Contents
JavaScript RegEx: Main Tips
- Regular expressions are objects which describe a character pattern.
- Using regular expressions, you can perform pattern searches, in addition to functions which search-and-replace text.
What RegEx is
JavaScript RegEx (or RegExp) is a shorter name for the regular expressions JavaScript developers use in their codes. They are used for describing character patterns that are to be applied for pattern searches and creation of functions. In case you need a quick trick to replace specific portions of strings, it is also important to understand the purpose and meaning of JavaScript RegExp.
JavaScript RegEx indicates a pattern which is to be manipulated with different JavaScript functions. Regular expressions can consist mainly of ordinary characters like letters (text) but they can also contain special symbols (/
, *
, \
, .
).
Bear in mind that by applying JavaScript RegEx you are looking for a direct match, which means that only exact characters will be returned.
Click on the Try it Live button under the example below and see how it works:
Still a bit confused? Let's get the example explained:
/bit/gi
is a regular expression.bit
is the pattern that we can use in a search.g
is the modifier which makes the search return all possible results instead of one.i
is the modifier which modifies the search so it is not case-insensitive.
Syntax Step by Step
Seeing the example above, you might have gotten the idea already that the general syntax for writing a regular expression JavaScript looks like this:
/pattern/modifiers;
Pattern
refers to the standard or special characters you will attempt to perform functions on, while the modifiers
indicate the type of matching.
When you want to specify the type of matching for regular expression JavaScript, you should include a specific modifier. As you can see in the table below, they can be used for performing global or case-insensitive searches:
Modifier | Description |
---|---|
i | Specify to perform case-insensitive matching. |
g | Specify to perform global match (find every match instead of stopping at the first). |
m | Specify to perform multiline matching. |
Function of Brackets
If you want to be more specific when it comes to finding matches for functions like JavaScript replace RegEx, you can indicate the range. While by default only exact matches can be returned, brackets can specify that any character can be considered as a match:
Expression | Description |
---|---|
[abc] | Specify to find any character that is between brackets. |
[^abc] | Specify to find any character that is NOT between brackets. |
[0-9] | Specify to find any digit between brackets. |
[^0-9] | Specify to find any digit NOT between brackets. |
(x|y) | Specify to find any of the alternatives specified. |
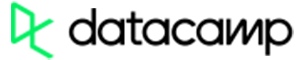
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
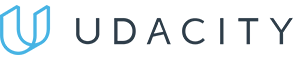
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
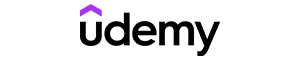
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Metacharacters: What They Are
Some characters are referred to as metacharacters as they have a special meaning in the pattern processing. Usually it's a backslash (\
) followed by a specific character, but it can also be a single symbol, such as a period (.
).
The following table explains the different symbols and combinations that can be applied. Notice that the case-sensitivity matters:
Metacharacter | Description |
---|---|
. | Specify to locate a single character (not the line terminator or newline!). |
\w | Specify to locate a word character. |
\W | Specify to locate a non-word character. |
\d | Specify to locate a digit. |
\D | Specify to locate a non-digit character. |
\s | Specify to locate a whitespace character. |
\S | Specify to locate a non-whitespace character. |
\b | Specify to locate a match at the beginning or the end of word. |
\B | Specify to locate a match not at beginning or the end of word. |
\0 | Specify to locate a NULL character. |
\n | Specify to locate a newline character. |
\f | Specify to locate a form feed character. |
\r | Specify to locate a carriage return character. |
\t | Specify to locate a tab character. |
\v | Specify to locate a vertical tab character. |
\xxx | Specify to locate a character that is specified with an octal number (xxx). |
\xdd | Specify to locate character that is specified with a hexadecimal number (dd). |
\uxxxx | Specify to locate a Unicode character that is specified with hexadecimal number (xxxx). |
Information on Quantifiers
JavaScript quantifiers could be called greedy. Why? Because they can only be satisfied with getting as many results as possible.
Quantifiers trigger RegEx to find as many matches of the specified pattern as possible. They indicate numbers of characters or expressions to match. See the table below for the list and explanations:
Quantifier | Description |
---|---|
n+ | Specify to match any string containing at least one n. |
n* | Specify to match any string containing none or more occurrences of n. |
n? | Specify to match any string containing none or one occurrence of n. |
n{X} | Specify to match any string containing a sequence of X n's. |
n{X,Y} | Specify to match any string containing a sequence of X to Y n's. |
n{X,} | Specify to match any string containing a sequence of at least X n's. |
n$ | Specify to match any string that ends with n. |
^n | Specify to match any string that starts with n. |
?=n | Specify to match any string followed by a specific string n. |
?!n | Specify to match any string not followed by a specific string n. |
RegExp Object Properties
When writing JavaScript RegExp, you should also understand the usage of various properties. They are used to get more information about the regular expressions.
Using properties, you can check the modifiers, constructors, and other details of JavaScript RegExp:
Property | Description |
---|---|
constructor | Return the function used to create RegExp object's prototype. |
global | Check whether the g modifier is set. |
ignoreCase | Check whether the i modifier is set. |
lastIndex | Specify index at which the next match will start. |
multiline | Check whether the m modifier is set |
source | Return RegExp pattern text. |
Possible Methods
In this section, we will cover some of the functions that might be related to JavaScript RegEx. Most of them have something to do with learning the content of strings. See the table below and memorize the functions for later use:
Method | Description |
---|---|
compile() | Compose a regular expression. Deprecated in version 1.5. |
exec() | Test for a match in the string and return the first one. |
test() | Test for match in string and return a boolean value. |
toString() | Return the string value of regular expression. |