JavaScript, as any other programming language, uses data types. There are strings, numbers, objects, arrays and others. Knowing what each of them represents and understanding how you can work with them allows you to have more control over the code you're writing or reading.
You may find it useful to know to manipulate data by converting it from one type to another. You may need to use JavaScript convert to string in some cases or convert string to numbers in others. In this tutorial, you will get familiar with JavaScript type conversions and learn to turn each of the data types to a different one.
Contents
JavaScript Type Conversion: Main Tips
- The three main types of conversion are
String()
,Number()
andBoolean()
. They convert data to the type of their respective names. - It is not possible to detect if the type of a JavaScript object is a date or an array by using
typeof
. For both date and array, the type ofobject
will be returned.
Possible Types
There are five types of data that are able to contain a value:
- number type
- boolean type
- string type
- function type
- object type
There are also three object types:
- object type
- date type
- array type
Finally, there are two types that cannot hold values:
- undefined type
- null type
typeof Operator
typeof
operator is used when you need to know the type of data in a JavaScript variable. That is crucial before performing any JavaScript type conversions:
Keep in mind:
- The type of data
NaN
isnumber
. - The type of data of array is
object
. - The type of data in date is
object
. - The type of data of
null
isobject
. - The type of data of an
undefined
variable isundefined
. - The type of data of a variable that doesn't have a value is
undefined
.
typeof "Joe" // Returns "string"
typeof 4 // Returns "number"
typeof NaN // Returns "number"
typeof false // Returns "boolean"
typeof [4,3,2,1] // Returns "object"
typeof {name:'Joe', age:45} // Returns "object"
typeof new Date() // Returns "object"
typeof function () {} // Returns "function"
typeof phone // Returns "undefined"
typeof null
constructor Property
This property brings back the constructor
function for all variables in JavaScript:
"Bob".constructor
(4.17).constructor
true.constructor
[1,2,3,4,5].constructor
{name:'Bob',age:28}.constructor
new Date().constructor
function () {}.constructor
It can be used to find out the object type (array and date):
function isArray(myArray) {
return myArray.constructor.toString().indexOf("Array") > -1;
}
function isDate(date) {
return date.constructor.toString().indexOf("Date") > -1;
}
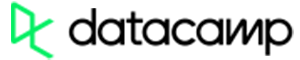
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
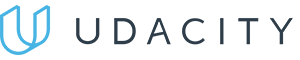
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
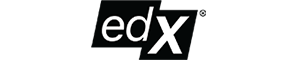
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
JavaScript Type Changes
A variable in JavaScript can be changed to different variables and types of data:
- By using a JavaScript function
- Automatically, by JavaScript
We will first cover using functions for type conversions. Each explanation will be followed by an example. Make sure not to skip those, as they are important in grasping the idea of how a certain function works!
Numbers to Strings
It's easy to modify numbers using JavaScript convert to string functionality with the String()
method. You can use it on various types of expressions, literals, variables, or numbers:
String(z) // brings back a string from a number variable z
String(321) // brings back a string from a number literal 321
String(300 + 23) // brings back a string from a number from an expression
To convert to string JavaScript toString()
method might also be used:
Booleans to Strings
You can modify booleans using JavaScript convert to string functionality with the String()
method:
String(false) // brings back "false"
String(true) // brings back "true"
Again, to convert to string JavaScript toString()
method might come in handy:
false.toString() // brings back "false"
true.toString() // brings back "true"
Dates to Strings
You can change dates using JavaScript convert to string functionality with the String()
method:
String(Date()) // brings back "Thu Aug 107 2017 14:37:20 GMT+0100 (E. Europe Daylight Time)"
ToString()
may be used the same way:
Date().toString() // brings back "Thu Aug 07 2017 14:34:20 GMT+0200 (E. Europe Daylight Time)"
Strings to Numbers
When changing strings to numbers, we use the Number()
method.
A string with "3.18"
gets changed to 3.18
. Strings with nothing inside are changed to 0
. Everything else is changed to NaN
:
Number("3.18") // brings back 3.18
Number(" ") // brings back 0
Number("") // brings back 0
Number("22 44") // brings back NaN
The Unary + Operator
When changing variables to numbers, we use Unary + operator:
The variables that can't be changed appear as NaN
number:
var z = "Bob"; // z is a string
var d = + d; // d is a number (NaN)
Booleans to Numbers
To perform JavaScript convert to number on a boolean, we can use the Number()
method:
Dates to Numbers
When executing JavaScript convert to number on a date, we use Number()
method:
getTime()
can also be used:
Automatic Changes
As we mentioned above, some changes also happen automatically in JavaScript. Let's pay those some attention, shall we?
JavaScript tends to change the value of the data type it is working on when the type is wrong. Sometimes it gives different results:
2 + null // brings back 2 because null is changed to 0
"2" + null // brings back "2null" because null is changed to "null"
"2" + 1 // brings back 22 because 1 is changed to "1"
"2" - 1 // brings back 1 because "2" is changed to 2
"2" * "4" // brings back 8 because "2" and "4" are changed to 2 and 4
When outputting a variable or an object, JavaScript calls out the toString()
variable's function by default:
{name:"Fbob"}.toString() // toString changes to "[object Object]"
[1,2,3,4,5].toString() // toString changes to "1,2,3,4,5"
new Date().toString() // toString changes to "Thu Aug 07 2017 14:54:55 GMT+0200"
It might not be clearly visible, but booleans and numbers are changed, too:
var number = 123;
number.toString() // toString changes to "323"
true.toString() // toString changes to "true"
false.toString() // toString changes to "false"
Conversion Table
It's always more convenient to have all the data in one place for easy referral, right? Especially when there's quite a bit of it. We couldn't agree more!
In the table below, we can see the changes of boolean, string, and number. Notice what happens if we change JavaScript string to boolean, as we didn't cover it with separate examples:
Original Values | Changed to Numbers | Changed to Strings | Changed to Booleans |
---|---|---|---|
false | 0 | "false" | false |
true | 1 | "true" | true |
0 | 0 | "0" | false |
1 | 1 | "1" | true |
"0" | 0 | "0" | true |
"1" | 1 | "1" | true |
NaN | NaN | "NaN" | false |
Infinity | Infinity | "Infinity" | true |
-Infinity | -Infinity | "-Infinity" | true |
"" | 0 | "" | false |
"10" | 10 | "10" | true |
"thirty" | NaN | "thirty" | true |
[ ] | 0 | "" | true |
[10] | 10 | "10" | true |
[40,50] | NaN | "40,50" | true |
["thirty"] | NaN | "thirty" | true |
["fifty","thirty"] | NaN | "fifty,thirty" | true |
function(){} | NaN | "function(){}" | true |
{ } | NaN | "[object Object]" | true |
null | 0 | "null" | false |
undefined | NaN | "undefined" | false |
Note: try to avoid JavaScript string to boolean conversions as a beginner, as it's easy to mess up and create bugs in result.
JavaScript Type Conversion: Summary
- You can apply JavaScript convert to string, number and a boolean.
- Array and date are of the object data type.
- Most data types contain values, but
undefined
andnull
don't. typeof
is an operator, so it does not contain a data type.