Contents
JavaScript replace: Main Tips
- The JavaScript string
replace()
method searches a string for a regular expression or a specified value and returns a string with replaced specified values. - Use the global
g
modifier to replace all value occurrences. - The original string is not modified by using this method.
replace() Explained
JavaScript replace()
function is designed to detect specified keywords and replace them with other strings. Besides the fact that you can replace strings with strings, you can also indicate functions that are to be called for the detected matches.
By default, the JavaScript replace()
method will find and modify the first identified match. If you wish to replace all specific patterns with new ones, you should apply the g
switch. It is supposed to be added to the regular expression parameter which determines the new pattern for a string.
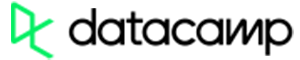
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
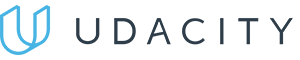
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
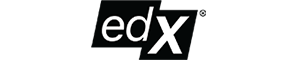
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Correct Syntax
In the snippet below, you can see the basic syntax for string replace JavaScript function:
string.replace(searchvalue, newvalue)
The function includes the string which is going to be affected by the string replace JavaScript function. The method returns the said string with certain replacements. In the following example, we replace bitdegree.org
with Doggo
:
var string = "This is bitdegree.org!";
var result = string.replace("bitdegree.org", "Doggo");
The JavaScript replace()
function contains two parameters and both of them are required. The first one can be called the searchvalue
, as it indicates what the method is supposed to replace. The name of the second argument - newvalue
- is quite self-explanatory: it specifies what the string will be replaced with.
Parameter | Description |
---|---|
searchvalue | Needed. The regular expression or value that will be replaced. |
newvalue | Needed. The regular expression or value to replace the original. |
Code Examples for Practice
You are welcome to practice using the JavaScript replace()
function right from your browser. Our code editor will open after you click the Try it Live button.
In this first example, we complete a global replacement:
function learnFunction() {
var string = document.getElementById("learn").innerHTML;
var result = string.replace(/white/g, "black");
document.getElementById("learn").innerHTML = result;
}
The code snippet below will show you how to complete a case-insensitive global replacement:
function learnFunction() {
var string = document.getElementById("learn").innerHTML;
var result = string.replace(/white/gi, "black");
document.getElementById("learn").innerHTML = result;
}
Now, in our last example, we will be using a function for returning the replaced text:
function learnFunction() {
var string = document.getElementById("learn").innerHTML;
var result = string.replace(/white|bed|table/gi, function myFunction(z) {
return z.toUpperCase();
});
document.getElementById("learn").innerHTML = result;
}