Contents
JavaScript Array Functions: Main Tips
- A JavaScript array is an object that can store multiple values.
- Arrays start at 0. The first element is indexed as 0, the second as 1, and so on.
- Arrays can hold a set of any values: numbers, words or objects.
- JavaScript array functions provide an easy way to modify them.
Definition and Purpose
JavaScript array functions are designed to help you manage strings by putting them into lists of similar items. These methods are built-in, meaning that the creation and manipulation of arrays are standardized in JavaScript for your convenience.
For instance, an array called cars
could consist of different automobile manufacturers like Audi, Mazda, and Volvo. The following code illustrates this example and reveals the syntax of an array:
One of the most common JavaScript array functions is the array.filter()
method. It is applied when developers need to implement the JavaScript array filter task. This function will collect elements that comply with the specified rule (i.e., generate a list of words that are under three characters in length).
Another frequently used method is the array.copyWithin()
. It is applied when programmers need to quickly JavaScript copy array elements. In other words, you can copy a portion of the array to another place in the same array.
For more information about the standard methods for dealing with arrays, continue reading this tutorial!
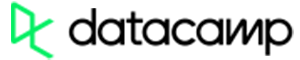
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
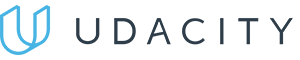
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
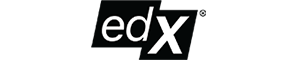
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Properties Explained
JavaScript array properties allow you to get information about the lists of elements you have generated. For instance, you can find out the function applied when an array was created using the constructor
property. It is also possible to receive information about the length of arrays by using length
. The prototype
property is used when developers want to add properties and methods to array objects.
Property | Description |
---|---|
constructor | Returns a function which was used in creating the array's prototype. |
length | Can be used to return and set the number of elements the array holds. |
prototype | Lets you add new methods and properties to the array object. |
Methods to Use
Manipulation of lists is implemented easily with these JavaScript array functions. We have already discussed a few of the methods that developers apply, but the following table introduces a variety of different actions you can perform on arrays.
For instance, the array.reverse()
is used when developers implement the reverse array JavaScript task. In other words, you can switch the order of elements in the list by putting the first item last, and vice versa.
Another useful example is the JavaScript array shift method (array.shift()
). It is used when it is necessary to delete the first element in an array. Furthermore, the removed element will be delivered as the return value.
The array.unshift()
method might seem similar to the previous function, but it actually has an opposite functionality. Unshifting arrays means adding an element (or a few) to the beginning of an array.
Method | Description |
---|---|
concat() | Joins multiple arrays. Returns a new array. |
copyWithin() | Copies elements from the array from one index to another. |
every() | Checks if all the elements of an array pass a test. |
fill() | Fills an array with some values. |
filter() | Creates a new array using every element that passes a test. |
find() | Creates a new array with all the elements that pass a test implemented by the provided function. |
findIndex() | Returns the index of the first element which passes a test. |
forEach() | Executes some code to each element of an array. |
indexOf() | Returns the index of a specified element. |
isArray() | Checks whether a specified element is in the array. |
join() | Puts array elements into a string list. |
lastIndexOf() | Returns an index of the specified element. Starts searching from the end of an array. |
map() | Executes a function for each element of an array and stores the result in a new array. |
pop() | Removes and returns the last element for the array. |
push() | Adds a new value to the end of an array and returns the new length of the array. |
reduce() | Reduces the values of elements in an array to a single value. |
reduceRight() | Reduces the values of elements in an array to a single value (starting from the right). |
reverse() | Reverses the order of the elements in an array. |
shift() | Removes and returns the first element from an array. |
slice() | Takes a part of an array and forms a new array. |
some() | Checks if at least one of the elements passes a test. |
sort() | Sorts the elements of an array alphabetically. |
splice() | Can be used to add or remove items from an array. |
toString() | Puts array elements into a string list. Returns the list. |
unshift() | Adds a new element to an array (at the beginning), and unshifts older elements (increases their index by one). Returns the new array length. |
valueOf() | Returns an array as a primitive value (usually a string). |