When writing JavaScript code, there are various problems you may run into. One of the things that can help you avoid bugs is JavaScript strict mode that you can turn on using JavaScript use strict directive.
Writing JavaScript in strict mode allows you to avoid possible bugs that you may run into if you accidentally deviate from the correct JavaScript syntax. Strict mode is also useful in cases of typos, which can result in unnecessary new variables.
Contents
JavaScript Use Strict: Main Tips
- By using JS
use strict
directive, you set JavaScript code to be executed in strict mode. - This directive will be recognized depending on where it was written. It can be the whole document or a particular function.
What is JS Use Strict?
This directive was newly introduced in ECMAScript5. Thus, it is ignored by earlier JavaScript versions. It is a literal expression, not a statement.
The purpose of the use strict
JavaScript directive is to make the code execute in strict mode. This mode sets up certain conditions for the execution of code to make it cleaner. For example, with strict mode, you cannot use undeclared variables.
Keep in mind that strict mode isn't universally supported. These are the first versions of the major browsers that support this directive (the later ones support it, too):
- IE version 10
- Firefox version 4
- Chrome version 13
- Safari version 6
- Opera version 12.1
Strict Syntax
Take a look at how your should enter the strict mode in JavaScript:
"use strict";
The syntax used for declaring strict mode is compatible with older JavaScript versions. However, it doesn't work in them. For example, when compiling number literals (1 + 2;
) or string literals ("John Johnson";
) JavaScript will not run into any problems. It will only compile to a variable that does not exist and die.
So, JS use strict
directive will only be read by the new compilers correctly. The older ones simply won't recognize it.
Note: make sure not to forget quotation marks and the semicolon at the end: "use strict"; .
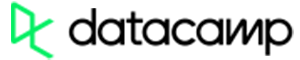
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
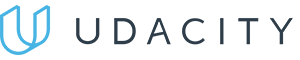
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
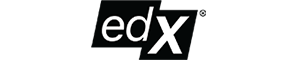
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Reasons to Use
This way of writing JavaScript may prove to be more secure than usual. It turns certain cases of bad syntax into actual errors, making your code cleaner and less likely to contain bugs. For example, if you mistype a variable name in JavaScript, it will create a new global variable. When using strict mode, an error will be thrown, making it impossible to create a new global variable by accident.
In JavaScript, developers usually do not receive any error feedback when assigning values to non-writable properties. In strict mode, you cannot assign anything to non-writable properties, get-only properties, non-existing properties, variables, or objects, for an error will be thrown straightaway.
Declaring Strict Mode
You can declare strict mode by using the JS use strict
directive at the beginning of a file or a function definition.
If declared at the beginning of a file, all of the code will execute in strict mode:
"use strict";
sampleFunction();
function sampleFunction() {
b = 2+2;
}
If declared at the beginning of a function definition, a function form of use strict
will be generated. It has a local scope, only applying to the block of code describing the function, and not outside of it:
a = 2 + 2;
sampleFunction();
function sampleFunction() {
"use strict";
b = 2 + 2;
}
What's Not Allowed
Let's go throught the list of what you cannot do when JavaScript is in strict mode. First of all, you cannot use undeclared variables:
You cannot use undeclared objects, too:
Variables, objects, or functions cannot be deleted using the delete
keyword:
You cannot duplicate a parameter name:
You cannot use octal numeric literals:
Escape characters are not allowed too:
Writing to a read-only or get-only property is not allowed:
"use strict";
var objec = {};
Object.defineProperty(objec, "a", { value: 1, writable: false });
objec.a = 2;
"use strict";
var objec = {get a() { return 0 } };
objec.a = 2;
You cannot delete undeletable properties:
You cannot use strings "eval"
or "arguments"
as variables:
You cannot use with
statement:
For security reasons, you cannot use eval()
for creating variables inside the scope it was called from. In a function call like f()
, the value of this was the global object. In strict mode, it is undefined
:
Future Proof Keywords
You may remember some words cannot be used to call new functions or variables. They are called reserved words. Usually, they are unavailable because they are used for inbuilt functions or other language elements already.
Now, some keywords are reserved for future JavaScript versions. Thus, they cannot be used in strict mode as well. Let's take a look at the list:
- interface
- implements
- package
- let
- protected
- private
- static
- yield
- public
JavaScript Use Strict: Summary
- The
use strict
directive executes JavaScript code in strict mode. - You have to be aware of where you place the
use strict
JavaScript directive, since it will give different results. For example, placing it within a function will create the function form ofuse strict
. - There are specific rules you must follow when writing code in strict mode.