TL;DR – HTML class attribute specifies a class name. You can use it to select and access HTML elements for various tasks performed using CSS and JavaScript.
Contents
Defining an HTML Class
Adding HTML class is no different to any other attribute. You have to include it in the opening tag and define the value wrapped in quotes:
Syntax | <tag class="name">content</tag> |
Example | <div class="bigger">Wash your hands!</tag> |
In HTML, you can also define multiple classes for a single element. All of them must be included in the same pair of quotes and separated using spaces:
Syntax | <tag class="name1 name2 name3 name4">content</tag> |
Example | <div class="harder better faster stronger">Wash your hands!</tag> |
Note: remember HTML classes are case-sensitive.
Using HTML Classes for CSS
The most common use for HTML classes is CSS styling. You can create a specific style and assign it to a particular class in HTML. All elements that have that class specified will inherit the style:
All the styling properties must be surrounded with <style> tags and placed in the head section of the document.
Note: remember to always include a period (.) in front so the system recognizes it as a CSS selector.
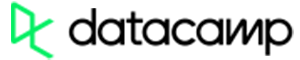
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
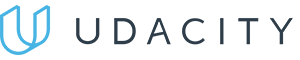
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
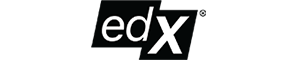
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Most Common Properties for Styling Classes
The color property defines the text color for the element. You can define colors by their names, RGB or HEX values:
To specify the background color, you can use background-color:
Tip: use a color picker to get the values for the exact tone you need.
To align an element, use text-align. There are three possible values for this property: left
, center
or right
:
The padding property creates space between the element's content and its border:
<style>
.bg-c {
background-color: #f3f3f3;
}
.pad {
padding: 20px 0 20px 0;
}
</style>
To add space between the element's border and the content outside it, you can use the margin property:
<style>
.bg-c {
background-color: #f3f3f3;
}
.mar {
margin: 0 0 0 50px;
}
</style>
Working With Classes in JavaScript
If you have some knowledge in JavaScript, you might recognize the className property. Developers use it to set or return the name of a class for an HTML element.
To access elements that have a particular HTML class name specified, you need to use the document.getElementsByClassName()
method in JavaScript:
var sampleElem1 = document.getElementsByClassName("sampleStyle")[0].className;
var sampleElem2 = document.getElementById("sampleDiv").className;
HTML Class: Useful Tips
- Some beginners get confused between using HTML
class
andid
attributes, as both can be used to access elements. Remember that an element can only have one ID which must be unique. Classes can be applied to multiple elements and also combined. - You can use any Unicode character for a class name (e.g., ♫ for music-related sections). However, it's not exactly a recommended practice: most developers advise staying with semantic names.