As you are already familiar with variables (if not, you should visit JavaScript Objects Tutorial), you know that they can contain objects. However, if you have multiple objects that you want to store, JavaScript arrays can be used.
In this tutorial, you will find everything about JavaScript array syntax and usage. You will understand when you should use arrays and when it's better to stick to regular variables. You will learn the differences between arrays and objects, and also the most common array properties and methods.
The process of creating a JavaScript multidimensional array might sound more confusing. However, managing a it is no different from a regular one.
Contents
JavaScript Array: Main Tips
- Javascript arrays are variables which contain multiple values (items).
- JavaScript array syntax is similar to regular variables.
- JavaScript arrays are used to store lists of items (names, titles, etc.).
Why and How to Create Arrays
When you need to describe a list of items (for example, phones), placing them in separate variables would look like this:
var phone1 = "Sony";
var phone2 = "Nokia";
var phone3 = "iPhone";
However, it is not convenient to create a new variable for each value. It's also not an option if you need a much larger number of items, for example, 300. Imagine how difficult it would be not to get lost among 300 different variables and objects!
To fix this problem, you can use the JavaScript arrays, as they can hold more than one value. Then, when you need to find a particular item in the array, you can simply use its index number.
Note: Arrays are useful when you have a list of values, which are of the same kind. Objects are better when you have a list of different types of values.
The syntax for creating JavaScript arrays is similar to regular JavaScript variables. All you have to do is:
- Declare a variable (
var
). - Give the variable a name (
array_name
). - Put an equal sign (
=
). - Open a square bracket (
[
). - List the values (items) (
["item1", "item2", ...]
). - Close the square brackets (
]
).
The final result should look like this:
var name_array = ["item1", "item2", ...];
Note: if the values are strings (text), you must encapsulate them in quotes. Otherwise, the item enlisted will be read by JavaScript not as a value, but another variable. This rule does not apply to numbers.
There are two ways to create arrays in JavaScript. One is by using an array literal method. The other is by using the new
keyword. Let's take a closer look at both.
Array Literal
All you have to do to create an array using the array literal method is to write everything according to the proper syntax described above! That doesn't seem too difficult. Let's see the example one more time to make sure we don't skip anything:
The item list can span in many lines, or stay in one. It does not make any difference to the functionality. Check out multiple line span in the example below:
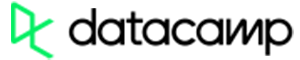
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
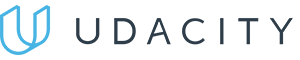
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
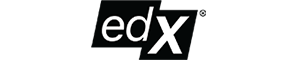
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
The Keyword new
However, there is one more way of creating an array. In the code below, you can see a JavaScript array created using the new
keyword:
Problems You Might Face
Both of the given examples of creating a JavaScript array have the same functionality. However, the first method is simpler, easier to read, and works faster than the second one. You should also keep in mind that array literal method is safer to use since there are a few problems that might occur when using the new
keyword.
var cars = new Array(); // Bad
var cars = []; // Good
Let's check out where these complications come from. In both examples, you can see the new Array()
method. The first one has two elements defined. As you can see, it doesn't cause issues:
// Create an array with two elements (40 and 100)
var cars = new Array(40, 100);
However, a problem arises if you only define one element which also happens to be a number. In the second example, you can see the number 50 is defined. However, the new Array()
method defines 50 undefined elements rather than one with a value of 50:
// Creates an array with 50 undefined elements
var score = new Array(50);
Handling Array Content
Since arrays are a particular kind of objects, they can store JavaScript objects, functions, and even other arrays as their content. Take a look at the example below to see a JavaScript array containing an object, a function, and an array:
Array[0] = Date.now;
Array[1] = myFunction;
Array[2] = myPhones;
Let's look at the variety of ways to can access and modify the items your JavaScript array holds.
Accessing Items
The index number is used to access a particular item in the array. The example below shows how to access the first item in phones
:
var phones = ["Sony", "Nokia", "iPhone"];
var name = phones[0];
Note: the index begins with 0 (not 1!) in JavaScript arrays. The first item is [0], the second [1], and so on.
In the example below, the index number person[0]
will return the first value in the array person
, which is John
.
Note: in a lot of programming languages, arrays with named indexes are supported and called JavaScript associative arrays. However, in JavaScript, only objects have named indexes: arrays can only have numbered indexes.
In a regular JavaScript object, in order to access a value, you'd have to use the objects name and its property name. In the example below, the property name car.firstCar
will return the first value from the object car
, which is Bmw
.
As you can see, the syntax is similar, but square brackets are changed to curly braces. Also, a value is assigned to the name property, and strings are stored in quotes.
Editing Items
In order to edit a particular item in an array, you have to use the index number. In the example below, the first item in phones
array is modified:
var phones = ["Sony", "Nokia", "iPhone"];
phones[0] = "Samsung";
Methods and Properties
Arrays in JavaScript have integrated methods and properties that you might use to your advantage. They look like this:
var y = phones.length; // The length property brings back the number of items
var z = phones.sort(); // The sort() method sorts arrays
Let's check out the most popular properties.
Length Property
This property shows the number of items stored in the array, also called the length of an array. This property can be very beneficial when there is a lot of items stored in an array, and you need to know exactly how many are there:
var cars = ["Bmw", "Audi", "Toyota", "Ferrari", "Lada"];
cars.length; // the length of cars is 5
Note: the amount is always the highest index number +1, because an array list starts with 0.
Adding Elements
The push()
method is very popular for inserting new elements into JavaScript arrays. In the example below, we use push()
to insert a new car name into an array:
var cars = ["Bmw", "Audi", "Toyota", "Ferrari"];
cars.push("Mercedes"); // adds a new item (Mercedes) to cars
This can also be achieved using length
:
var cars = ["Bmw", "Audi", "Toyota", "Ferrari"];
cars[cars.length] = "Mercedes"; // adds a new item (Mercedes) to cars
Looping Elements
for
is the loop mostly used for JavaScript array. It has its own syntax and functionality: if you want to know more about it, you can visit JavaScript For Loop tutorial. You can see how the for
loop is used to loop through a JavaScript array in the example below:
var cars, text, length, i;
cars = ["Bmw", "Audi", "Toyota", "Ferrari"];
length = cars.length;
text = "<ul>";
for (i = 0; i < length; i++) {
text += "<li>" + cars[i] + "</li>";
}
text += "</ul>";
JavaScript Array: Summary
- JavaScript array is a variable that can contain more than one object.
- There are two ways of defining an array in JavaScript, but it's consideed a better practice to use array literal method.
- JavaScript arrays can store functions, objects, and other arrays.
- Arrays have various useful methods and properties.