When using JavaScript, you will often run into situations where you will have to use a loop. Part of the ability to work with loops and switches is being familiar with break and continue statements. In this tutorial, you will learn about their meaning and possible usages.
JavaScript break and continue statements are especially important when working with switch statements. Yet, you should know them when working with loops as well. You will also learn about label reference, used with break and continue statements.
Contents
JavaScript break and continue: Main Tips
- The JavaScript
break
statement stops a loop from running. - The
continue
statement skips one iteration of a loop. - These statements work on both loops and
switch
statements. - Both
break
andcontinue
statements can be used in other blocks of code by usinglabel
reference.
break
The break
statement stops executing a loop when a defined condition is met and continues running the code following the loop. In the example below, you can see the implementation of JavaScript break for loop:
for (i = 0; i < 15; i++) {
    if (i === 5) { break; }
    text += "Number: " + i + "<br>";
}
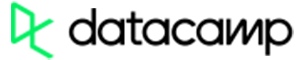
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
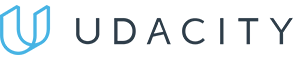
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
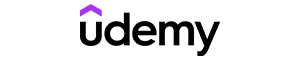
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
continue
The JavaScript continue statement stops the current iteration of the loop when a specified condition is met and proceeds the loop with the next iteration. Basically, it skips a defined iteration.
The example below skips the value of 5:
for (i = 0; i < 15; i++) {
    if (i === 5) { continue; }
   text += "Number: " + i + "<br>";
}
Label Reference
break
and continue
statements are usually used in loops and switch statements. However, they can be used within any block of code. In such cases you must use a label as shown below:
break labelname;
continue labelname;
In the example below, you can see a label reference used with a break JavaScript statement in a block of code. Here, the display of the list stops after displaying the first three items:
var phones = ["iPhone", "Samsung", "Nokia", "Motorola"];
list: {
text += phones[0] + "<br>";
text += phones[1] + "<br>";
text += phones[2] + "<br>";
break list;
text += phones[3] + "<br>";
}
Here you can see a label reference used with a JavaScript continue statement in a nested for
loop. A continue
statement is located within a for
loop labeled Loop2
. It is nested within another for
loop named Loop1
.
When j
is equal to 7, the continue
statement will make the loop skip this iteration, and make the Loop2
continue on iterating:
var text = "";
var i, j;
Loop1: // first loop
for (i = 0; i < 5; i++) {
text += "<br>" + "i = " + i + ", j = ";
Loop2: // second loop
for (j = 6; j < 10; j++) {
if (j === 7) {
continue Loop2;
}
document.getElementById("example").innerHTML = text += j + " ";
}
}
Note: labels are not very common, so you shouldn't overuse them.
JavaScript break and continue: Summary
- You may use JavaScript break for loops to stop them from running after a certain condition is met.
- The JavaScript
continue
statement skips an iteration and makes a loop continue afterwards. - These statements work on both loops and
switch
statements. - By adding a
label
reference, both of these statements can be used on other blocks of code.