Contents
JavaScript Boolean: Main Tips
- There are two values of JavaScript boolean:
true
orfalse
. - Booleans check whether an expression is true or not.
Values Explained
Developers use boolean data type when they want to manage expressions, variables, and objects.
The JavaScript boolean function can be used to find out whether a specified expression is true:
The JavaScript boolean has two values: true and false. As a common practice, developers use the boolean type together with if else .
There's also an even simpler way of applying JavaScript boolean operators. As booleans have logical values, we might use logical operators:
You can apply JavaScript boolean to whole string statements to determine whether they are true or false. The results of such application will be linked to the next action a program will perform (for instance, when if
statement is used).
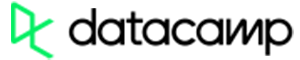
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
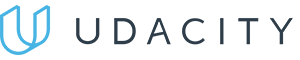
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
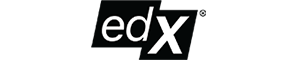
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Converting String to Boolean
In some cases, developers need to convert JavaScript string to boolean when the boolean value is in a string format.
Note: the bad news is that JavaScript does not offer an built-in function for such an action. You have to declare one.
<!-- Code for converting strings to the boolean value by using the == operator -->
<script>
function convertToBoolean() {
var input = document.getElementById("input");
var x = document.getElementById("div");
var str = input.value;
x.innerHTML = str == 'coding';
}
In the example above, we are creating an input field. According to the text users type in, you make JavaScript return Boolean false
or true
values. To get true
, you have to type in the word coding in the input field.
Properties
There are several boolean JavaScript properties you should be familiar with. The constructor
indicates the method applied when the boolean prototype was created.
The second property called prototype
allows developers to enhance boolean prototypes with additional methods and properties.
Property | Description |
---|---|
constructor | Return function used to create JavaScript boolean prototype. |
prototype | Allow adding properties and methods to Boolean prototype. |
Methods to Apply
The toString()
function does not make JavaScript convert string to boolean. Instead, this method converts booleans to strings.
Additionally, you can retrieve the primitive value of boolean using the valueOf()
method.
Method | Description |
---|---|
toString() | Convert boolean value to a string, and return the result. |
valueOf() | Return the primitive value of boolean. |