JavaScript forEach: Main Tips
- JavaScript
forEach()
applies a specified function for every item in a particular array individually. - This method only executes the specified function in an array item which has a value.
- It's return value is
undefined
. - This loop was introduced with ECMAScript 3.
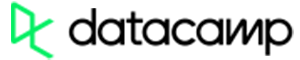
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
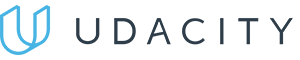
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
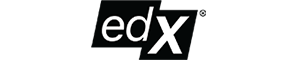
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Usage and Purpose of forEach
The JavaScript forEach()
loop is used when you want to inflict a specific method to an entire array.
Remember: arrays are lists of elements.
If you need to invoke a function to all of the array items, it's best that you apply the JavaScript array forEach loop.
The forEach()
code example we have here uses a number you input to multiply every number the array contains with, and display the result in the container name demo:
<button onclick="numbers.forEach(myFunction)">Multiply</button>
Now, this example takes every value in the array, and displays the sum of those values:
var sum = 0;
var numbers = [58, 45, 122, 787];
function myFunction(item) {
sum += item;
sumspan.innerHTML = sum;
}
There are no limitations when it comes to the functions you can call on elements with the JavaScript forEach()
. You can modify the items using any JavaScript function.
The specified method will not be applied to elements that have no value. For instance, the forEach JavaScript method will not affect sparse arrays in which most of the items do not have values.
The following example shows the usage of JavaScript forEach()
:
var numbers = [8, 2, 5];
numbers.forEach((item) => {
document.writeln(item);
});
forEach Syntax Explained
Take a look at the code snippet below. In it, you can see the correct syntax used or writing the JavaScript forEach()
:
array.forEach(function(current_Value, index, array), this_Value)
The forEach JavaScript can have two main parameters: the function
to be applied to the array items, and this_Value
. The specified function has its arguments as well:
- The
current_value
indicates the value of the currently-processed item. - The second parameter reveals the
index
of the same element. - The last argument is called
array
, and indicates the array the function is going to affect.
Note: only current_value is required to enter - the other parameters are optional.
Parameter | Description |
---|---|
function(current_value, index, array) | Needed. A function each array element to be run. |
this_Value | Optional. A value to be its this value. If skipped, it will be passed as undefined. |