Contents
JavaScript map Function: Main Tips
- The JavaScript map function is used to invoke a function for every element of an array in order.
- It should be noted that it does not execute the function on the elements without values.
- The JavaScript map method does not change the original array.
What map() Function Is
Map function JavaScript is one of the most practical methods to take advantage of if you want to invoke a specific function for all elements in an array. However, there are other functions that perform similar tasks: a forEach loop is one of the alternatives to consider.
The JavaScript map function is applied when it is necessary to call a function for every element of an array. From the generated results, the method will create a new array. Therefore, the first array will remain unchanged: all of the modifications to the elements will be represented in the brand-new array. If you apply the JavaScript map function, you should use the new array to make the usage of this method worthwhile.
In this example, we use JavaScript array map to multiply all elements in the array:
var nums = [64, 45, 11, 5];
function multiplyElement(num) {
return num * document.getElementById("multiplyBy").value;
}
function mapFunction() {
document.getElementById("result").innerHTML = nums.map(multiplyElement);
}
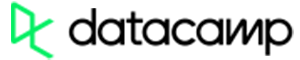
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
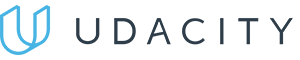
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
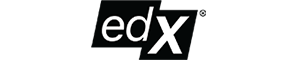
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Correct Syntax to Learn
In the following example, we present you with the correct way of writing the map function JavaScript. Firstly, you should indicate elements of which array should be modified with a specific function:
array.map(function(currentValue, index, arr), thisValue)
As you can see, the function to be applied to elements needs to be indicated in the parentheses of the map()
. Explanation of the function()
arguments will be provided in the following section.
Parameter Values: Optional and Required
There are a few components of the JavaScript map function that we need to discuss. As we have discussed in the previous section, it is necessary to specify the function to be applied to the array elements. The function parameter in this method can also take three arguments. However, only one of them is required.
The first argument in the function()
is called currentValue and represents the value of the element. Unlike the other two parameters, it must be included. The second optional argument is the index: it stands for the index of the element. The third one is arr, which represents the array that the element belongs to.
Parameter | Description |
---|---|
function(currentValue, index, arr) | Function that will be called for each element in array. Required. |
currentValue: Value of current element. Required. | |
index: Array index of current element. Optional. | |
arr: array object current element belongs to. Optional. | |
thisValue | Value to be passed to method's function to be used as its this value. Optional. Undefined if left empty. |
Return Value
As we have indicated before in this tutorial, the JavaScript map function will return a new array, consisting of modified elements. The original array will remain unchanged.
Return Value: | A new array that has an index similar to the original one, except that each element has now been affected by the specified function. |
---|---|
JavaScript Version: | ECMAScript 3 |
The following code shows how with the JavaScript map function the age and name can be retrieved from the people
array:
var people = [
{ name : "Dan", age: 32 },
{ name : "Joe", age: 45 },
{ name : "Brian", age: 28 }
];
function getNameAge(item, index) {
var nameAge = [item.name, item.age].join(" ");
return nameAge;
}
function mapFunction() {
document.getElementById("result").innerHTML = people.map(getNameAge);
}