Contents
setAttribute JavaScript: Main Tips
- This method is used to add a specified attribute to an element, giving the attribute a certain value.
- If the JavaScript attribute is already assigned to an element, the value is overwritten.
Using setAttribute
This setAttribute JavaScript method is used to add a specified attribute to an element, giving the attribute a certain value. Take a look at the example below. It shows how you can modify an input field to an input button:
document.getElementsByTagName("input")[0].setAttribute("type", "button");
If the attribute is already assigned to an element, the value is overwritten. If not, elements are assigned a new attribute with indicated values and name. Once you make JavaScript add attribute, the indicated name is set to lowercase letters only.
HTML elements define JavaScript properties that correspond to all standard HTML attributes. Therefore, when you try to set attributes that are not standard, you should use the JavaScript setAttribute function.
The following code example shows how to add an href attribute to an element:
document.getElementById("sampleAnchor").setAttribute("href", "https://www.BitDegree.org");
It is not advised to use this JavaScript attribute for styling. To change or add styles, you can access the Style object, which will effectively change the style, while the CSS stylesheet may override the style set by the HTML style attribute.
This example illustrates how not to do it.
element.setAttribute("style", "background-color: green;");
This next example is the correct way to do it:
Tip: using the removeAttribute() method will remove a specified JavaScript attribute from an element.
In the code snippet below, you may see how to check whether elements have a target attribute:
// Access the element with id="sampleAnchor" and assign its value to the variable elem
var elem = document.getElementById("sampleAnchor");
// if this element has a target attribute, its value will be set to "_self"
if (elem.hasAttribute("target")) {
elem.setAttribute("target", "_self");
}
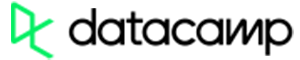
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
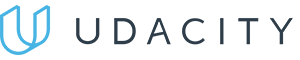
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
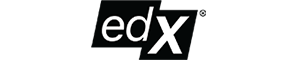
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
setAttribute Syntax
Look at the code example below. That's the standard way of writing JavaScript setAttribute function:
element.setAttribute(attributeName, attributeValue)
You can see two parameters need to be added to the parentheses. In this case, these arguments are not optional: they both must be included in the method.
Another important aspect is the possible errors you should avoid while making JavaScript add attributes. If you add characters that are not recognized by JavaScript, you will receive InvalidCharacterError
.
Parameter Values
There are two arguments to add to the setAttribute JavaScript function: name and value. Both of them are vital for the method to perform its task.
In the attributeName parameter, you are to include the name of the attribute you want to apply to an element. As we mentioned, it is automatically converted to lowercase letters.
The attributeValue should contain the value you want to assign to attributes. If the specified value is non-string, the setAttribute JavaScript function will turn it into a string.
Note: setAttribute JavaScript method does not produce a return value.