Contents
JavaScript setTimeout(): Main Tips
- The JS
setTimeout()
method will call a function after the time specified in milliseconds (1000 ms = 1 second) has passed. - The specified function will be executed once. If you need to run a function multiple times, use the setInterval() method.
- To stop the timeout and prevent the function from executing, use the
clearTimeout()
method. - The JavaScript
setTimeout()
method returns an ID which can be used inclearTimeout()
method.
Usage and Purpose
Sometimes it is necessary to program a function call in a specific moment. The JavaScript setTimeout()
function is ready to help you with this task-scheduling by postponing the execution of a function until the specified time.
Let's say you are a developer who wants to enhance user experience in their website. One of the possible features to include is to schedule a message to be displayed after users scroll down to the end of the page. JavaScript setTimeout()
function can help you set timeout for the method and impress your website visitors with a new feature.
Please bear in mind that the setTimeout JavaScript function will execute functions once. If you want the function to be repeated multiple times, you should use setInterval()
.
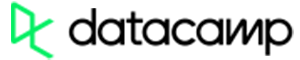
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
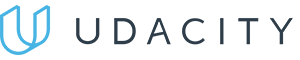
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
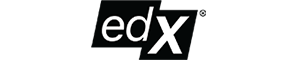
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Required Syntax
As you can see in the snippet below, the JavaScript setTimeout()
function can contain several parameters:
setTimeout(function, milliseconds, param_one, param_two, ...)
First of all, the setTimeout JavaScript method should contain the function that you intend to apply. The second parameter sets a time when the specified function is to be called. However, it is optional, and the default value is 0. You can also specify parameters for the function you have indicated previously.
Parameter | Description |
---|---|
function | Required. Specifies the function that will be executed. |
milliseconds | Not required. Specifies the time the function will be executed. 0 milliseconds by default. |
param_one, param_two, ... | Not required. Parameters passed to the function. |
Code Examples for Practice
To grasp the concept better, use the examples provided below. Don't forget to open them in the code editor and play around a little. This way, you'll get a much better understanding of how everything works.
The first example illustrates how JavaScript setTimeout()
function can open an alert box after 2 seconds:
var sampleVar;
function sampleFunction(){
sampleVar = setTimeout(alertFunc, 2000);
}
function alertFunc(){
alert("Two seconds have passed!");
}
This next example will change the text of an element every 2 seconds (3 times). For this to work properly, you must set an ID of some HTML element to counter
:
var x = document.getElementById("counter");
setTimeout(() => { x.innerHTML = "2 seconds" }, 2000);
setTimeout(() => { x.innerHTML = "4 seconds" }, 4000);
setTimeout(() => { x.innerHTML = "6 seconds" }, 6000);
The last example we prepared will stop the timeout if the clearTimeout
is called before the timer runs out:
var sampleVar;
function sampleFunction(){
sampleVar = setTimeout(() => {
alert("This timer will be stopped")
}, 3000);
}
function sampleStopFunction(){
clearTimeout(sampleVar);
}
sampleFunction();