Contents
JavaScript Number to String: Main Tips
- The JavaScript number to string method can convert a number to a string.
- It is the opposite of
parseInt()
function used to convert string to number JavaScript. - All browsers support it.
- The function returns a string which represents a number.
Purpose and Usage
The toString()
function is used to convert any JavaScript number to string. It is one of the basic techniques that every programmer needs to learn if they wish to handle numbers properly.
Let's say you have implemented specific calculations and wish to display the received number as text. To do this, you are supposed to use the JavaScript to string method and convert the number to a string.
In case of an opposite situation, when you want to convert string to number JavaScript, you should apply the parseInt(). function. After it is applied, you will be able to use an integer (the whole number) in your calculations. You could also use the number() function to convert the string to a number, but parseInt()
is considered as a more practical choice.
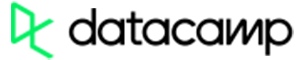
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
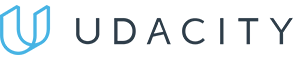
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
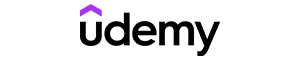
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Standard Syntax
To successfully turn a JavaScript int to string, you need to make sure you use the correct syntax. The following example reveals the basic syntax of the JavaScript toString function:
number.toString(radix)
As you can see, the JavaScript number to string function can take one optional parameter. It is referred to as radix
and indicates the way an integer is depicted in the string. You can add parameters from 2 to 36 to indicate the way numeric values are rendered.
Parameter | Description |
---|---|
radix | Optional. An integer (2-36) used as a base for representing a numeric value. |
2: A binary value. | |
8: An octal value. | |
16: A hexadecimal value. |
As you might expect, the return value of the JavaScript toString function is the string representing the number object.
Practical Code Examples
If you wish to master the JavaScript number to string function, we highly recommend that you practice using this method. Click Try it Live buttons to open the code editor and edit the code we provided!
In the example below, we convert a number 20 to a string:
function learnFunction() {
var number = 20;
var z = number.toString();
document.getElementById("learn").innerHTML = z;
}
Now in this example, we convert a JavaScript int to string, using different bases:
function learnFunction() {
var number = 20;
var a = number.toString();
var b = number.toString(2);
var c = number.toString(8);
var d = number.toString(16);
var z = a + "<br>" + b + "<br>" + c + "<br>" + d;
document.getElementById("learn").innerHTML=z;
}