JavaScript date objects can display date and time in differing formats, depending on the level of detail you aim to include. Dates are incorporated by using JavaScript get current date and JavaScript current time properties.
This tutorial will help you master JavaScript date object properties and features, and explain how the JavaScript date to string function can transform the content of date objects into strings. When you finish this chapter, we suggest following up with JavaScript date methods tutorial.
Contents
JavaScript Date Object: Main Tips
- JavaScript date object allows you to work with milliseconds, seconds, minutes, hours, days, months, and years.
- JavaScript date object can display a date in various formats.
- Date methods allow you to set years, months, days, hours, minutes, etc.
- If you are getting or setting a date without defining the time zone, the time will be chosen according to the browser's time zone.
- To learn more about JavaScript get current date and other properties, you should follow-up with the JavaScript Date Methods tutorial.
Creating Date Objects
The Date()
object allows you to format the date in various formats. In the example below, you can see four most common ways it is performed in JavaScript:
new Date()
new Date(milliseconds)
new Date(dateString)
new Date(year, month, day, hours, minutes, seconds, milliseconds)
If you want to get current time, create a new Date JavaScript object. If the time is not specified, JavaScript new Date()
property takes it from the browser and then displays it:
Note: months start at index 0 (January) and ends at 11 (December).
If you are using JavaScript new Date(string)
, it will create date object with specified time and date:
Using new Date(number)
creates a date with 0 time, which means the value of the date object will be 01 January 1970 00:00:00 UTC, plus the number you entered:
Using JavaScript date object new Date(7 numbers)
will create an object that will display the years, months, days, hours, minutes, seconds, and milliseconds of the date:
The code in the example below allows you to pass any four parameters:
Note: if you specify the year by only using two numbers, the program will read it as 19xx.
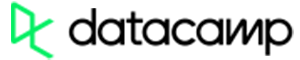
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
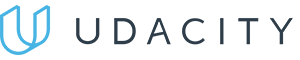
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
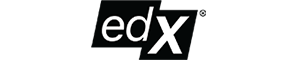
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Displaying Dates
Displaying dates in HTML code will automatically convert it to a string:
It is identical to using a toString()
method:
d = new Date();
document.getElementById("example").innerHTML = d.toString();
toDateString()
method converts date to a more readable format than the previous one:
var d = new Date();
document.getElementById("example").innerHTML = d.toDateString();
It is also possible to convert a JavaScript date to string. The toUTCString()
function will convert the date object to a UTC string (a date display standard):
var d = new Date();
document.getElementById("test").innerHTML = d.toUTCString();
JavaScript Date Object: Summary
- JavaScript date object displays the current date and time on your site.
- You can display either date, time, or both.
- If the date is not specified, JavaScript date object takes it from the browser and gives current time.