The main option for handling events in JavaScript is to use event handlers. The JavaScript addEventListener command allows you to prepare and set up functions that are going to be called when a specified event reaches its target.
In this tutorial, you will read all about JavaScript addEventListener. You will learn the proper syntax and all the ways this function can be used. You will also understand how this functionality can be added in your code. The tutorial will also cover event bubbling and capturing, which are important concepts to grasp.
Contents
JavaScript addEventListener: Main Tips
- JavaScript provides event handlers that allow you to set up the code to react to certain events occuring on certain HTML elements.
- JavaScript handlers of HTML DOM events can use not only HTML elements, but also any other DOM object.
- EventTarget method is case sensitive, so you should always use correct capitalization.
Description and Syntax
To attach a JavaScript event handler to a specific element, you have to use the JavaScript addEventListener()
method.
This method is specifically used to attach an event handler to a specified element in a way that doesn't overwrite other present event handlers. Multiple event handlers may be applied to a single element (for example, two click events might be assigned to the same element).
Any DOM object may be assigned a JavaScript event handler, which includes not only HTML elements, but, for example, the window itself as well.
The JavaScript addEventListener()
method can also make it easier to control the way an event reacts to bubbling.
JavaScript is separated from the markup of HTML when using the JavaScript addEventListener()
to improve readability, and will even allow adding event listeners without the control of the HTML markup. By using the removeEventListener()
method, event handlers can be easily removed:
document.getElementById("button").addEventListener("click", displayDate);
Syntax
Let's now look at the rules of syntax that apply and make sure we understand the parameters required:
element.addEventListener(event, functionName, useCapture);
We'll now explain it to you step by step:
- The first parameter specifies the event type (e.g.
"click"
or"mousedown"
). - The second parameter defines the function to be called and executed when the event occurs.
- The optional third parameter is a boolean value using which you may specify whether to use event capturing or bubbling.
Note: Unlike setting the event handlers through HTML attributes such as onclick, you don't need the on prefix here. All you need to write is click, needed to specify the type of the handled event.
Adding Event Handlers
JavaScript addEventListener()
provides you with a lot of different opportunities, which we will now review one by one. First, we will learn to simply add an event handler to an element. Then, we'll try adding more than one at once.
Once we get the idea, we'll see how event handlers should be applied to window objects. Carefully review the code examples provided to grasp the concepts.
Adding an Event Handler to an Element
When you add a JavaScript event listener, the function to be executed upon an event can be either anonymous or named. In the example below, you can see the anonymous function used. In this case, you need to define the code in the function:
In the example below you can see the named function being used. In this case, you are referencing an external function:
a.addEventListener("click", clickFunction);
function clickFunction() {
alert ("So that's what happens when you click.");
}
Add Many Event Handlers to the Same Element
The JavaScript addEventListener()
method lets you add multiple event handlers to a single element, while not overwriting any previously assigned event handlers. Take a look at the example below:
a.addEventListener("click", firstFunction);
a.addEventListener("click", secondFunction);
Different event types may be specified as well:
a.addEventListener("mouseover", mouseOverFunction);
a.addEventListener("click", clickFunction);
a.addEventListener("mouseout", mouseOutFunction);
Add an Event Handler to the Window Object
The JavaScript addEventListener()
method lets you add event listeners to HTML DOM objects, such as HTML elements, the document that the HTML is in itself, the window object, and any other object that supports events (like the xmlHttpRequest object).
window.addEventListener("resize", () => {
document.getElementById("text").innerHTML = Math.random();
});
Passing Parameters
When you want to pass values for the parameters, you may want to use an anonymous function which will call the specified function using the parameters.
a.addEventListener("click", () => {
clickFunction(param1, param2);
});
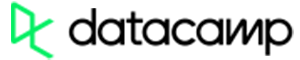
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
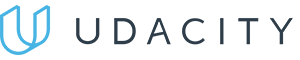
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
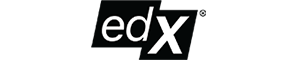
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Event Bubbling or Event Capturing?
Capturing and bubbling are the two ways of propagating HTML DOM events.
Event propagation defines the element order when an event occurs. For example, when you have an <img>
element in a <div>
, and the <img>
element is clicked, which click event will have to be handled first?
In the case of bubbling, the element that is on the lowest level event is handled first, and the outer ones afterwards. For example, the click event on <img>
, and the click event on <div>
after.
This order is reversed in the case of capturing the click event on <div>
is handled first, then the click event on <img>
.
When using the JavaScript addEventListener()
method you may set which propagation method will be used with the useCapture
parameter.
By default, this parameter is set to false
, meaning that bubbling will be used, and only uses capturing if this value is manually set to true
.
document.getElementById("pElement").addEventListener("click", clickFunction, true);
document.getElementById("divElement").addEventListener("click", clickFunction, true);
The removeEventListener() Method
This method will remove event handlers that was previously attached using JavaScript addEventListener()
method:
JavaScript addEventListener: Summary
- JavaScript event listener is simple, but requires a proper syntax.
- You can add multiple event listeners to the same element.
- You can specify whether you want event bubbling or capturing to be executed.
- You can easily remove the event listener with
removeEventListener()
method.
Browser support
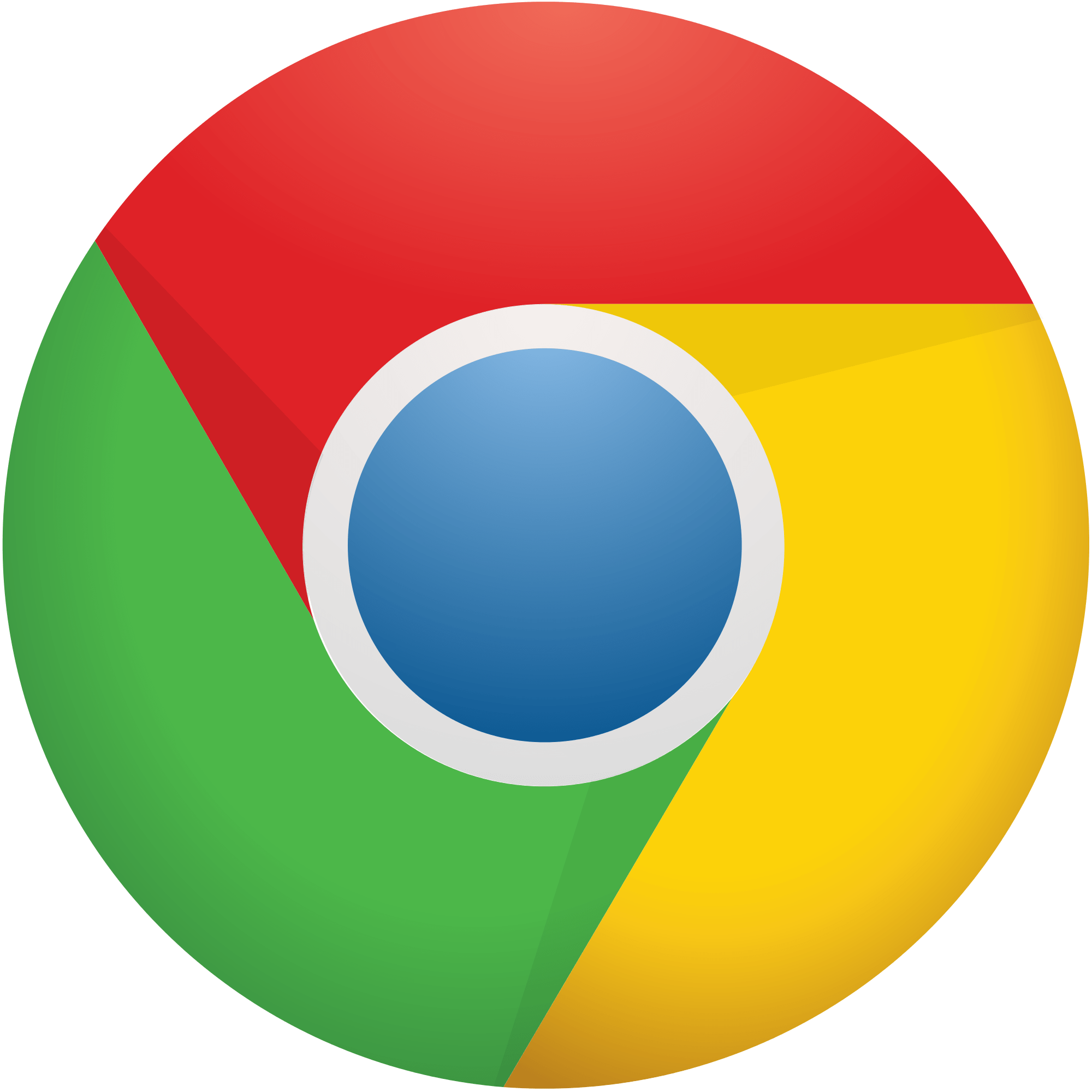
Chrome
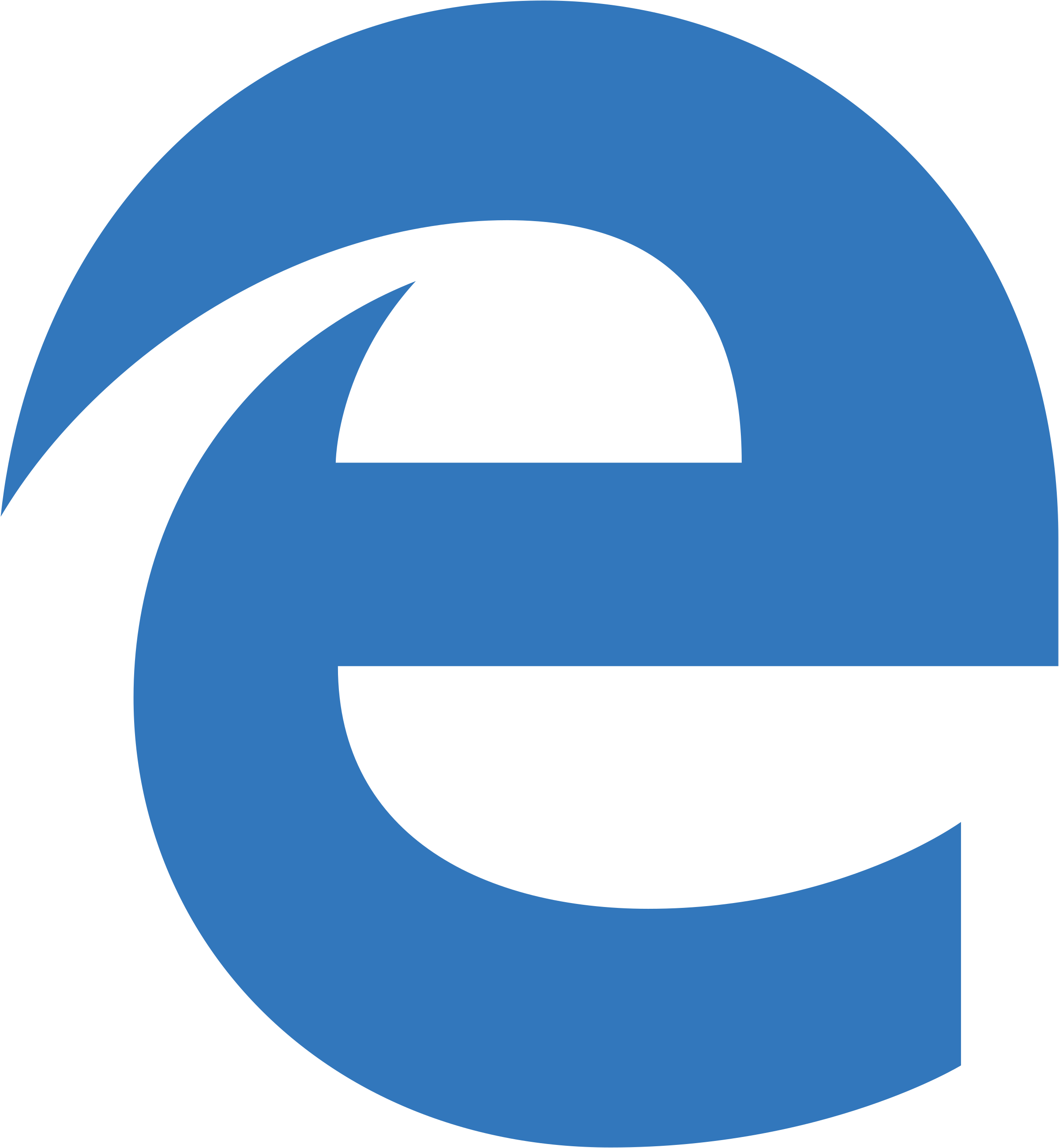
Edge
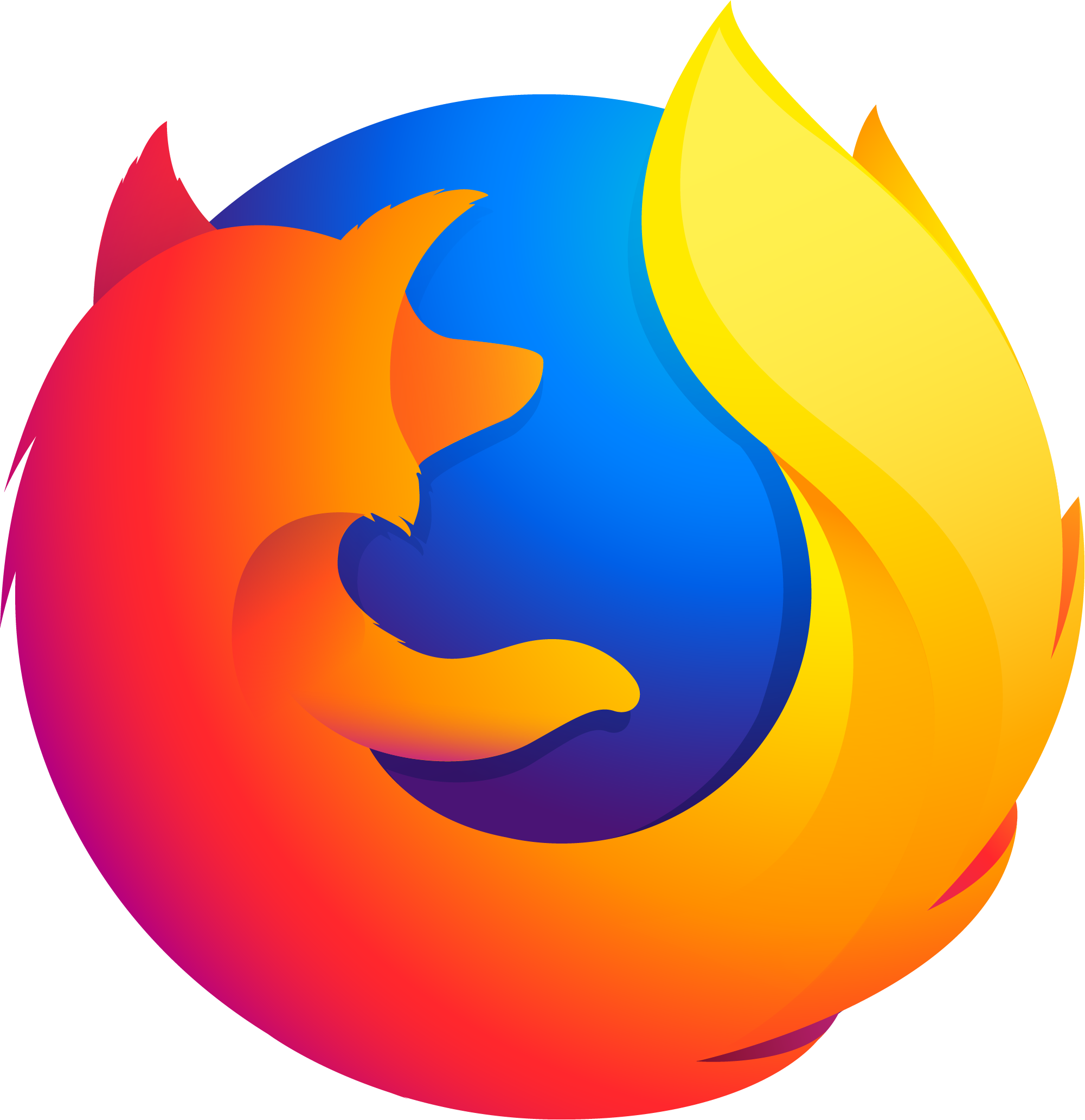
Firefox
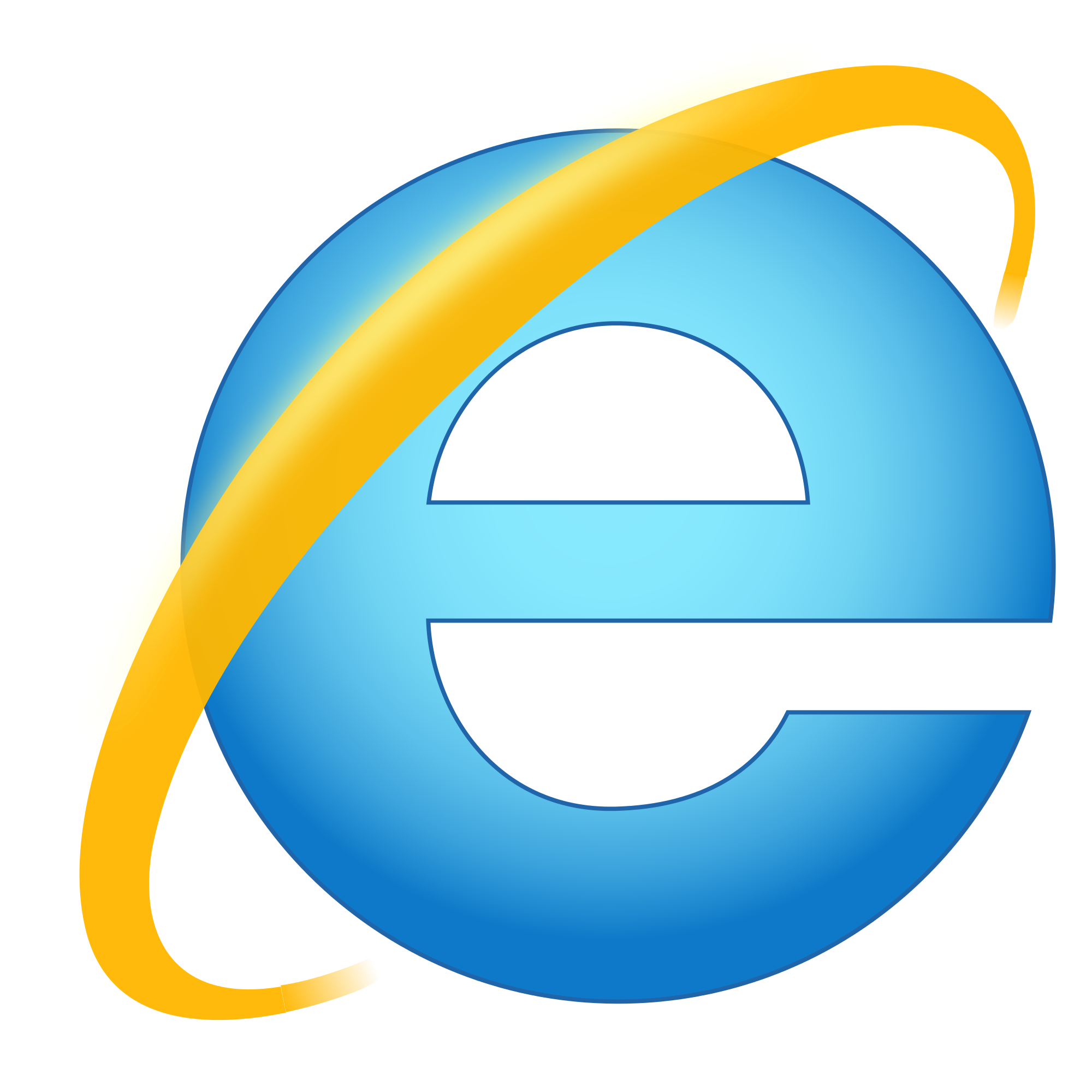
IE
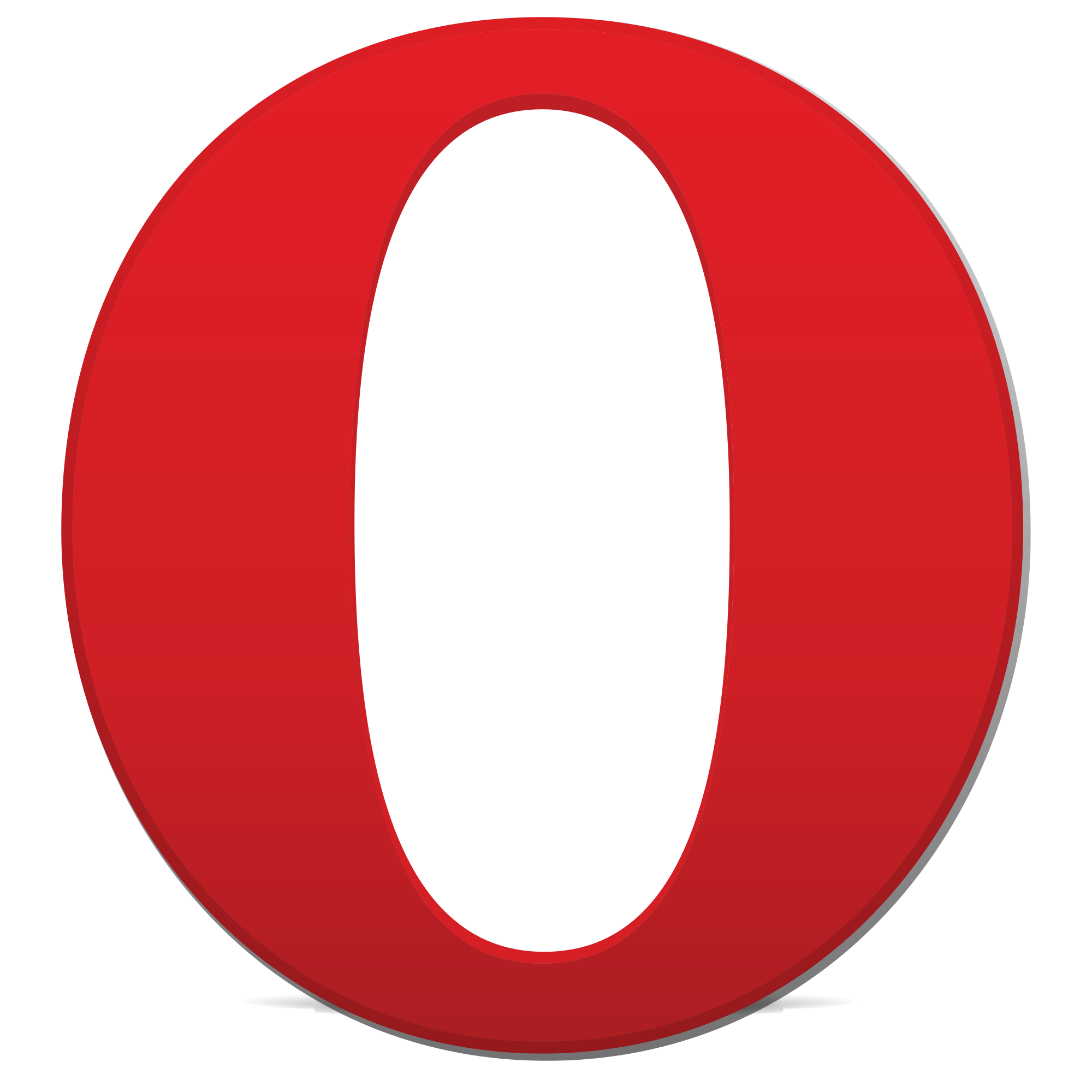
Opera
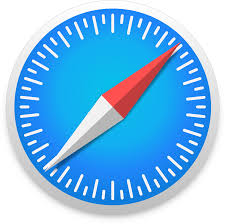
Safari
Mobile browser support
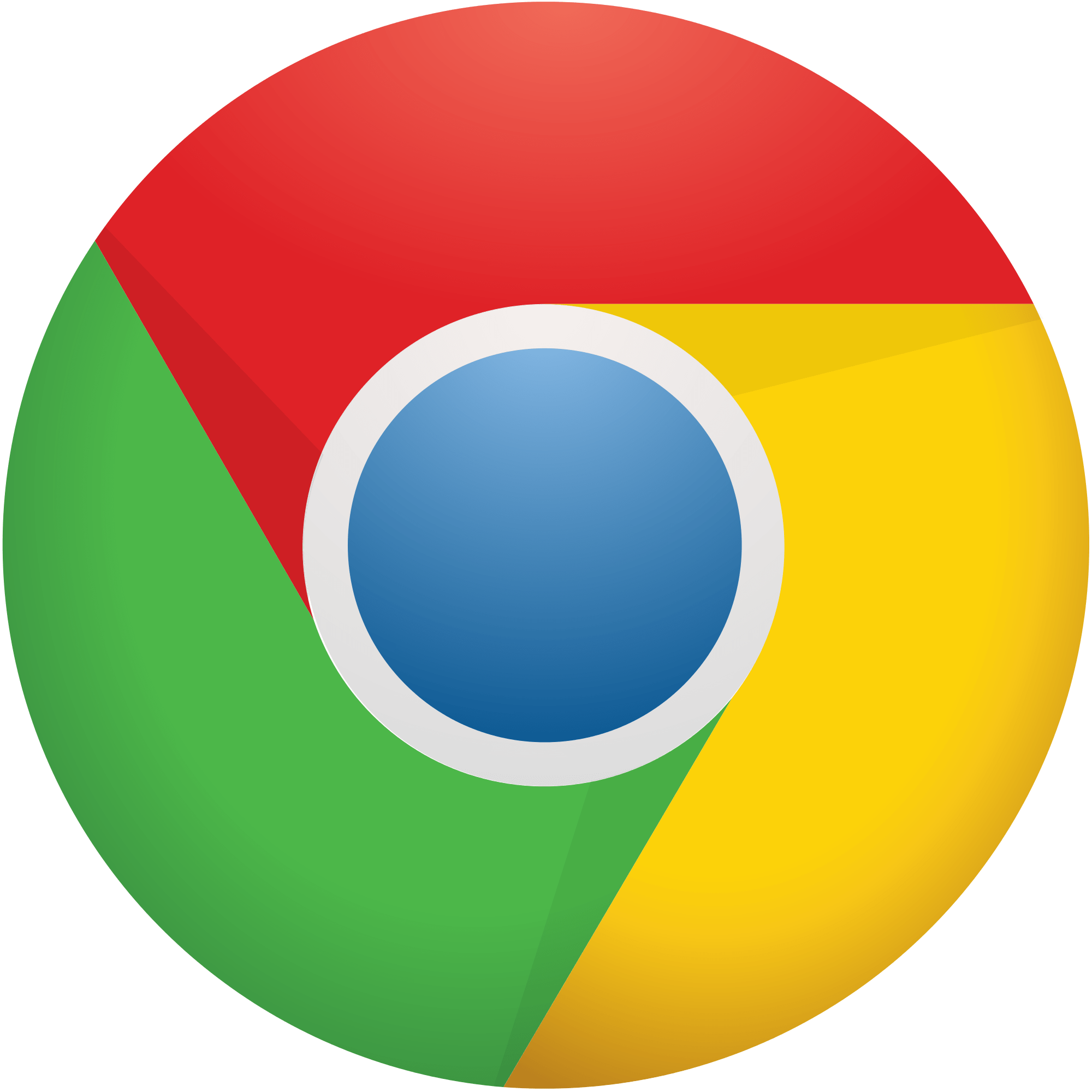
Chrome
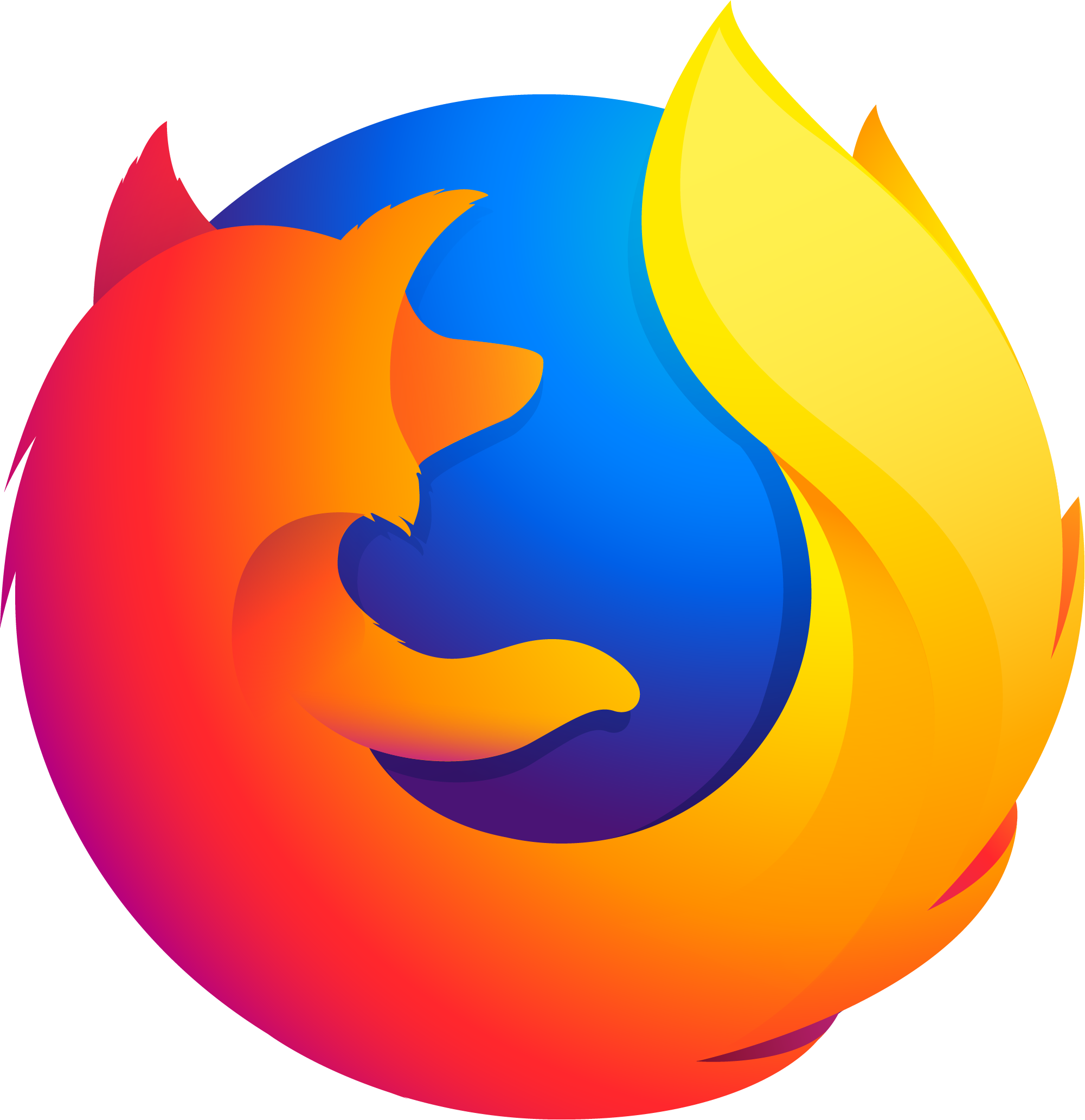
Firefox
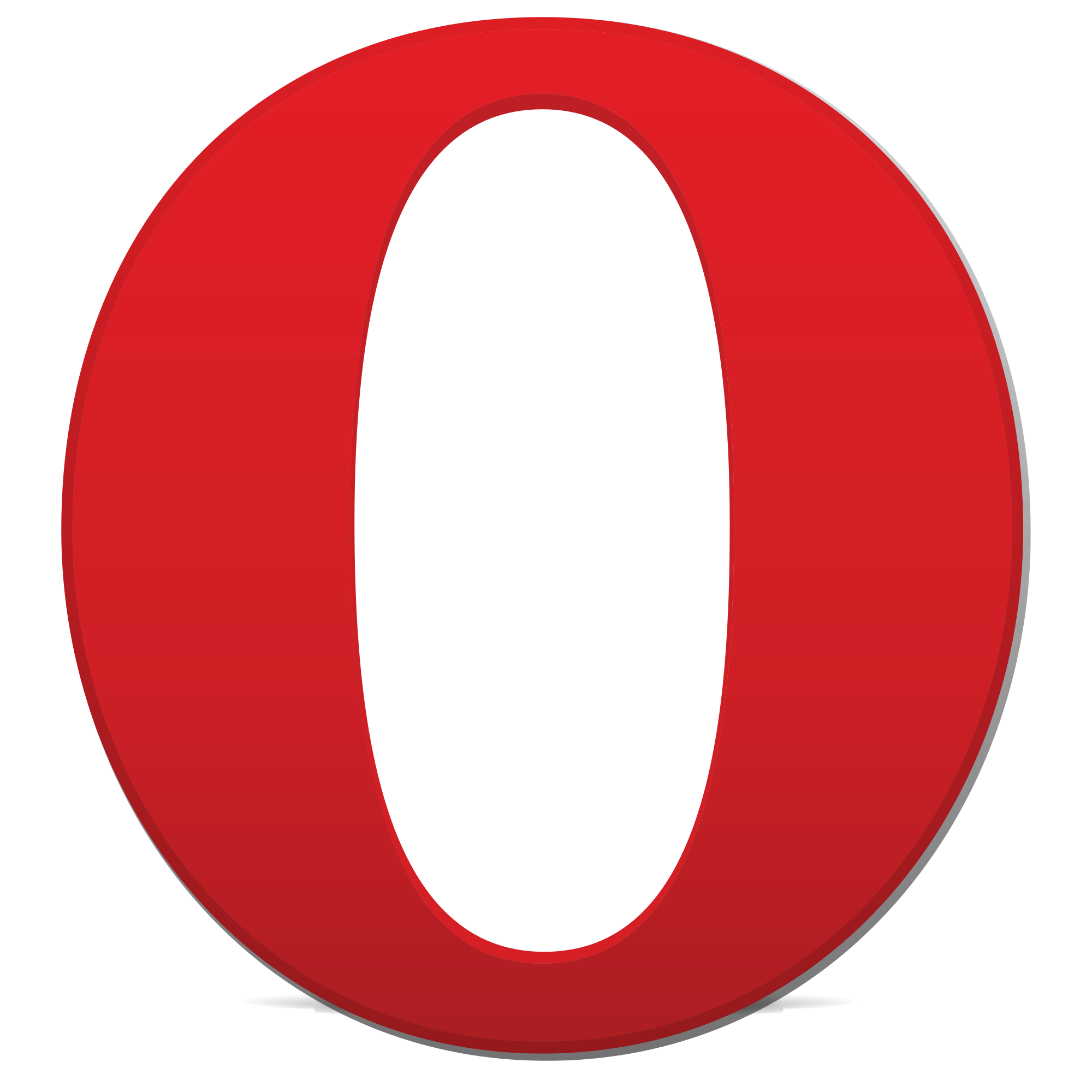
Opera
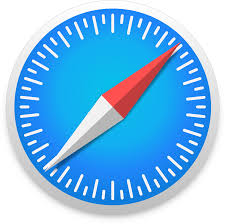