Every coder understands the importance of web security. Safety breaches can cause small inconveniences as well as substantial financial losses and privacy violations. That is why PHP form validation should also be considered a priority.
After users submit a form with their data, it is crucial to validate this input. In the past lessons, we already spoke about using simple and advanced PHP filters to validate and sanitize the data that might reach our code from outside.
Now we will learn to apply that knowledge to PHP forms. After all, they are specifically meant to collect external data provided by the visitors of your website. Using numerous PHP form validation examples, we will explain it to you step by step.
Contents
PHP Form Validation: Main Tips
- To ensure that your PHP form is secure, you need to set up form validation.
- If you set up PHP form validation properly, it will protect your database from SQL injection and exploitation.
How Forms Are Checked: Code Examples
To see how the PHP form processing works, let's first create an example of a form itself. It will include three input fields:
- Nickname (required): can only consist of letter characters and spaces.
- Feedback (optional): a multiline text field.
- Post anonymously? (required): one value must be chosen in order to submit the form.
This is the full HTML form PHP code we'll use:
<form method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>">
Nickname: <input name="nickname" type="text">
Feedback: <textarea name="feedback" rows="4" cols="50"></textarea>
Post anonymously?
<input name="anon" type="radio" value="yes">Yes
<input name="anon" type="radio" value="no">No
<input name="submit" type="submit" value"submit">Submit</input>
</form>
Now this is a script for the PHP form validation:
<?php
// define variables and set to empty values
$nickname = $anon = $feedback = '';
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$nickname = test_input($_POST['nickname']);
$feedback = test_input($_POST['feedback']);
$anon = test_input($_POST['anon']);
}
function proc_input($input) {
$input = trim($input);
$input = stripslashes($input);
$input = htmlspecialchars($input);
return $input;
}
?>
First, we pass all of our submitted variables through htmlspecialchars()
and turn all special characters to HTML entities. It makes entries safe to process.
Second, we will use the trim()
function to remove extra spaces, tabs and newlines from the input data, followed by stripslashes()
to remove backslashes from the input. Instead of repeatedly writing these functions for each piece of input, we define them all in a single proc_input()
function and use it on each of them.
At the beginning of the script, we check whether the input was submitted using $_SERVER["REQUEST_METHOD"]
. Assuming REQUEST_METHOD
was POST
, the form is submitted and validated. Otherwise, a blank form will be displayed.
Input Elements to Validate
There are several options of the way input can be required from users. One of the most common types is the text field. However, you can customize your forms with radio buttons as well.
Text Fields
To create text input fields, we will use HTML <input>
tag and assign the name attribute. That way we will be able to reference the input field in our PHP code. Take a look:
Nickname: <input name="nickname" type="text" >
Feedback: <textarea name="feedback" rows="4" cols="50"></textarea>
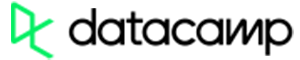
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
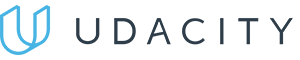
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
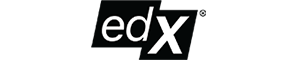
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Radio Buttons
Radio buttons are used when a user has to choose a single option from a selection provided. To create two of them, create two input elements with a similar name attribute. Don't forget to assign them their respective value attributes!
View an example to see how your code should look like:
Post anonymously?
<input name="anon" type="radio" value="yes">Yes
<input name="anon" type="radio" value="no">No
Form Element
To create an HTML form PHP element that is resistant to various exploits connected to PHP form validation and submision, we will use the htmlspecialchars()
function to turn special characters (such as angle brackets <>) to HTML entities. The method we assign to this form is POST, since our goal is to upload data.
The $_SERVER["PHP_SELF"]
is a global variable, which we use to reference the page we're currently on when using the htmlspecialchars()
function:
<form method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>">
Note: The htmlspecialchars() function is a great tool for avoiding $_SERVER["PHP_SELF"] exploits because it prevents attackers from entering special characters that may allow them to enter code to your website.
PHP SELF: Security Notes
Beware of the variable called $_SERVER["PHP_SELF"]
.
By using PHP_SELF
in the current page and entering the /
symbol, an attacker can use Cross Site Scripting (XSS) commands.
Note: Cross-site scripting (XSS) is a common security fault that most web applications have. Using XXS, an attacker can change client side script on your website.
Imagine this is the form element in our file form.php:
<form method="post" action="<?php echo $_SERVER["PHP_SELF"];?>">
If a normal URL is entered, the code above will be turned into something similar to this PHP form validation example:
<form method="post" action="form.php">
Well, that would happen normally. However, someone could enter a URL like this one:
http://www.example.com/form.php/%22%3E%3Cscript%3Ealert('hacked')%3C/script%3E
Then, the code above would be turned into something like this:
<form method="post" action="form.php/"><script>alert('hacked lmao')</script>
In this case, the URL enters a command, which makes our site display a JavaScript alert saying hacked. The lesson here is that using the PHP_SELF
is a weak spot that might be easy to exploit.
Keep in mind that by adding <script>
tags, a hacker can run JavaScript code on your site. This invasion would let them harm your database or website.
PHP Form Validation: Summary
- Form validation is crucial: without it, you can't be completely sure your forms are protected.
- If you take your time to prepare PHP validation, you might avoid SQL injection and exploitation of your databases.
- Using PHP SELF, attackers can damage your website if you skip crucial PHP form validation steps.