Contents
JavaScript Style Display: Main Tips
- This property is used to set or return the display type of a specified element.
- In case you are dealing with a block element, you can also use the float property to change its display type.
style.display Explained
The JavaScript style display property is meant for setting and returning the display type of a specified element.
Most HTML elements have the inline or block display types. The content that inline elements feature floats on their left and right sides. Block elements are different because they do not show content on their sides: they fill the entire line. Furthermore, the JavaScript style display property can be used to complete the JavaScript hide element (as well as show element).
You can notice that a similar function can be achieved with the visibility property. However, they have their differences: when the display property is set to none
, it will conceal the whole element (it will no longer take any space). When the visibility property is set to hidden
, the element will be displayed in its rightful place (only its content will be concealed).
Of course, there's no choosing sides here. There are different situations, and while one property might seem best in one case, another will prove to be more useful in another.
The example below shows how JavaScript style display property can make an element invisible:
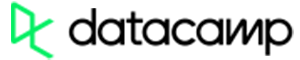
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
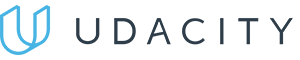
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
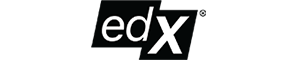
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Proper Syntax
If you need to find out the display type for a certain object, you can follow the example below to return the display property:
object.style.display
If you want to set the display property yourself, you will need to use this example for reference:
object.style.display = value
To use the JavaScript style display property to the max, you should learn all of the possible values. There are different types of them, fit for different situations. We have already discussed the none
value which is used to hide elements. The table below briefly reviews various other values to use:
Value | Description |
---|---|
block | Renders as block-level element. |
compact | Renders as inline or block-level element. Depends on context. |
flex | Renders as block-level flex box. New with CSS3. |
inherit | The value of the display property is inherited from parent element. |
inline | Renders as inline element. Default. |
inline-block | Renders as block box inside inline box. |
inline-flex | Renders as inline-level flex box. New with CSS3. |
inline-table | Renders as inline table (like <table>), without line break before or after table. |
list-item | Renders as list. |
marker | Content is set before or after box to be marker (can be used along with :before and :after pseudo-elements. Otherwise identical to inline). |
none | Element is not displayed in any way (but remains in the DOM). |
run-in | Renders as block-level or inline element. Depends on context. |
table | Renders as block table (similar to <table>) with a line break before and after the table. |
table-caption | Renders as table caption (similar to <caption>). |
table-cell | Renders as table cell (similar to <td> and <th>). |
table-column | Renders as column of cells (similar to <col>). |
table‑column‑group | Renders as group of one or more columns (similar to <colgroup>). |
table-footer-group | Renders as table footer row (similar to <tfoot>). |
table-header-group | Renders as table header row (similar to <thead>). |
table-row | Renders as table row (similar to <tr>). |
table-row-group | Renders as group of one or more rows (similar to <tbody>). |
initial | Set property to default. |
inherit | Inherit property from its parent. |
Return Value
As most JavaScript functions, the method used for style display also has a return value. It shows the display type of a specific element.
You are now familiar with all the display types. Therefore, it will be easy for you to identify the return value you get when trying out the function yourself. Keep in mind that by default the JavaScript style display property will be set to inline
.
Default Value: | inline |
---|---|
Return Value: | A string, which represents the display type of a specified element |
CSS Version | CSS1 |
Useful Code Examples
To make the process of learning JavaScript style display easier, we shall introduce you to some code examples, illustrating how different values work.
The following example presents how the none
value should be written in comparison to the visibility property (which is set to hidden
):
function sampleDisplay() {
document.getElementById("samplePara1").style.display = "none";
}
function sampleVisibility() {
document.getElementById("samplePara2").style.visibility = "hidden";
}
Now, this next example demonstrates how to toggle between showing and hiding elements:
function displayFunction() {
var sampleDiv = document.getElementById('sampleDiv');
if (sampleDiv.style.display === 'none') {
sampleDiv.style.display = 'block';
}
else {
sampleDiv.style.display = 'none';
}
The code below shows how three specific values are different from one another, specifically inline
, block
and none
:
function displayFunction(sampleDiv) {
var selectedWhich = sampleDiv.selectedIndex;
var sel = sampleDiv.options[selectedWhich].text;
var elem = document.getElementById("sampleSpan");
elem.style.display = sel;
}
With the following example, it is possible to retrieve display type of <p>
: