JavaScript is one of the main web programming languages, so it should always be functional when a website opens. To make sure it is, the developer must know when to use JavaScript global variables, and when it is better to stay with the local scope.
This tutorial will teach you everything about scope in JavaScript - you'll be familiar with JavaScript global variables, as well as local ones. If you are looking for JavaScript static variables though, remember this language doesn't support static keywords (unlike other languages, like C or C++).
Contents
JavaScript Scope: Main Tips
- Functions and objects are variables in JavaScript.
- A JavaScript variable scope defines the visibility (accessibility) of the variable. Variables can be local and global.
- JavaScript global variables are accessible throughout the whole web document.
- Local variables are available only in the current function.
Local vs. Global
We're sure your intention is to understand when a global or a local variable is a better choice, and learn how to use them. Still, practice can't start without covering the bases in theory. To make proper choices between the two, you first have to understand their differences.
We will now review each of the scopes carefully and provide examples to ensure you understand the material. Soon, JavaScript variable scopes will seem to you like a no-brainer!
Local Scope
Local function variables are the ones which are declared inside of a function in JavaScript. Local variables can be accessed only from within the said function. Therefore, you can't reach them from any other function in your document.
It's good to use local variables in JavaScript functions because it allows you to use variables with the same name, as long as they are local variables that stay within separate functions. While using local variables, you can only access them in the current function, which means the program will only access a variable that is in its scope.
// in here the "phone" variable can not be accessed
function simpleFunction(){
var phone = "smartPhone";
// here the "phone" variable can be accessed
}
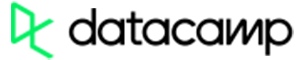
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
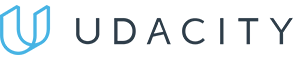
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
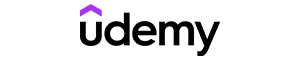
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Global Scope
All variables that are created outside of functions are called JavaScript global variables. Such objects can be accessed throughout the whole website. You must have different names for your global variables: otherwise, the browser will fail to present the action you want.
A global variable has a global JavaScript scope. All functions can access it throughout the web page:
var phone = "SmartPhone";
// code here can use "phone"
function simpleFunction() { Â
// code here can use "phone"
}
Set Global Variables Automatically
A variable can automatically become global if you set a value to a variable that has not yet been declared. This can happen even if you assign a value to a variable in JavaScript functions:
// code here can use "phone"
function myFunction() { Â
 Â
phone = "SmartPhone";Â Â Â
// code here can use "phone"
}
Note: you need to add var at the start of the statement to properly create a new local variable.
Note: to not create such global variable JavaScript should stay in strict mode while you work.
Global Variables in HTML
JavaScript global variables work in the whole JavaScript environment. From the first line of code, your global JavaScript variable scope begins and extends to the end of the script. Variables in JavaScript become available from the moment they are declared. They stop when the function ends.
However, JavaScript global variables belong to the window object in HTML and become available from the moment a person opens a browser window. Variables are deleted when the page is closed:
// code here can use window.phone
function simpleFunction() {
  Â
phone = "SmartPhone";
}
JavaScript Scope: Summary
- Local variables are available only within a function they have been declared in.
- Global variables are accessible throughout the whole web document.
- Variables that have not been declared, but have been assigned values (even within functions), become global automatically.
- If you wish to not automatically generate global variable JavaScript should stay in strict mode.
- In JavaScript static variables are not supported.