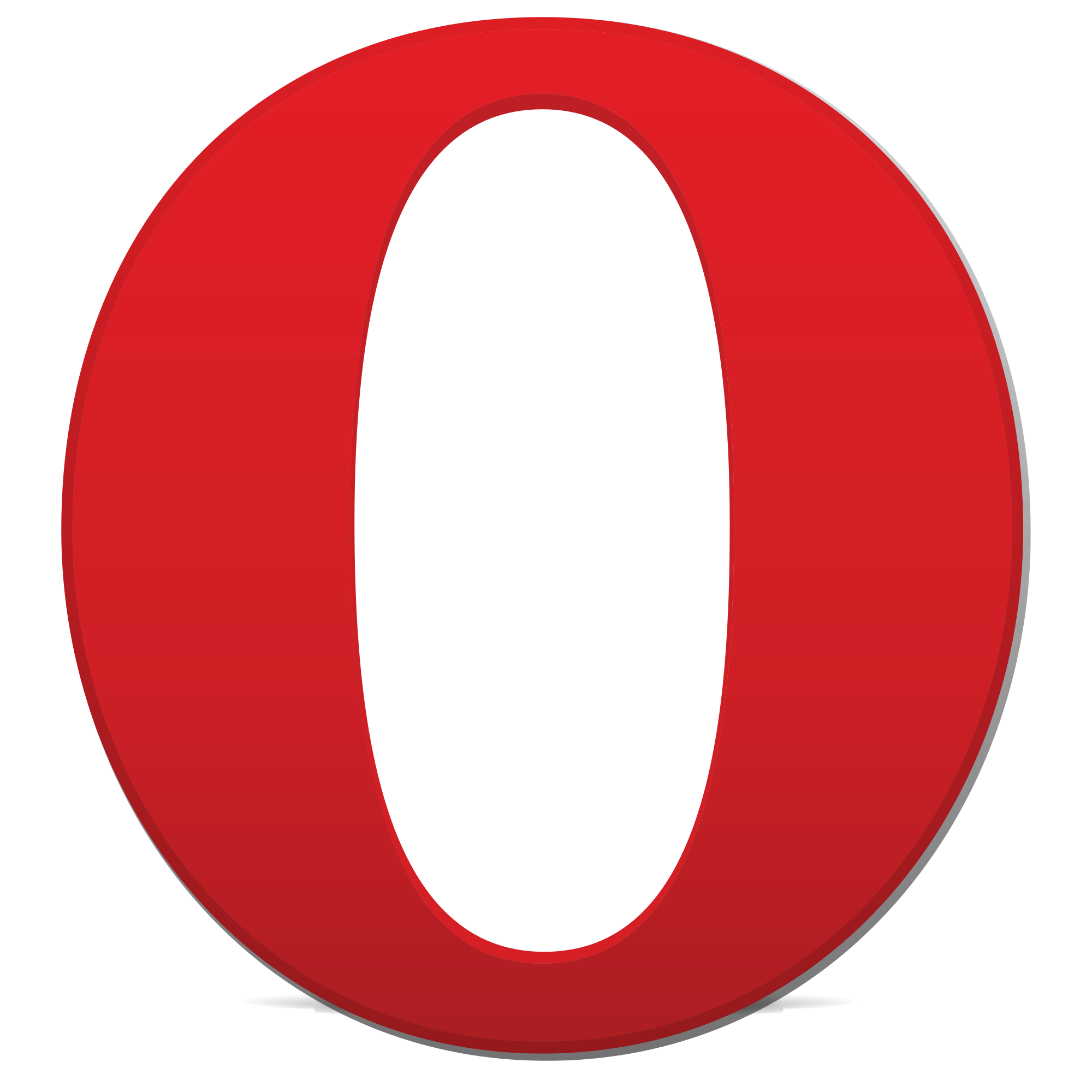
Opera
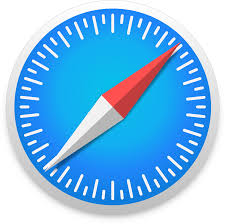