Each of the JavaScript data types has their own functionality, properties, and methods you need to be aware of. Differentiating each one is fundamental when starting out with JavaScript.
JavaScript strings store textual data. In this tutorial, you will learn all about JavaScript string methods and properties. You will understand how to manipulate textual data using them, how to make JavaScript concatenate strings, cut, append or prepend text, as well as get certain characters from a string.
Contents
JavaScript String Methods: Main Tips
- String methods assist in using and modifying strings.
- There is a large variety of string methods in JavaScript.
- You must change the string to an array before accessing it as an array using array methods.
Searches in a String
There are often cases when you need to perform a specific search in your JavaScript string. Whether you need to look for an index or a string, there are functions that allow you to do just that. Let's take a look at their descriptions and examples.
indexOf() is used to bring back an index of the first instance of a set character or text in the text string:
var loc = "Lets find where 'place' occurs!";
var plc = loc.indexOf("place");
Now, lastIndexOf()
is used to bring back an index of the last instance of a set character or text in the text string:
var plc = "Please locate where 'place' occurs!";
var loc = plc.lastIndexOf("place");
The two methods bring back -1 when no text is found. We already know that JavaScript starts calculating from 0. Therefore, the methods may take in an additional parameter as the starting index to start the inquiry.
To find the starting index of a defined value, search()
is used:
var txt = "Let us find where 'place' starts!";
var loc = txt.search("place");
Note: search() and indexOf() may appear the same, but indexOf() does not accept regular expressions, while search() takes only one start position argument.
Extracting String Parts
The methods listed below are used for extracting a piece of a string:
slice(start, end)
substring(start, end)
substr(start, length)
JavaScript slice() is used to extract a piece of string and return it as a new string. There are two parameters: a start position index and an end one. In the code below, you can see a part of a string being sliced from 7th to 13th position:
When there is a negative value defined, the calculation is started from the end string location. In the code below, you can see a part of a string being sliced from -12th to -6th position:
This method slices the other part of a string if the second parameter is excluded:
It can be counted starting from the end:
Note: IE 8 does not support negative values.
The JavaScript substring() method is different in one major way: it will not receive negative value indexes:
var txt = "BMW, Audi, Mercedes";
var ans = txt.substring(7, 13);
Now, the substr() method has its own difference as well. The length
is defined in the second value parameter, thus, it can't be negative.=:
Speaking of the length of a JavaScript data string, you can use the length
property to bring it back. See the example below:
Extracting Characters
There are also two methods that you can use when you need to take any characters of a string safely:
charAt(position)
charCodeAt(position)
We use charAt JavaScript method to return a character at a specified index in text. We type the function name as charAt()
:
charCodeAt()
is used to return a unicode of a character at a defined index location in a string:
Replacing String Content
Using replace()
changes the defined value to another value:
txt = "I like to learn with bitdegree!";
var x = txt.replace("bitdegree", "bitdegree.org");
By default, the replace()
function replaces only the first match:
txt = "I like to learn with bitdegree. I like to learn with bitdegree.";
var x = txt.replace("bitdegree", "bitdegree.org");
The /g
is used to switch out all matched up strings:
txt = "I like to learn. I like to learn with bitdegree.";
var x = txt.replace(/bitdegree/g, "bitdegree.org");
The replace()
is case-sensitive so using capital letters will not make it work:
txt = "Lets visit bitdegree!";
var x = txt.replace("BITDEGREE", "bitdegree.org");
/i
is used to solve this problem:
txt = "Let's visit bitdegree!";
var x = txt.replace(/bitdegree/i, "bitdegree.org");
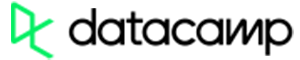
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
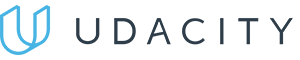
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
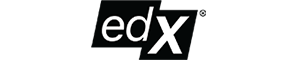
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Converting Cases
In some cases, you might need to quickly convert the whole content of the string to a single case. For example, you might wish to accent something by putting it in all caps. There are functions in JavaScript designed to perform just that!
toUpperCase()
method converts the text into uppercase:
var txt1 = "Hi World!"; // String
var txt2 = txt1.toUpperCase(); // txt2 is txt1 converted to upper
toLowerCase()
method converts the text into lowercase:
var txt1 = "Hi World!"; // String
var txt2 = txt1.toLowerCase(); // txt2 is txt1 converted to lower
Joining Strings
Sometimes we need to join separate strings into one. This method is called JavaScript string concatenation, and it can be executed by using the conCat JavaScript function:
var txt1 = "Hi";
var txt2 = "World";
var txt3 = txt1.concat(" ", txt2);
Besides being a JavaScript string concatenation method, concat()
is sometimes used as a plus operator. Both lines match in functionality:
var txt = "hi" + " " + "World!";
var txt = "hi".concat(" ", "World!");
Both of these methods will be returning a unique string. The primary string is not changed. That being said, strings can only be swapped out.
Converting a String to an Array
Sometimes to achieve the results we need we have to change the data type. JavaScript split() function is used to transform string to array JavaScript will use. When excluding the separator, the array will then store the full string in [0]:
var str = "x,y,z,g,t"; // String
str.split(","); // Split on commas
str.split(" "); // Split on spaces
str.split("|"); // Split on pipe
If we use an empty string as a separator (""
), the array is going to consist of single letter characters:
var str = "Hello"; // String
str.split(""); // Split in characters
JavaScript String Methods: Summary
- There are various string methods you can use to manipulate strings.
- You can use string methods to JavaScript concatenate strings, split them, get indexes of characters and perform many other tasks.
- You can also turn string to array JavaScript will recognize.