TL;DR – HTML table contains tabular data in multiple rows and columns.
Contents
A Basic HTML Table Example
A table in HTML is defined using <table> tags. The whole content of the table must be included within this element:
<table>
<tr>
<th>Name</th>
<th>Surname</th>
<th>Points</th>
</tr>
<tr>
<td>Kayle</td>
<td>Jax</td>
<td>42</td>
</tr>
<tr>
<td>Eve</td>
<td>Cho</td>
<td>14</td>
</tr>
</table>
HTML tables are meant to hold tabular data only: don't use them to modify the layout of the page.
Defining Table Structure with HTML Tags
To understand how to make a table in HTML, you must start with learning appropriate tags. The <table> element defines a table in an HTML document. All other HTML table tags must be included in this tag:
<table>
<tr>
<td>This is the first row in the first column</td>
<td>This is the first row in second column</td>
</tr>
<tr>
<td>This is the second row in the first column</td>
<td>This is the second row in the second column</td>
</tr>
</table>
The <tr> element sets a row in the HTML table. A row can contain two types of cells:
<table>
<tr>
<th>Fruits</th>
<th>Prices</th>
</tr>
<tr>
<td>Apple</td>
<td>$1.26</td>
</tr>
<tr>
<td>Banana</td>
<td>$0.60</td>
</tr>
</table>
The <caption> element specifies the main title of the HTML table. While <th>
defines a header for a part of table's content, <caption>
creates the name to define the whole table:
<table style="width: 100%; border: 1px solid;">
<caption>Average Price List of Fruits [July 2017]</caption>
<tr>
<th>Fruits</th>
<th>Prices</th>
</tr>
<tr>
<td>Apple</td>
<td>€0.60</td>
</tr>
<tr>
<td>Banana</td>
<td>€0.32</td>
</tr>
</table>
Note: <caption> should be included right after the <table> opening tag before any other content.
Table Head, Body and Footer
In every HTML document, there is a <head> section and a <body> section. HTML tables have a very similar structure, which you can define using <thead>, <tbody> and <tfoot> elements.
The <thead> element is used to group header content:
<table border>
<thead>
<tr>
<td>Purchase</td>
<td>Price</td>
</tr>
</thead>
</table>
The <tbody> tag defines the body of the HTML table. All content should be included in this element:
<table>
<tbody>
<tr>
<td>Long Island</td>
<td>45</td>
<td>50</td>
</tr>
<tr>
<td>Geeky stuff</td>
<td>85</td>
<td>90</td>
</tr>
<tr>
<td>StayAtHomeMart</td>
<td>25</td>
<td>30</td>
</tr>
<tr>
<td>BestBuy</td>
<td>60</td>
<td>10</td>
</tr>
</tbody>
</table>
The <tfoot> element defines the footer of the table. It must be placed in the beginning of the code – however, the browser will display it at the end of the table:
<tfoot>
<tr>
<td>Summary</td>
<td>It was mostly sunny.</td>
</tr>
</tfoot>
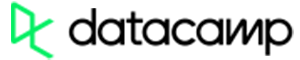
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
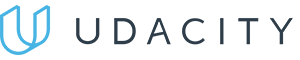
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
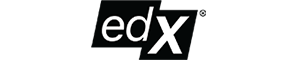
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Merging Cells with Attributes
In some cases, you might need to merge cells in your HTML table. This can be achieved using special attributes.
The colspan
attribute merges multiple cells in one row to create one:
<table>
<tr>
<th colspan="2">List of Fruit Prices</th>
</tr>
<tr>
<td>Apple</td>
<td>€0.60</td>
</tr>
<tr>
<td>Banana</td>
<td>€0.32</td>
</tr>
</table>
The rowspan
attribute acts in the same manner, but instead of merging cells in one table row, it spans through a column downwards:
<table>
<tr>
<th>Fruits</th>
<th>Prices</th>
<th rowspan="3">Month of June 2017</th>
</tr>
<tr>
<td>Apple</td>
<td>€0.6</td>
</tr>
<tr>
<td>Banana</td>
<td>€0.32</td>
</tr>
</table>
Styling HTML Tables
If you wish to style one or multiple columns in a different manner than the whole HTML table, you need to be familiar with <colgroup> and <col> elements. Using them allows you to assign specific inline styling for particular columns:
<table>
<colgroup>
<col span="1" style="background-color: cornsilk;">
<col style="background-color: bisque;">
</colgroup>
<tr>
<th>Price</th>
<th>Item</th>
<th>Quantity</th>
</tr>
<tr>
<td>100</td>
<td>Peanut Butter</td>
<td>2</td>
</tr>
</table>
Using CSS Properties
You can also create a custom table look using CSS internal styling. In this section, we will review the most common properties.
The border property creates an HTML table border:
In the example above, the border is doubled. This happens because the property adds a separate border to every cell of the table. However, you can use the border-collapse
property to merge the double border into a single line:
You can also keep the double border and style it. The border-spacing
property allows you to modify the spacing between the borders of separate cells in your HTML table:
By default, HTML tables do not have any styling to them. If you want to create spacing between your data, you can set it while using the padding property which is usually defined in pixels:
You can align the text in your HTML table by using the text-align property. You can set the alignment to the left, right or center:
Note: header cells already have a center alignment, and body cells are aligned to left by default.
HTML Table: Summary
- HTML table width depends on its content. It's a block-level element, but won't span through the whole page width automatically if the content takes up less space.
- Keep in mind that screen readers for disabled users announce the presence of HTML tables and read them from left to right and from top to bottom.
- Notice that <col> is an empty element, but <colgroup> is not, as it contains all the
<col>
elements.