Knowing how to increase your page load is one of the things every web developer should know. This tutorial will help you get a better understanding of what does JavaScript performance mean and how to improve it. JavaScript best practices that allow you to make your page load faster will be explained in detail.
You will understand how to write loops in a way that increases loading speed, learn about DOM access, and grasp the role it plays in slowing down your page. We will cover the usage of variables and their part in JavaScript optimizations.
Contents
JavaScript Performance: Main Tips
- This tutorial is going to help you speed up the performance of your scripts.
- Generally speaking, a well-structured code with practically handled variables and loops will always run fast enough.
Remove Unnecessary Code From Loops
Loops in programming are very common.
Every statement in a loop, including the for statement, is executed for every iteration of the loop. That is why only the code that needs to be looped should be in the loop's block of code. Let's see how to perform JS optimization in this case.
Bad Code
for (i = 0; i < myArray.length; i++) {
// Your code
}
Better Code
length = myArray.length;
for (i = 0; i < length; i++) {
// Your code
}
In the first case, the length
property is accessed with each iteration, although it does not need to be looped through each time and can be placed outside of the loop itself.
So, the second version moves it out of the looped code block, allowing the loop to iterate faster.
Reduce DOM Access
Accessing the DOM is rather slow, yet, you need it quite often in JavaScript.
If you do intend to use the DOM repeatedly, it's better to access it only once, using it in the form of a local variable.
elem = document.getElementById("myElement");
elem.innerHTML = "I'm in.";
Reduce DOM Size
You should try to avoid using too many elements and keep the HTML DOM small.
By not using too many elements, you will improve the loading time of your website, the rendering, which is especially noticeable on small devices. It can also be considered as JS optimization.
Every time the DOM needs to be searched for an element, a smaller amount of elements will benefit you.
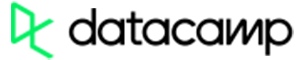
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
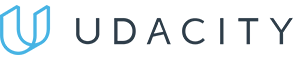
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
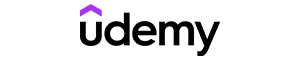
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Avoid Unnecessary Variables
If you are not planning to save a value, don't make a variable for it.
For example, here we have a piece of code where a variable doesn't need to be created:
var name = firstName + " " + lastName;
document.getElementById("myElement").innerHTML = name;
We can change it into this to improve the performance:
document.getElementById("myElement").innerHTML = firstName + " " + lastName
Delay JavaScript Loading
If you put your script at the bottom of the page, it will allow the page to be loaded before the code is applied.
While your script, typically found in another file, is loading, the browser cannot start any additional downloads and the rendering process. Parsing may be blocked as well.
Alternatively, you can use the defer="true"
inside the script tag. This attribute will specify that the script is not to be executed after the page finishes parsing; however, it will only work for external scripts.
Also, you can add the script to your page after the page loads using a code similar to the one in the example below.
window.onload = scriptsDown();
function scriptsDown() {
var elem = document.createElement("script");
elem.src = "javaScript.js";
document.body.appendChild(elem);
}
Note: according to the HTTP specification no two components should be downloaded in at the same time.
Avoid Using with
You should avoid the with
keyword. It clutters JavaScript scopes and can slow down the page.
with
keyword is not allowed when in strict
mode.
JavaScript Performance: Summary
- There are multiples ways to perform JavaScript optimizations.
- It's important to pay attention to how you write loops.
- Don't declare variables if they are not necessary.
- Try avoiding the
with
keyword.