AJAX form submitting allows you to send data in the background, eliminating the need to reload websites to see the updates. This makes the user experience much smoother.
By letting the browser and server exchange information without disturbing other activities, it saves both time and resources you would otherwise waste constantly reloading your site.
Contents
AJAX Form: Main Tips
- AJAX stands for Asynchronous JavaScript and XML.
- By sending asynchronous requests, it allows an application to refresh a part of a page without having to reload the whole document.
- Using AJAX, forms can be made more functional and dynamic.
Form With Options to Select
First of all, we create a simple input form using HTML:
<form>
<select name="users" onchange="showUser(this.value)">
<option value="">Select a person:</option>
<option value="johny">Johny Dawkins</option>
<option value="margaret">Margaret Johnson</option>
<option value="julie">Julie Doodley</option>
</select>
</form><br>
<div id="txt_hint">
<strong>This is where the information will appear</strong>
</div>
In it, the user can choose one of three possible options from a dropdown menu. When they do, an onchange
event is triggered.
We also create a div
element as an area for the additional information. However, that functionality will not work yet. To enable it, we have to call the showUser()
function:
function showUser(str) {
if (str == ""){
document.getElementById("txt_hint").innerHTML = "";
return;
} else {
if (window.XMLHttpRequest) {
// script for IE 7+ and other popular browsers
xmlhttp = new XMLHttpRequest();
} else {
// script for IE 5 and 6
xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");
}
// check if server response is ready
xmlhttp.onreadystatechange = function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
// get the element in which we will display our suggestions
document.getElementById("txt_hint").innerHTML = xmlhttp.responseText;}
};
xmlhttp.open("GET","getuser.php?q="+str, true);
xmlhttp.send();
}
}
To enable our AJAX form, we create an XMLHttpRequest
. The triggered onchange
event will call the txt_hint
function. The additional information about the person you chose will then be displayed below the input area.
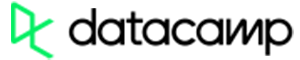
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
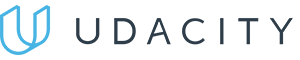
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
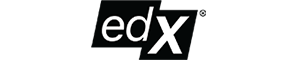
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Text Input AJAX form
In our next example, we will create a form with a text field:
<body>
<p><strong>Try typing a name into the below input field:</strong></p>
<form>
First name: <input type="text" onkeyup="showHint(this.value)">
</form>
<p>Possible suggestions: <span id="txt_hint"></span></p>
</body>
The form will recognize the same values we had in our first example. However, for it to show additional information, we will have to call showHint()
function:
function showHint(str) {
//check if input field is empty
if (str.length == 0) {
document.getElementById("txt_hint").innerHTML = "";
return;
} else {
//if the field is not empty, create an XMLHttpRequest to get suggestions
var xmlhttp = new XMLHttpRequest();
// server response is ready, we call the function
xmlhttp.onreadystatechange = function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
document.getElementById("txt_hint").innerHTML = xmlhttp.responseText;
}
};
xmlhttp.open("GET", "/learn/best-code-editor-example?q=" + str, true);
xmlhttp.send();
}
}
By checking the str.length
variable, the form identifies whether the field is empty or not. If it is, we discard the txt_hint
placeholder and exit the function.
If the input text field has something in it, we can make an XMLHttpRequest object, and set up the function to be ready to execute once the server response is prepared.
Note: the str variable is holding the content of our input text field.
AJAX Form: Summary
- AJAX sends asynchronous requests that help browser and server exchange information. Thus, you can see updates without reloading the page.
- It saves you time and simplifies user experience, as there is no need to refresh pages constantly.
- With AJAX, form submittion is smoother and more user-friendly.