The JavaScript date functions manipulate time or retrieve information about it. This tutorial discusses the two categories of methods related to dates. Also, we explain how the JavaScript date object is created with the new Date() function. These date objects use a Unix Time Stamp.
You can always revisit our JavaScript date object and JavaScript date formats tutorials if needed. They're dedicated to JavaScript date object methods.
Contents
JavaScript Date: Main Tips
- Date methods make JavaScript get time or date, or set them.
- Date values can be expressed in years, months, days, hours, minutes, seconds or milliseconds.
- There are two categories of date methods:
get
methods andset
methods. get
methods return a part of a date.set
methods specify in what form the parts of the date will be returned.- By default, in computer languages, time is measured from January 1, 1970. That's called the Unix time.
get Methods
Date get
methods return a part of the date you need. Take a look at the table to get a better idea:
Method | Description |
---|---|
getDate() | Get the current day as a number (from 1 to 31) |
getFullYear() | Get the current year as a four digit number |
getMonth() | Get the current month (0-11, 0 is January) |
getDay() | Get the current weekday as a number (0-6, 0 is Sunday) |
getHours() | Get the current hour (0-23) |
getMilliseconds() | Get the current milliseconds (0-999) |
getSeconds() | Get the current seconds (0-59) |
getMinutes() | Get the curent minutes (0-59) |
getTime() | Get the time in milliseconds (since January 1st, 1970) |
getTime() Method
The method lets JavaScript get current time. It outputs the amount of milliseconds that have passed since January 1st, 1970:
var dt = new Date();
document.getElementById("dateOut").innerHTML = dt.getTime();
getFullYear() Method
The method lets JavaScript get current date year in a four digit number:
var dt = new Date();
document.getElementById("dateOut").innerHTML = dt.getFullYear();
getDay() Method
getDay()
returns the weekday as a number:
var dt = new Date();
document.getElementById("dateOut").innerHTML = dt.getDay();
Note: This method returns a number from 0 to 6 that indicates the current week day, 0 representing Sunday.
set Methods
You are familiar with the ways to get date JavaScript, but sometimes you need to set it. Let's review set
methods that help us set a part of the date:
Method | Description |
---|---|
setDate() | Set the current day as a number (from 1 to 31) |
setFullYear() | Set the current year (you can also set the day and month) |
setHours() | Set the current hour (from 0 to 23) |
setMilliseconds() | Set the current milliseconds (from 0 to 999) |
setMinutes() | Set the curent minutes (from 0 to 59) |
setMonth() | Set the current month (from 0 to 11, 0 is January) |
setSeconds() | Set the current seconds (from 0 to 59) |
setTime() | Set the time in milliseconds |
setFullYear() Method
The method sets the date to be a specified date. The example below sets the date to January 1st, 2018.
var dt = new Date();
dt.setFullYear(2018, 0, 1);
document.getElementById("dateOutput").innerHTML = dt;
Note: just as weekdays, months are counted starting from 0. January is 0, December is 11.
setDate() Method
The method sets which day of the month it is (1-31).
var dt = new Date();
dt.setDate(14);
document.getElementById("dateOut").innerHTML = dt;
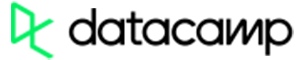
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
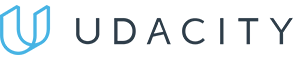
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
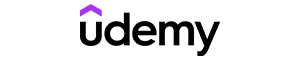
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Parsing Dates
There are different ways to make JavaScript get time or date. For example, Date.parse()
method lets you parse a properly written date from a string and convert it to milliseconds:
var milSec = Date.parse("November 14, 1995");
document.getElementById("dateOut").innerHTML = milSec;
You can perform a reverse action as well. Convert the milliseconds back and voila - you get the date JavaScript:
var milSec = Date.parse("November 14, 1995");
var dt = new Date(milSec);
document.getElementById("dateOut").innerHTML = dt;
JavaScript Compare Dates
You can easily make JavaScript compare dates. The example below compares the date of today with November 14th, 1995:
var today, goodDay, textOut;
today = new Date();
goodDay = new Date();
goodDay.setFullYear(1995, 10, 14);
if (goodDay > today) {
   textOut= "Today is before November 14, 1995.";
} else {
   textOut= "Today is after November 14, 1995.";
}
document.getElementById("dateOut").innerHTML = textOut;
UTC Date Methods
There is a time standard used in the whole world to regulate time and clocks. It's called the Coordinated Universal Time, abbreviated to UTC. Keep in mind daylight savings do not affect it in any way.
Naturally, UTC is also important in programming. In JavaScript, you can use special date methods to work with UTC:
Method | Description |
---|---|
getUTCDate() | Identical to getDate(), but returns the UTC date |
getUTCDay() | Identical to getDay(), but returns the UTC day |
getUTCFullYear() | Identical to getFullYear(), but returns the UTC year |
getUTCHours() | Identical to getHours(), but returns the UTC hour |
getUTCMilliseconds() | Identical to getMilliseconds(), but returns the UTC milliseconds |
getUTCMinutes() | Identical to getMinutes(), but returns the UTC minutes |
getUTCMonth() | Identical to getMonth(), but returns the UTC month |
getUTCSeconds() | Identical to getSeconds(), but returns the UTC seconds |
JavaScript Date: Summary
- You can use JavaScript date object methods to either set or get date values in years, months, days, hours, minutes, seconds or miliseconds.
get
methods return a part of a date.set
methods specify the format of the date to be returned.- You can make JavaScript get date according to Coordinated Universal Time (UTC).