It's nearly impossible not to encounter array when coding in JavaScript. In this tutorial, you will learn all about various JavaScript array methods.
You will understand how to convert string to array JavaScript code will use, or in other words, perform a data type conversion. We will also explain different ways to split arrays into smaller ones, remove items from different positions in the array, sort them, merge them, and much much more.
Contents
JavaScript Array Methods: Main Tips
- The biggest advantage of arrays in JavaScript is the variety of their methods.
- Array methods provide convenient ways to use arrays.
- There are multiple methods to manipulate arrays and their data.
Most Common Methods
A complete list of JavaScript array functions can be found here. However, we will now talk about the ones that are used most commonly. Simple code examples will also be provided to help you get a better idea in each case.
toString() Method
The toString()
method returns all the elements of an array as a string list:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
document.getElementById("text").innerHTML = cars.toString();
// output: Audi, Mazda, BMW, Toyota
join() Method
The join()
method puts all the elements of the array into a string list. The difference from toString()
method is that you can specify a separator (in our case, a dash - (" - ")
):
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
document.getElementById("text").innerHTML = cars.join(" - ");
// output: Audi - Mazda - BMW - Toyota
pop() Method
JavaScript pop()
method is used when you need to remove the last element of an array. It returns the removed item's value:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
cars.pop();
// New array: Audi, Mazda, BMW
push() Method
The push()
method adds a new specified element to the end of an array. It returns a numeric value that is the length of the new array:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
car.push("new");
// New array: Audi, Mazda, BMW, Toyota, new
shift() Method
This method, just like JavaScript pop()
method, removes an element from an array.
The difference between these two is that shift()
removes the first element. It is called shift because after removing the first element, it shifts all the other elements to a lower index:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
cars.shift();
// new array: Mazda, BMW, Toyota
unshift() Method
The unshift()
method adds a new element to the beginning of an array, and unshifts older elements. Then, it returns the new array length:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
cars.unshift("new");
// New array: new, Audi, Mazda, BMW, Toyota
splice() Method
The splice()
method can add and remove elements from an array.
The first parameter specifies the index where a new element should be inserted. The second specifies how many elements should JavaScript remove from array. The rest of the parameters set the values that will be added (this method can add multiple elements):
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
cars.splice(2, 0, "new", "newer");
// new array: Audi, Mazda, new, newer, BMW, Toyota
sort() Method
This method sorts an array alphabetically. You can learn more about all of the possibilities the sort()
method presents in the JavaScript sorting arrays tutorial:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
cars.sort();
// array: Audi, BMW, Mazda, Toyota
reverse() Method
This method reverses the order of the elements in an array. It can be used when sorting an array - this way, it will be sorted in descending order:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
cars.sort();
cars.reverse();
// array: Toyota, Mazda, BMW, Audi
concat() Method
JavaScript concat()
method joins two arrays and makes a new one. It can take any number of arguments:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
var people = ["Joe", "Leona"];
var joined = cars.concat(people);
// joined array: Audi, Mazda, BMW, Toyota, Joe, Leona
slice() Method
This method cuts out a part of an array and makes a new one. It can take one or two arguments: the first one specifies an index where to start slicing, and the second one sets the end index of the slice. If the second argument is not specified, it will slice to the end of the array:
var cars = ["Audi", "Mazda", "BMW", "Toyota", "Suzuki"];
var myCars = cars.slice(1, 3);
// myCars: Mazda, BMW
valueof() Method
This method converts the value of the array to a primitive one. The primitive value of an array is a string by default, so by default this method does exactly the same thing as toString()
method:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
document.getElementById("text").innerHTML = cars.valueOf();
// output: Audi, Mazda, BMW, Toyota
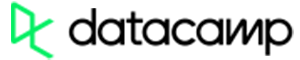
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
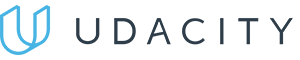
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
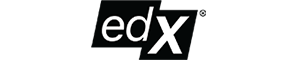
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Changing Elements
All array elements are accessed by their index number. You can change any array element by setting a new value to them:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
cars[0] = "Opel";
//cars: Opel, Mazda, BMW, Toyota
You can easily append new item to the end of an array by using its length
property:
var cars = ["Audi", "Mazda", "BMW", "Toyota"];
cars[cars.length] = "Opel";
// cars:Audi, Mazda, BMW, Toyota, Opel
Note: arrays start at 0. So, if you need to access the first element of an array, you must use 0 as an index.
Numeric Sort
The sort()
function works only on strings by default.
Sorting words this way is convenient: "Apple" comes before "Cactus". However, it does not work correctly when sorting numbers: according to the method, "36" is bigger than "101", because the first character "3" is bigger than "1". This can be fixed by specifying a compare function as a sort()
method's argument.
var numbers = [41, 102, 1, 8, 25, 10];
numbers.sort((a, b) => { return a - b });
// numbers: 1, 8, 10, 25, 41, 102
JavaScript Array Methods: Summary
- Arrays have a lot of methods you can use to manipulate them and their content.
toString()
andvalueOf()
are not methods unique to arrays. All JavaScript objects have these methods.- To make JavaScript remove from array properly, use JavaScript
pop()
orshift()
methods. - To perform array concatenation, use JavaScript
concat()
method.