As you learn PHP basics, you have to pay close attention to the syntax. It is a set of rules on how you write if you wish for your text (in this case, PHP code) to be understood correctly and work its magic as it should.
If you don't follow PHP syntax, all your hard work will be in vain: even if you know the functions by heart, the server will not be able to understand and execute your commands.
To tame the chaos, in 2009, PHP Framework Interop Group introduced a set of documents called The PHP Standards Recommendation. While it's not necessary to know every word, the basics are a must. That is exactly why we prepared a simple tutorial on PHP code syntax for you to follow and use as a cheat sheet.
Contents
PHP Code: Main Tips
- Every PHP script has to stay between between
<?php
and?>
. - Every PHP statement must end with a semicolon (;).
- Only variables are case-sensitive: functions or keywords are not.
Basic PHP Syntax
A PHP file usually consists of HTML tags and PHP script, and its default file extension is .php. You can put PHP script anywhere in the document, as long as you surround it with <?php
and ?>
:
<?php
// Here is PHP code
?>
This is a simple PHP example with echo
statement:
<!DOCTYPE html>
<html>
<head>
<style>
</style>
</head>
<body>
<h1>My first PHP web page</h1>
<?php echo "Hello Drago!"; ?>
<script>
</script>
</body>
</html>
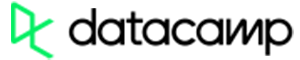
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
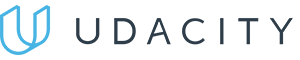
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
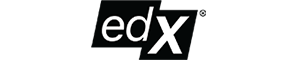
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Comments in PHP
A comment in PHP code cannot be executed as a program: it can be seen only by the developer reading the code. Mostly PHP comments are used as friendly reminders or explanations.
As you code in a rush of passion, you might sometimes leave little elements that your brain will not remember later. That is how comments in PHP can be helpful not only to others who view your PHP code but also to yourself.
You might also comment out PHP code lines - leave parts of it hidden. Maybe you'll return to them later, or maybe your colleague will find it a helpful suggestion outside the PHP basics box.
In the example you can see different types of PHP comments that are supported:
<!DOCTYPE html>
<html>
<head>
<style>
</style>
</head>
<body>
<?php
// A comment in the single line
# Another way of a comment in the single line
/*
A comment in
the multiple
lines
*/
// A code line parts can be left out with comments
$number = 19 /* + 39 */ + 12;
echo $number;
?>
<script>
</script>
</body>
</html>
PHP Case Sensitivity
In the rules of PHP syntax, case-sensitivity does not apply to any keywords (else
, if
, echo
, while
, etc.), functions, user-defined functions or classes.
In the example below all three echo
lines are equal and produce exactly the same result.
<?php
ECHO "Hello, Drago!<br>";
echo "Hello, Drago!<br>";
EcHo "Hello, Drago!<br>";
?>
However, variable names are always case-sensitive.
First echo
statement that you can see in the example below (the one that begins with $name
) will be displayed correctly. The other two will not: $NAME
and $nAmE
are treated as different variables because they are written in a different way in the PHP code.
<?php
$name = "John";
echo "I love " . $name . "<br>";
// echo "This is " . $NAME . "<br>";
// echo "My friend is " . $nAmE . "<br>";
// uncoment lines above to try how it doesn't work.
?>
PHP Code: Summary
- You should always surround your PHP code with
<?php
and?>
and end your statements with semicolons (;). - Functions or keywords aren't case-sensitive, but variables are.