Contents
JavaScript Split String: Main Tips
- JavaScript
string.split()
method splits a data string into a substrings array and returns the new array. - You can specify where the string will be split by choosing a separator.
- It's also possible to limit the number of substrings to return.
Using string.split()
The JavaScript split string function transforms a specified string into an array of substrings. It does not affect the original string: instead, it generates new ones.
The syntax for JS split string accepts two parameters:
string.split(separator, limit)
Both of them will be explained with examples in the following section.
var string = "What's your name?";
var result = string.split(" ");
In the JavaScript split string example above, the function returns an array of three substrings. You can also see you need to first specify the string that you wish to divide.
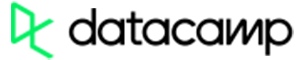
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
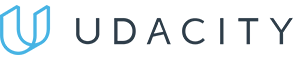
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
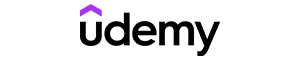
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Splitting String with a Separator
The separator
sets a string which determines the points the original string should be divided in. This parameter is not required. If you skip it, the function will return a single element. The same will happen if no matches for the separator are found.
In the example below, you can see a case when the separator is omitted:
function learnFunction() {
var string = "What's your name?";
var result = string.split();
document.getElementById("learn").innerHTML = result;
}
Now here we choose a single letter (a
) as our separator:
function learnFunction() {
var string = "What's your name?";
var result = string.split("a");
document.getElementById("learn").innerHTML = result;
}
Now in this example, an empty string is chosen as the separator (""
). Thus, every UTF-16 code unit is separated:
function learnFunction() {
var string = "What's your name?";
var result = string.split("");
document.getElementById("learn").innerHTML = result;
}
Limiting Substring Quantity
limit
is also an optional parameter. However, if it is included in the parentheses of JS split string function, the number of splits will be fixed. Thus, the returned array will consist of a specific number of strings.
In the JavaScript split string example below, the result array is limited to two values:
function learnFunction() {
var string = "What's your name?";
var res = string.split(" ", 2);
document.getElementById("learn").innerHTML = res;
}
Note: even if the limit is set, the function might deliver a shorter list if the original string contains fewer matches.