TL;DR – JavaScript reduce()
function applies a specified callback (or a reducer) function on an array.
Contents
The use of JS reduce() made easy
There are some specific terms necessary for understanding reduce()
method. First of all, we have the accumulator: the value of the final result. Furthermore, reducer refers to the function that we apply to reach that final value.
For instance, if you have an array with multiple numeric values, you might want to get their sum. JavaScript reduce()
has an elegant way of implementing this action.
It uses the callback function with two parameters: accumulator and the currentValue. The value of the accumulator can be set to any value as the second parameter of the JS reduce()
function. The following code example shows the steps of getting the sum value from an array of elements:
const array1 = [55, 3, 76, 34];
document.getElementById("test").innerHTML = array1;
function myFunction() {
const reducer = (accumulator, currentValue) => accumulator + currentValue;
document.getElementById("test").innerHTML = array1.reduce(reducer);
}
A step-by-step explanation on how the code example above works:
- You create an array with values.
- The accumulator takes the first value. The initialValue becomes the second value.
- A callback function runs on every element in the array.
- You get one value.
Note: the value of the accumulator changes after every round.
If we want a specific accumulator, we need to define the initialValue. The next code example defines the initialValue as 10:
const array1 = [55, 3, 76, 34];
document.getElementById("test").innerHTML = array1;
function myFunction() {
const reducer = (accumulator, currentValue) => accumulator + currentValue;
document.getElementById("test").innerHTML = array1.reduce(reducer, 10);
}
As you can see, since the JavaScript array reduce function had an initial value, the sum of the elements increased.
Main syntax rules
The full syntax of JavaScript reduce()
is as follows. However, not all parameters need to be defined for the function to work.
array.reduce(callback(accumulator, currentValue[, index[, array]] )[, initialValue])
callback
: the function to be applied on every array element. It might not affect the first value if you define the initialValue.accumulator
depends on the supplied initialValue.currentValue
: the value being processed in the array.index
: the index of the currently processed element.array
: the array JSreduce()
is applied to.initialValue
: the value to use as the first argument when the callback function runs. If it is not defined, the callback function takes the first element and skips it.
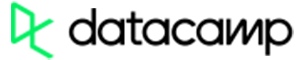
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
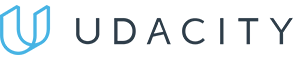
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
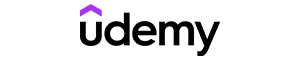
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Flattening arrays with reduce()
Flattening of arrays means that we transform a multidimensional array into a regular one-dimensional collection. You can use the flat()
function for this as well, but it is always beneficial to have more options. JavaScript reduce()
is one of the candidates.
Here we have a multidimensional array:
var myArray = [[5, 7], [2, 5, 6], [9, 5, 3, 7]];
We want it to become like this:
[5; 7; 2; 5; 6; 9; 5; 3; 7]
In the following example, we turn the multidimensional array into one-dimensional:
var myArray = [[5, 7], [2, 5, 6], [9, 5, 3, 7]];
document.getElementById("test").innerHTML = myArray.map(e => e).join("; ");
function myFunction() {
var myNewArray = myArray.reduce(function (prev, curr) {
return prev.concat(curr);
});
document.getElementById("test").innerHTML = myNewArray.map(e => e).join("; ");
}