JavaScript is heavily reliant on objects. The most used type of data in JavaScript is considered to be the object. JavaScript objects have properties and methods.
In this tutorial, you'll learn all about objects JavaScript holds, creating and handling them. Correct syntax of writing a JavaScript object will also be covered. You will understand JavaScript object properties and methods, defining and using them.
Contents
JavaScript Objects: Main Tips
- JavaScript, just like many other computer languages, is based on objects.
- To better understand the concept, JavaScript objects can be compared to real life objects.
- JavaScript object is an independent entity. It has its methods and properties.
- A property's value may be a function, in which case the property is known as a method.
- Do not declare numbers, strings, and booleans as objects.
- JavaScript object properties can be accessed in two ways:
human.firstName
andhuman["firstName"]
.
Real Life Example
In real life, a bike is an object. A bike has properties like color and height, and methods like drive and break:
Object | Properties | Methods |
![]() |
bike.name = mountain300; bike.weight = 15kg; bike.color = black; |
bike.drive() bike.break() |
All bikes have the same properties, but their values are different for each bike. Also, all bikes have the same methods, but they can be executed at different times.
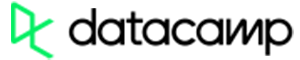
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
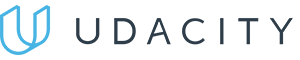
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
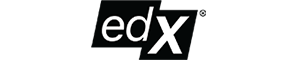
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Objects in Variables
Variables in JavaScript are used for storing data. A JavaScript object is data signed to a variable. In the editor example below, you can see that the value mountain300
is assigned to a variable bike
. The value mountain300
instantly becomes a JavaScript object:
JavaScript objects are also variables, but they can have more than one value assigned. The example below assigns multiple values to the bike
variable: mountain300
, 15
, black
.
To write multiple values, you need to use the right syntax. The values are assigned to names, and name: value
combinations are formed:
var bike = {
title: "mountain300",
weight: "15",
color: "black"
};
Note: the values a JavaScript object contains are called properties.
Methods You Might Use
Methods represent actions which can be performed on JavaScript objects. Just like you've seen in the example with a bike before, a bike can be driven and stopped using brakes. In this case, drive and brake are the methods that can be used on the bike
object.
Methods are stored in properties as function definitions:
Property | Property Value |
---|---|
title | mountain300 |
weight | 15 |
price | 210 |
color | black |
info | function() {return this.title + " " + this.price;} |
You already know how to access JavaScript objects' properties, so you may have a general idea of how the object methods can be accessed. The JavaScript object syntax is a bit different but relatively simple.
In case you access the fullName
property without the parentheses ()
, the function definition will be returned instead of the value:
JavaScript Objects: Summary
- JavaScript objects have properties and methods.
- JavaScript object properties are assigned as
name: value
pairs. - javaScript object method is a property that is a function.