Contents
JavaScript Input: Main Tips
- The JavaScript input text property is used to set or return the value of a text input field.
- The value property contains either the default value that is present upon loading the element, the value entered by the user, or the value assigned by the script.
textObject.value Property Explained
JavaScript .value
property can help you manage content on your websites. For instance, forms and dropdown lists are appreciated elements that website developers include in their pages to communicate with their visitors or retrieve information.
The following code example shows how the JavaScript input property can modify the value of a text field:
However, if you want to perform the JavaScript get input value task, you should remove everything that comes after equal sign. Then, you will not set the value but receive it.
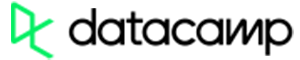
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
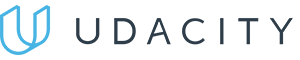
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
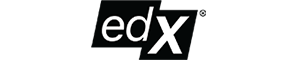
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Write it Correctly
We have two examples of get input value JavaScript usage. The first one holds a code which will return the value of the property. JavaScript get value of input:
textObject.value
The second example can be used for setting the value of the property. As you can see, it contains a value called text
, indicating the value of the text input field:
textObject.value = text
Just like many other JavaScript properties, this one will also generates a return value. The JavaScript input text value property will produce a string, containing the value of the text field.
Useful Code Examples for Practice
If you are only starting to learn the get input .value
JavaScript, we recommend that you start by practicing with the code examples we provide in this section. While the first one might seem rather long, it illustrates how form validation can be achieved:
var email = document.getElementById("email").value.indexOf("@");
var age = document.getElementById("age").value;
var firstName = document.getElementById("firstName").value;
Submitted = "true";
if (firstName.length > 10) {
alert("The name must have no more than 10 characters");
Submitted = "false";
}
if (isNaN(age) || age < 12 || age > 100) {
alert("The age must be between numbers 12 and 100");
Submitted = "false";
}
if (at == -1) {
alert("The email address you entered is not valid");
Submitted = "false";
}
if (Submitted == "false") {
return false;
}
If you click Try it Live button, you will see that the text input field contains a dropdown list:
var sampleList = document.getElementById("sampleList");
document.getElementById("favoriteElem").value = sampleList.options[sampleList.selectedIndex].text;
This example shows a different type of dropdown list in the text input field. See for yourself and compare:
var none = document.getElementById("none");
var option = none.options[none.selectedIndex].text;
var sampleText = document.getElementById("resultElem").value;
sampleText = sampleText + option;
document.getElementById("resultElem").value = txt;
Now, the following example illustrates how default value and value property differ:
var textVal = document.getElementById("sampleText");
var defaultTextVal = textVal.defaultValue;
var currentTextVal = textVal.value;