Whenever you create a new website, you want to capture the attention of your users, keep them engaged, and provide them with a great experience. A great way to do that is making the code you write in JavaScript animate your pages.
JavaScript animations can be of various kinds - a content box sliding in upon page load, some hilarious text animation, anything you can imagine. However, overstacking your website with JS animations might make it look tacky.
In this tutorial, you will learn to create a simple JavaScript DOM animation. We will also go through aspects of HTML and CSS to explain how to set up a proper layout.
Contents
JavaScript Animation: Main Tips
- To create an HTML animation JavaScript can be used.
- Container elements must have a
relative
position, and JS animation elements must have anabsolute
position.
Code Examples
Let's write our initial code for what will eventually be a JavaScript animation.
<strong>An animation of JavaScript</strong>
<div id="animate">The location of the animation</div>
Now, we must have some place to put our creation in. Using the code below, we will position it within a Javascript animation container:
<div id="contain">
<div id="animation">The location of the animation</div>
</div>
Let's now set the container element to position: relative
and the animation JavaScript element to position: absolute
:
#container {
width: 500px;
height: 500px;
position: relative;
background: green;
}
#animate {
width: 60px;
height: 60px;
position: absolute;
background: red;
}
An element's style is changed by programming movement step by step. A timer calls out those changes. Continuous JavaScript animations are achieved by setting a tiny timer interval using setInterval
:
var id = setInterval(frame, 6);
function frame() {
if (/* test */) {
clearInterval(id);
} else {
/* element style changes */
}
}
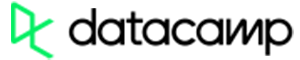
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
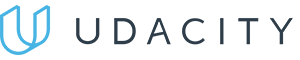
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
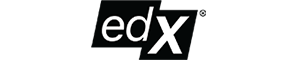
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Create JS Animations
We have covered every step, and you should have grasped the basic ideas by now. Of course, to strengthen this new knowledge, you should try to put it to practice. Use the code snippets provided, get inspired, and create your first JavaScript animation examples on your own:
function myMove() {
var el = document.getElementById("animate");
var loc = 0;
var ids = setInterval(frame, 6);
function frame() {
if (loc == 350) {
clearInterval(ids);
} else {
loc++;
el.style.top = loc + 'px';
el.style.left = loc + 'px';
}
}
}
JavaScript Animation: Summary
- You can use JavaScript to create HTML animations.
- It is important to pay attention to and set the right value to the
position
property. - You can use
style
to style the elements of your JavaScript animations in any way you like.