PHP operators are symbols used to make code perform specific actions. For example, the PHP or operator checks multiple conditions at once and determines whether any of them are true. The values a particular operator uses are called operands.
This topic is beginner-friendly as most of the symbols are easy to recognize: + is used to add, - to subtract, and * to multiply. However, some, like PHP .= operator, are more complicated to learn.
Do not assume operators are similar to functions. There are cases in which some operators (such as the PHP ternary) can be used instead of functions.
Contents
PHP Operators: Main Tips
- PHP operators execute various actions with values and variables.
- Arithmetic, comparison, assignment, increment or decrement, string, logical, and array operators serve different purposes in the code.
- Operators can be used with functions to modify already declared values.
- In PHP 7, spaceshi operators was introduced.
Meaning and Usage of Different Operators
Arithmetic
These operators are meant to be used with numeric values to perform basic arithmetic actions (such as adding or multiplying). In the table below, you can see how they are used and what results are achieved.
Operator | Name | Example | Result |
---|---|---|---|
+ | Add | $z + $x | Sum of $z and $x |
- | Subtract | $z - $x | Difference of $z and $x |
* | Multiply | $z * $x | Product of $z and $x |
/ | Divide | $z / $x | Quotient of $z and $x |
% | Modulus | $z % $x | Remainder of $z divided by $x |
** | Exponentiate | $z ** $x | Result of raising $z to the $x'th power |
See how they work in a simple example:
echo($x + $y), "\n";
echo($x - $y), "\n";
echo($x * $y), "\n";
echo($x / $y), "\n";
echo($x % $y), "\n";
echo($x ** $y), "\n";
Note: the exponentiate operator has only been introduced in PHP 5.6. If you are using older versions, it will be invalid.
Assignment
Operators in this category assign values to variables. Most commonly used assignment operator is =
, which assigns the variable on the left the value on the right side of the operand.
See the table below for the list and descriptions of each individual PHP operator:
Assignment | Same as... | Description |
---|---|---|
z = x | z = x | The variable on the left gets value of the variable on the right |
z += x | z = z + x | Adds the value on the left of the operand to the value on the right |
z -= x | z = z - x | Subtracts the value on the left of the operand from the value on the right |
z *= x | z = z * x | Multiplies the value on the left of the operand by the value on the right |
z /= x | z = z / x | Divides the value on the left of the operand by the value on the right |
z %= x | z = z % x | Displays the modulus of the value on the left of the operand by the value on the right |
View an example of their usage:
$a = 17;
echo $a, "\n";
$b = 4;
$b += 12;
echo $b, "\n";
$c = 38;
$c -= 10;
echo $c, "\n";
$d = 3;
$d *= 7;
echo $d, "\n";
$e = 16;
$e /= 4;
echo $e, "\n";
$f = 20;
$f %= 5;
echo $f;
Comparison
To check for certain differences or similarities between values and variables, this type of PHP operator is needed. It works like booleans, returning a value of either true
or false
. For example, using PHP not equal to operator will display true
if the two variables you are comparing are not equal in value.
Operator | Name | Example | Result |
---|---|---|---|
== | Equal to | $z == $x | Returns true if $z is equal to $x |
=== | Identical to | $z === $x | Returns true if $z is equal to $x, and they are of the same type |
!= | Not equal to | $z != $x | Returns true if $z is not equal to $x |
<> | Not equal to | $z <> $x | Returns true if $z is not equal to $x |
!== | Not identical to | $z !== $x | Returns true if $z is not equal to $x, or they are not of the same type |
> | Greater than | $z > $x | Returns true if $z is greater than $x |
< | Less than | $z < $x | Returns true if $z is less than $x |
>= | Greater than or equal to | $z >= $x | Returns true if $z is greater than or equal to $x |
<= | Less than or equal to | $z <= $x | Returns true if $z is less than or equal to $x |
See what boolean values they return in an example:
var_dump($x == $z);
var_dump($x === $z);
var_dump($x != $y);
var_dump($x !== $z);
var_dump($x < $y);
var_dump($x > $y);
var_dump($x <= $y);
var_dump($x >= $y);
Comparison operators can also establish conditions. This functionality is similar to the if()
function. For example, a PHP ternary operator consists of three operands: a particular condition and results for each true
and false
. They are separated by a question mark and a colon:
<?php
$weatherstr = ($temp < 20) ? 'spring' : 'summer';
?>
Note: make sure not to overuse PHP ternary operator as it makes your code harder to read.
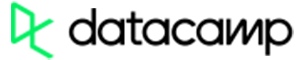
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
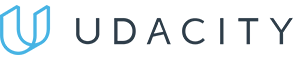
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
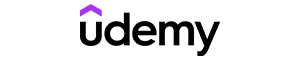
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Increment/Decrement
These operators increase (increment) or decrease (decrement) the value of a variable. They are sometimes called unary operators because they work on just one operand. See the table below to get a better idea:
Operator | Name | Description |
---|---|---|
++$z | Pre-increment | increases $z by one, then returns $z |
$z++ | Post-increment | Returns $z, then increments $z by one |
--$z | Pre-decrement | Decreases $z by one, then returns $z |
$z-- | Post-decrement | Returns $z, then decrements $z by one |
See how they work in an example below:
$x = 25;
echo ++$x;
echo $x;
$x = 25;
echo $x++;
echo $x;
$x = 25;
echo --$x;
echo $x;
$x = 25;
echo $x--;
echo $x;
Logical
These operators combine conditionals and are mostly used to check multiple conditions at once. For example, PHP or
operator checks whether at least one value out of two is true. If you need to check whether both of them are true, you should use PHP and
operator.
See the explanations in the following table:
Operator | Name | Example | Result |
---|---|---|---|
and | And | $z and $x | True if both $z and $x are true |
or | Or | $z or $x | True if either $z or $x is true |
xor | Xor | $z xor $x | True if either $z or $x is true, but not both |
&& | And | $z && $x | True if both $z and $x are true |
|| | Or | $z || $x | True if either $z or $x is true |
! | Not | !$z | True if $z is not true |
See an example of how this works:
if ($x == 17 and $y == 13)
echo "True";
if ($x == 14 or $y == 13)
echo "True";
if ($x == 17 xor $y == 9)
echo "True";
if ($x == 17 && $y == 13)
echo "True";
if ($x == 17 || $y == 14)
echo "True";
if (!($x == 13))
echo "True";
String
There are two operators for strings. Using them on numerical values might bring unexpected results:
- PHP
.
operator is also called the concatenation operator. It links separate strings together. - PHP
.=
operator is known as the concatenating assignment operator. It appends the argument on the right side to the one on the left.
Compare their usage using an example:
$x = "Bit";
$y = "De";
$z = "gree";
echo $x . $y . $z, "\n";
$x .= $y . $z;
echo $x;
Array
To compare separate arrays, use the operators listed below. Make sure you use the correct syntax:
Operator | Name | Example | Result |
---|---|---|---|
+ | Union | $z + $x | Union of $z and $x |
== | Equality | $z == $x | Returns true if $z and $x have the same key/value pairs |
=== | Identity | $z === $x | Returns true if $z and $x have the same key/value pairs in the same order and of the same types |
!= | Inequality | $z != $x | Returns true if $z is not equal to $x |
<> | Inequality | $z <> $x | Returns true if $z is not equal to $x |
!== | Non-identity | $z !== $x | Returns true if $z is not identical to $x |
See an example of their usage in the code editor:
var_dump($x == $y);
var_dump($x === $y);
var_dump($x != $y);
var_dump($x <> $y);
var_dump($x !== $y);
Spaceship
PHP7 brought a new kind of operator called spaceship operator. Similarly to some other kinds of operators, it compares two values (integers, floats, or strings). However, it returns an integer instead of a boolean value:
Case | Result |
---|---|
$x < $y | -1 |
$x > $y | 1 |
$x = $y | 0 |
See how it works in the example below:
PHP Operators: Summary
- Operators are used with variables for various actions or with functions for changing previously declared values.
- Operators are divided into seven groups based on their purpose: arithmetic, comparison, assignment, increment or decrement, string, logical, and array.
- PHP 7 introduced a new kind: the spaceship operator.
- PHP ternary operator can be used instead of
if()
function, though such code will be less readable.