Contents
JavaScript Random Number: Main Tips
- The JavaScript random number method returns a random number from 0 (inclusive) up to but not including 1 (exclusive).
- In order to get numbers from other ranges (e.g 0-100), you can multiply the result by a specific number.
- At the run time, the seed is selected automatically. The user can not choose or reset the seed.
Usage and Purpose Explained
The JavaScript Math.random()
method is applied when developers need to generate JavaScript random number. As a result, the function will deliver a floating-point number in a set scope: higher than 0 but lower than 1. You will need to use a library named Math.
Although the selection of numbers might be random, such an impression does not reflect the way JavaScript Math.random function works. It can be stated to be almost random since it performs as a pseudorandom number generator. Programming languages rarely present purely random numbers.
Therefore, the JavaScript math random function returns a number which can be identified as pseudorandom as it depends on the initial value called the seed.
If you are a website developer, we have a basic way for you to include the JavaScript random number function in your code. Let's say you want to add a button to randomly open an article from your site. With the JavaScript math random function, you can generate a random number and apply it to open a random page.
Below you can see the way that the JavaScript random number method is written. Pay attention that it has no parameters in the parentheses:
Math.random()
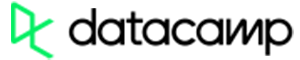
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
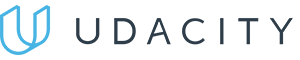
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
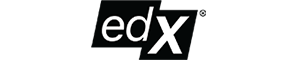
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Results: Return Value
It is important to note that JavaScript itself has little say when it comes to the random number JavaScript presents. In reality, this mostly depends on your browser. As a default, the JavaScript random number method will return numbers that are higher than 0 but less than 1.
The example below returns a float number between 0 and 1 (e.g., 0.28992849082058536)
Beginner-Friendly Practice Exercises
This tutorial covered many theoretical aspects of the JavaScript get number function. Now, it is time to take a look at the actual usage of the function used to make JavaScript generate random number. Do not forget to click the Try it Live buttons to open the code editor and make modifications to the code provided.
Using the examples below, we will introduce you ways to get random numbers in other ranges than 0-1. The first one will return a random number between 0 and 100:
Now, the example below will return a random number between 100 and 500: