JavaScript handles data forms. Another topic to discuss is the JavaScript form validation, allowing to guarantee that specific type of data will be submitted.
This tutorial covers JavaScript methods and properties to validate user inputted data. The form validation JavaScript finds mistakes in the provided data. To increase validation requirements afterwards, read the JavaScript Regular Expressions tutorial to learn how to create your own validation patterns.
Contents
JavaScript Form Validation: Main Tips
- HTML form validation can be performed using JavaScript.
- JavaScript form validation checks the inputted information before sending it to the server.
- Usually, if the entered data is incorrect or missing, the server would need to send the request back to the user. But with JavaScript, validation proceeds on the client's computer.
Methods To Use
When using constraint validation, you can use two methods. Both of them will validate the data well, but the visitors of your website will notice the difference. Using setCustomValidity()
lets you add a customized message that the user will see upon inputting data.
Methods | Description |
---|---|
checkValidity() | Checks an input element and returns true if it contains valid data |
setCustomValidity() | Adds a validationMessage property to an input |
The example below displays a standard message if the inputted data is invalid using CheckValidity()
method:
function clickFunction() {
var inpObj = document.getElementById("out");
if (inpObj.checkValidity() == false) {
document.getElementById("test").innerHTML = inpObj.validationMessage;
}
}
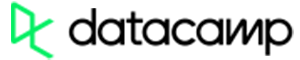
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
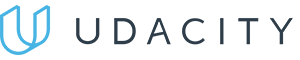
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
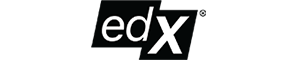
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Properties To Understand
There are three basic DOM properties used in constraint validation: validity, validationMessage, and willValidate. Let's see what each of them does:
Property | Description |
---|---|
validity | Holds values used for validating an input data |
validationMessage | Holds text which will be displayed when validation fails |
willValidate | Returns whether inputted data will be validated |
The values validity
holds are of a boolean type, meaning they can only return True or False. In the table below, you can see various properties it uses. Conditions in which they return True are listed on the right:
Property | Returns true if |
---|---|
customError | A custom message of validity is set |
patternMismatch | An element's pattern attribute does not match its value |
rangeOverflow | An element's value exceeds its attribute max |
rangeUnderflow | An element's value is lower than its attribute min |
stepMismatch | An element's value is incorrect per its attribute step |
tooLong | An element's value is longer than its attribute maxLength |
typeMismatch | An element's type is incorrect per its type attribute |
valueMissing | An element has no value |
valid | An element's value is correct and valid |
Validation Examples
The more examples you analyze, the better it sticks to your brain. We'll use a few more to illustrate the properties from the table we have above.
In this example, we will be using the rangeOverflow
property. A message will be displayed if the input is greater than 50. It will announce Value exceeds maximum:
function clickFunction() {
var text = "";
if (document.getElementById("out").validity.rangeOverflow) {
text = "Value exceeds maximum";
} else {
text = "Input OK";
}
document.getElementById("test").innerHTML = text;
}
For an opposite purpose, rangeUnderflow
property can be used. Take a look at the example below. If a user inputs a value that is less than 50, they will again see a message. This time, though, it will state Value too small:
function clickFunction() {
var text = "";
if (document.getElementById("out").validity.rangeUnderflow) {
text = "Value too small";
} else {
text = "Input OK";
}
document.getElementById("test").innerHTML = text;
}
JavaScript Form Validation: Summary
- You can use JavaScript to validate your HTML forms before sending.
- There are several methods you can use to check validity.
- In addition to methods, you can deploy multiple
properties
for JavaScript form validation.