Contents
JavaScript subString: Main Tips
- The JavaScript
string.substring()
method extracts the symbols between two indexes and returns a data string. - This method extracts the symbols in a string between end and start, not including end itself.
- If the end index has a smaller value than start, the arguments will be switched.
- If either end or start is defined as negative number, the value is 0.
- The original string is left unchanged after using this method.
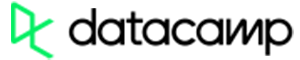
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
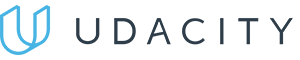
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
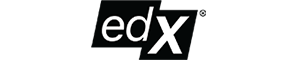
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
substring() Explained
The JavaScript substring()
method retrieves a specific portion of a string. During this extraction process, the original element is not modified. Instead, the subString
JavaScript will return a brand new string, containing the characters taken from the original string.
The usage of JS substring()
can vary since it's possible to indicate different indexes to start and end extraction.
In this example, extraction begins from the fourth symbol:
function learnFunction() {
var string = "Whats your name?";
var result = string.substring(3);
document.getElementById("learn").innerHTML = result;
}
Here we can see how the values are swapped when the end value turns out to be smaller than start value:
function learnFunction() {
var string = "Whats your name?";
var result = string.substring(5, 1);
document.getElementById("learn").innerHTML = result;
}
In this example, the start value is less than 0 (negative). Therefore, the command will start from index number 0:
function learnFunction() {
var string = "Whats your name?";
var result = string.substring(-4);
document.getElementById("learn").innerHTML = result;
}
Let's see how only the first symbol can be extracted:
function learnFunction() {
var string = "Whats your name?";
var result = string.substring(0, 1);
document.getElementById("learn").innerHTML = result;
}
In this example, only the last symbol is extracted:
function learnFunction() {
var string = "Whats your name?";
var result = string.substring(15, 16);
document.getElementById("learn").innerHTML = result;
}
You might notice that the JavaScript substring()
function is similar to the substr()
method. Read about their differences and the substr()
function in this tutorial.
The JavaScript string.substring()
function can start the extraction of a string from the first character. Then, the start index should be set to 0.
Note: in case the start index is indicated as a negative number, the function will automatically render it as 0.
In the following example, the start index is set as 1 (the second character), while the end index is set to 4 (the fifth character).
Open the code editor by clicking the Try it Live button. You will see that the returned string is hat
. The fifth character s
is not included as the end index is never extracted by the subString
JavaScript function:
function learnFunction() {
var string = "Whats your name?";
var result = string.substring(1, 4);
document.getElementById("learn").innerHTML = result;
}
JavaScript substring(): Syntax
Follow this syntax for the JavaScript substring()
function:
string.substring(start, end)
start
indicates from which index should the characters be extracted. It is a required parameter of thesubString
JavaScript.end
specifies when the extraction process should conclude. If omitted, the function will automatically extract the rest of the string. Optional.
Remember: the JavaScript character index starts at 0, not 1.