One of the many features of JavaScript is knowing how to work with nodes. Once you access the Document Object Model (DOM) nodes of specific elements, you can manage their properties, orientation, and appearance. In this tutorial, you will learn all about JavaScript HTML Document Object Model Navigation.
DOM nodes help you analyze relations between elements. Because of the element nesting, a node tree is created to provide better visualization. The structure and relationships between nodes will be explained as well. You will find out what a root, parent, child, and a sibling is in a node tree.
Contents
JavaScript HTML DOM Navigation: Main Tips
- The HTML DOM allows you to navigate the node tree by making use of the node relationships.
- By learning to navigate the document object model (DOM), you can make use of every object in the HTML that your JavaScript will be applied to.
What are Nodes and How are They Connected
The HTML Document Object Model standard says that everything in HTML documents is a node:
- The document itself is a document node.
- Each element is an element node.
- All of the text inside the elements are text nodes.
- Each attribute assigned to an element is an attribute node.
- Each comment is a comment node.
Using the HTML document object model, each of these nodes is accessible to JavaScript. You can create new nodes, and each node can be deleted or modified.
The nodes are connected by hierarchical relationships in the DOM tree. The terms parent, child, and sibling are used to describe this relationship:
- Root is the top node of the tree.
- Parent is an element that contains other elements. Every element has one, except for the root.
- Child is below, or contained by, the parent. A parent may have any number of child nodes.
- Siblings are nodes under the same parent.
Let's illustrate this with an example:
<html>
<head>
<title>Amazingly Creative Title</title>
</head>
<body>
<h2>Wonderful Heading</h2>
<div>The text!</div>
</body>
</html>
In an example of an HTML script above, you can see that:
<html>
is the root node, has no parent and is the parent of<head>
and<body>
.<head>
and<body>
are children of<html>
.<title>
is a child of<head>
and has a single child, which is a text nodeAmazingly Creative Title
.<body>
has two children:<h2>
and<div>
.<h2>
has a single child text node:Wonderful Heading
.<div>
has a single child text node:The text!
.<h2>
and<div>
are siblings.
Navigating Between Nodes
The following node properties allow you to navigate the DOM tree using JavaScript:
childNodes[nodeNumber]
parentNode
lastChild
firstChild
previousSibling
nextSibling
Expecting an element node to contain text is a frequent error when working with the document object model.
For example: in the case of <title>Amazingly Creative Title</title>
, the element node <title>
itself contains no text. However, it does contain a text node Amazingly Creative Title
.
This can be accessed by using the nodeValue
property, or innerHTML
.
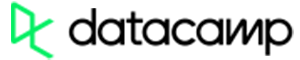
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
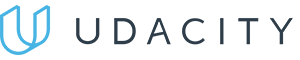
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
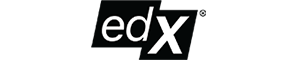
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Child Nodes and Node Values
While the innerHTML
property is handy for changing the content of an element, childNodes
and nodeValue
properties can be used to get the element's content.
The code in the example below gets the value of <h2>
element and copies it into a <div>
element:
<html>
<body>
<div id="el1">Amazingly Creative Heading</div>
<div id="el2">Look this text DOM!</div>
<script>
document.getElementById("el2").innerHTML = document.getElementById("el1").childNodes[0].nodeValue;
</script>
</body>
</html>
In the example above, getElementById
is a method, meanwhile childNodes
and nodeValue
are properties.
For the purposes of this tutorial, we are going to use the innerHTML
property. This does not mean the above method is somehow less useful for learning and understanding the DOM's underlying structure!
Keep in mind that using firstChild
has a similar effect as childNodes[0]
.
<html>
<body>
<h2 id="el1">Amazingly Creative Title</h2>
<div id="el2">the Text!</div>
<script>
document.getElementById("el2").innerHTML = document.getElementById("el1").firstChild.nodeValue;
</script>
</body>
</html>
DOM Root Nodes
There are two JavaScript get body element properties that allow you to access the document node itself:
document.body
is used for getting the document's body.document.documentElement
used to get the whole document.
Look at the examples below to see both of them used in code:
<html>
<body>
<script>
alert(document.body.innerHTML);
</script>
<p>Amazingly Creative Title</p>
<div>
<p>Hail the DOM!</p>
<p>Witness the power of the <strong>document.body</strong> property.</p>
</div>
</body>
</html>
<html>
<body>
<script>
alert(document.documentElement.innerHTML);
</script>
<p>Check this out!</p>
<div>
<p>Hail the DOM!</p>
<p>Witness the power of the <strong>document.documentElement</strong> property!</p>
</div>
</body>
</html>
Properties You Should Know
The nodeName
property is used to specify the node's name and is always read-only. It can return these values:
- Tag name
- Attribute name
- #text (in case of a text node).
- #document (in case of a document node).
- #comment (in case of a comment node).
Note: nodeName will always contain the HTML element name in uppercase.
Now, the nodeValue
property is used to specify the value of a node. It has three possible return values:
- NULL (in case of a document or element node)
- Content (in case of a text or comment node)
- Attribute value (in case of an attribute node)
The nodeType
property is used to return the type of node. Review the table below to see the most important types of nodes:
Element type | nodeType |
---|---|
Element | 1 |
Attribute | 2 |
Text | 3 |
Comment | 8 |
Document | 9 |
JavaScript HTML DOM Navigation: Summary
- You can navigate the DOM with JavaScript through node relationships.
- Node relationships are defined as
root
,parent
,child
, andsibling
. - There are various node properties you can use to access nodes.