If you are an active Internet user, you have probably seen this phrase: Your session has expired. It often shows up when you reopen a page after an extended period of inactivity. As a result, you don't get automatically logged in, and the functions of a specific webpage are no longer available.
PHP sessions are the reason why data becomes accessible to all webpages of a particular application. That particular data, now held in the form of variables, is temporarily stored on the server. When you close the window or tab of the browser, the session will be finished.
In this tutorial, we will speak about what PHP sessions are, how they start or end. We will also get familiar with PHP session variables and understand what PHP $_SESSION means.
Contents
PHP Sessions: Main Tips
- A session is a method of storing data (using variables) so the browser can use it throughout multiple webpages.
- In contrast to cookies, the data is not kept on the user's system.
- Session variables contain data about the current user. They are accesible to all pages contained in a single web application.
- Session data is not permanent, but you can load permanent user data for particular users using databases.
Starting a Session
To start PHP sessions, you must use the function session_start()
.
To set session variables, you will need to apply a global PHP $_SESSION
variable .
In the example below, we begin the PHP file demo_session1.php. Let's take a look at how we do just that:
<?php
// Start the session
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Set session variables
$_SESSION["color"]= "blue";
$_SESSION["animal"]= "dog";
echo "The session variable are set up.";
?>
</body>
</html>
Note: The PHP session_start() function has to be the first thing in your document: all HTML tags come after.
Getting Values of Variables
To continue, we create demo_session2.php. Using this file, we will access the data on demo_session1.php. Notice how the session data (in form of variables) must be individually retrieved (PHP session_start()
function).
See the example below to get a better understanding on how PHP $_SESSION
variable holds all of the session data declared in your files:
<?php session_start(); ?>
<!DOCTYPE html>
<html>
<body>
<?php
// Echo session variables that were set on previous page
echo "The color of my choice is " . $_SESSION["color"] . ".<br>";
echo "The animal of my choice is " . $_SESSION["animal"] . ".";
?>
</body>
</html>
In the script example below, you can see how you can display all of the currently declared session variables:
<?php session_start(); ?>
<!DOCTYPE html>
<html>
<body>
<?php
print_r($_SESSION);
?>
</body>
</html>
Wondering how does a session know whose it is? It is simple: sessions create a user identifier on the users' computers. It is a string of 32 random characters (for instance, 3c7ght34c3jj9083hjaje2fc650e344t).
When a session starts in another window, it checks for the previously created user identifier and continues if it is found.
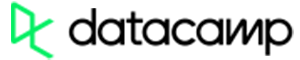
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
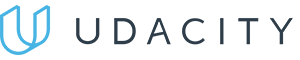
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
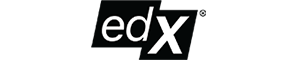
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Modification of Variables
You can change session variables by merely overwriting them:
<?php session_start(); ?>
<!DOCTYPE html>
<html>
<body>
<?php
// you can change a session variable by assigning a new value
$_SESSION["color"] = "red";
print_r($_SESSION);
?>
</body>
</html>
How Session Is Destroyed
session_unset()
will remove all of the global variables, while session_destroy()
will destroy the session entirely. However, both have a similar effect:
<?php session_start(); ?>
<!DOCTYPE html>
<html>
<body>
<?php
//remove all session variables
session_unset();
// destroy the session
session_destroy();
?>
</body>
</html>
PHP Sessions: Summary
- A method of storing variables that contain data for the browser to use is called a session. This data is not permanent and is not saved in the personal computers of users (unlike cookies).
- Information about the current user is kept in the session variables and accesible to all the pages of a web application. The global PHP
$_SESSION
variable stores values of all session variables. - To finish the session, you should simply close the window or tab in which a website was loaded.
- It is possible to load permanent user data for particular users using databases.