Contents
JavaScript className: Main Tips
- This property is used to set or return the name of a class of an HTML element.
- It is very similar to the
classList
property. - Multiple assigned classes have to be separated by spaces.
className Explained
You might recognize the term class as representing an attribute in elements of HTML. However, JavaScript className is a property and not an attribute. It can be used to perform a JavaScript change class function. Aside from that, it can be used to retrieve a class of HTML elements. Therefore, it allows developers to manage the class attribute quickly.
Another important aspect of this property is the usage of document.getElementsByClassName()
function which retrieves a live array consisting of child elements that all belong to the same specified class.
When this method is applied to the document object, document.getElementsByClassName()
will search the entire document (root node as well). After this method is invoked, a developer will receive an HTMLCollection of elements found.
The example below shows how you can make JavaScript set class for an element:
document.getElementById("sampleDiv").className = "sampleStyle";
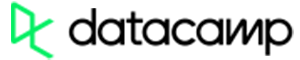
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
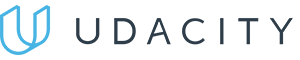
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
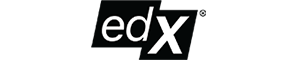
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Syntax
As you have learned from the previous section, JavaScript className property can retrieve or set a class to HTML elements. The syntax of this property is not difficult, and you should be able to memorize its capitalization and structure quickly.
However, we should briefly explain how multiple class names should be set. To avoid mistakes, you should separate each class name with a space between them so the code would be read correctly.
To return the className property, use HTMLElementObject.className
. Now, to set the JavaScript className property, choose HTMLElementObject.className = class
.
Values
The JavaScript className property accepts one value. It is called class and it is used to define a class of an element. However, when you want to JavaScript change class, remember that multiple classes have to be separated by spaces to be assigned (for example, class1 class2
).
The return value className provides is a string which represents the class, or a space-separated class list of a specified element in case it has multiple classes.
Learn from Code Examples
Learning theory is one thing: you should not wait around and practice what you have learned. Therefore, click Try it Live buttons to take this lesson to the code editor. Most of the code examples demonstrate how to modify or retrieve information about <div>
.
The first code example illustrates the way the element class is retrieved:
var sampleElem1 = document.getElementsByClassName("sampleStyle")[0].className;
var sampleElem2 = document.getElementById("sampleDiv").className;
With the following code, you can retrieve the information about elements that have multiple classes:
<div id="sampleDiv" class="sampleStyle">What a splendid Div element!</div>
document.getElementById("sampleDiv").className = "newSampleClass";
In case you want to add a new class to an element and keep the old one, you follow this code snippet:
document.getElementById("sampleDiv").className += "anotherSampleClass";
This example shows how you can change the font size of the element:
var sampleElem = document.getElementById("sampleDiv");
if (sampleElem.className === "sampleStyle") {
sampleElem.style.fontSize = "20px";
}
The following code example explains how you can toggle between two class names. The function will search for sampleStyle
in the <div>
and replace it with sampleStyle2
:
function sampleFunction(){
var sampleElem = document.getElementById("sampleDiv");
// If "samplestyle" exists, it is overwritten it with "sampleStyle2"
if (sampleElem.className === "sampleStyle") {
sampleElem.className = "sampleStyle2";
} else {
sampleElem.className = "sampleStyle";
}
}
If you want to toggle between two classes and make modifications to them according to scroll position, follow this example:
window.onscroll = () => sampleFunction();
function sampleFunction() {
if (document.body.scrollTop > 100) {
document.getElementById("samplePara").className = "test";
} else {
document.getElementById("samplePara").className = "";
}
}