Contents
JavaScript setInterval: Main Tips
- The JavaScript
setInterval()
method executes a specified function multiple times at set time intervals specified in milliseconds (1000ms = 1second). - The JS
setInterval()
method will keep calling the specified function untilclearInterval()
method is called or the window is closed. - The JavaScript
setInterval()
method returns an ID which can be used by theclearInterval()
method to stop the interval. - If you only need to execute a function one time, use the
setTimeout()
method.
Usage of JavaScript setInterval
JavaScript interval to be set use setInterval()
function. It could be defined simply as a method which allows you to invoke functions in set intervals.
The JS setInterval()
function is similar to the setTimeout method. How are they different? Well, setTimeout()
calls functions once. However, with the set interval method you can invoke them multiple times.
Let's say you need to display a message to your website visitors every 3 seconds. By applying the JavaScript setInterval()
function, you will be able to perform this task and incorporate a new feature on your website.
However, we would recommend not to overuse this function as it might disrupt the overall user experience. The following code example shows the way the message is set to be displayed every 3 seconds:
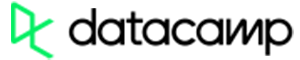
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
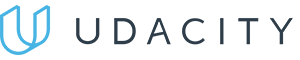
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
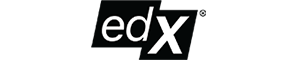
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Syntax to Follow
See the code snippet below. This is the syntax of the function that you should keep in mind:
setInterval(function, milliseconds, param_one, param_two, ...)
As you can see, the JavaScript setInterval()
can contain three parameters. The first one identifies which function is going to be applied in the set time intervals. As you might expect, you also should include the milliseconds to set the frequency of your function. Additionally, you can specify parameters for the function to be applied.
Parameter | Description |
---|---|
function | Required. Defines the function to run. |
milliseconds | Required. Defines how often the function will be executed (in millisecond intervals). |
param_one, param_two, ... | Not required. Defines any additional function parameters. |
Note: Any interval defined to be shorter than 10 milliseconds will be automatically changed to 10.
Stopping the Function
In some cases, you might need to make JavaScript stop setInterval()
from being executed before the times comes. You'll need to use the clearInterval()
method. It's meant to stop the timer set by using the setInterval JavaScript function.
The setInterval()
returns a variable called an interval ID. You can then use it to call the clearInterval()
function, as it's required by the syntax:
clearInterval(intervalId);
clearInterval()
itself has no return value: the only result is achieves is making JavaScript stop setInterval()
from running.
Code Examples to Analyze
For a more productive learning experience, we include useful code examples that you can practice using the setInterval JavaScript function.
The example below displays a popup alert every 2 seconds:
var myVar;
function myFunction() {
myVar = setInterval(every2sec, 2000);
}
function every2sec() {
alert("Alert Text!");
}
myFunction();
Now, this next example finds an HTML element with an ID clock
, and changes its text to show the current time every second. In other words, it works like a digital clock:
var idVar = setInterval(() => {
timer()
}, 1000);
function timer() {
var dateVar = new Date();
var time = dateVar.toLocaleTimeString();
document.getElementById("clock").innerHTML = time;
};
While this next example works exactly like the one above, it has a stopMyFunction
method. If you ran the function, it would stop the clock:
var idVar = setInterval(() => {
timer()
}, 1000);
function timer() {
var dateVar = new Date();
var time = dateVar.toLocaleTimeString();
document.getElementById("clock").innerHTML = time;
}
function myStopFunction() {
clearInterval(idVar);
}
You can you use the code in the example below to modify the width of a specified element until it's exactly 100px wide:
function move() {
var elem = document.getElementById("moveBar");
var width = 0;
var idVar = setInterval(change, 10);
function change() {
if (width == 100){
clearInterval(idVar)
}
else {
width++;
elem.style.width = width + '%';
}
}
}
Our last example toggles the background color from lime to red until the stopColor
function is executed:
var idVar = setInterval(() => {
setColor()
}, 400);
function setColor() {
var x = document.body;
x.style.backgroundColor = x.style.backgroundColor == "blue" ? "red" : "blue";
}
function stopColor() {
clearInterval(idVar);
}