Files and various databases are useful for storing data, but as they get bigger, they might as well become easier to get lost in them. Finding information that you have to use in some particular moment, manually might take ages. The problem is that when you need something, you usually need it fast.
Making PHP read XML file can help you retrieve data quickly. XML is one of the most common formats for keeping data strings. If you prefer it as well, you can use AJAX to read XML files. In other words, AJAX can help you connect with the XML document that holds information and quickly find the exact data.
Contents
Read XML File: Main Tips
- By using AJAX, you can interactively communicate with XML documents and make PHP read XML files.
- The example in this tutorial will show you how to quickly fetch XML data using AJAX, XML and PHP.
First Step: Interface
When learning how to make PHP read XML files, let's hang on to the idea of a CD list as our data. To make a web application for a PHP reading XML, we will first need to create the interface. It will include a dropdown list and a container for the table: that is where the information about the selected album will be displayed.
Once a CD is elected from the dropdown list, show_cd()
function is called. Without it, there's no chance of our PHP reading XML files. An onchange
event triggers the specified function:
<html>
<head>
<script>
function showCD(str) {
if (str == '') {
document.getElementById("txt_hint").innerHTML = '';
return;
}
if (window.XMLHttpRequest) {
// script for browser version above IE 7 and the other popular browsers (newer browsers)
xmlhttpreq = new XMLHttpRequest();
} else {
// script for the IE 5 and 6 browsers (older versions)
xmlhttpreq = new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttpreq.onreadystatechange = function() {
//check if server response is ready
if (this.readyState == 4 && this.status == 200) {
document.getElementById("txt_hint").innerHTML = this.responseText;
}
}
xmlhttpreq.open("GET", "get_cd.php?q="+str, true);
xmlhttpreq.send();
}
</script>
</head>
<body>
<form>
Select a CD:
<select name="cds" onchange="show_cd(this.value)">
<option value="">Select a CD:</option>
<option value="Alice Cooper">Alice Cooper</option>
<option value="John Lennon">John Lennon</option>
<option value="Frank Zappa">Frank Zappa</option>
</select>
</form>
<div id="txt_hint"><b>This is where the info about CDs goes!</b></div>
</body>
</html>
Then, the show_cd()
function performs these actions:
- Checks whether a CD has been selected from the list.
- Creates an XMLHttpRequest object.
- Sets up the function to be prepared for execution once the server response is prepared.
- Sends the request to a PHP document (get_cd.php) located on our server.
Note: Be attentive when you apply q parameter to the get_cd.php?q="+str.
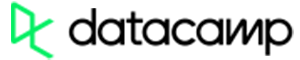
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
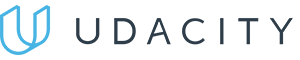
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
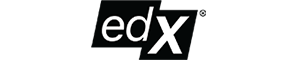
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Step Two: get_cd.php File
On the server, we have a PHP file called get_cd.php, and an XML file that contains all the necessary data about the musical albums. We called it cd_list.xml to fit its content. Now, we can make the PHP read XML files.
The PHP code will work in three stages. First, it will load the necessary XML file. Secondly, it will retrieve the necessary data from it by running a query. Finally, the results will be presented in the form of HTML:
<?php
$q = $_GET["q"];
$xml_doc = new DOMDocument();
$xml_doc->load("cd_list.xml");
$x = $xml_doc->getElementsByTagName('MUSICIAN');
for ($i=0; $i< = $x->length-1; $i++) {
//Set only to process nodes from elements
if ($x->item($i)->nodeType==1) {
if ($x->item($i)->childNodes->item(0)->nodeValue == $q) {
$y=($x->item($i)->parentNode);
}
}
}
$cd = ($y->childNodes);
for ($i=0; $i<$cd->length; $i++) {
//Set only to process nodes from elements
if ($cd->item($i)->nodeType==1) {
echo("<b>" . $cd->item($i)->nodeName . ":</b> ");
echo($cd->item($i)->childNodes->item(0)->nodeValue);
echo("<br>");
}
}
?>
Once the query is sent to the PHP using JavaScript, this is what happens:
- An XML DOM object is generated by PHP.
- Among all the
<musician>
elements, the suitable (matching the name received from JavaScript) ones are found. - The information about the selected CD is outputted by sending it to the
txt_hint
container. - We celebrate how beautifully we made our PHP read XML file.
Read XML File: Summary
- To use your PHP code for reading XML data strings, AJAX is needed.
- A combination of AJAX, XML and PHP lets the developer quickly find and access the data they wish to quickly retrieve.