PHP include file functionality means taking one PHP file and inserting it straight into the other. The code then treats it the same as a copied element.
Using this can save you a lot of time. If you copied and pasted the same piece to multiple places, you would have to update each one separately whenever a need arises. If you make PHP include file, you only need to modify the code in the original version of the inserted file.
Before you start using in your daily work, you have to get familiar with two statements: PHP require and PHP include. In this tutorial, we will help you do just that.
Contents
PHP include File: Main Tips
- PHP
include
andrequire
statements are used to take all the code or text a certain file contains and to insert it where the statement is used. - Dividing code into large sections like that makes it easier to read or modify.
- Using PHP
include
andrequire
statements, you can create various templates to be used repeatedly (such as a standard header or footer). This is especially useful in frameworks, web applications and content management systems (CMS).
Correct Syntax Explained
You already know that to make a function behave as it should you must closely follow the correct syntax in our scripts. Now, we wish to successfully PHP include file. Let's see how it should look in this case:
include 'filename';
or
require 'filename';
Note: there are no requirements to use parentheses (), just like when using print and echo statements.
Usage of Statements: Examples
In this section, we will review the usage of PHP include
file method with code examples. After analyzing the way this statement is applied, you will be able to simplify your coding process as you won't need to copy portions of code to different web pages.
Footer
Let's begin by viewing a standard footer described in HTML. This is what such code would look like:
<html>
<body>
<h1>Welcome!</h1>
<?php
require 'no_file_exists.php';
echo "I have a " . $color . " " . $car . ".";
?>
</body>
</html>
To PHP insert file that contains the script used to display the footer where we want it, we use the PHP include
statement:
<html>
<body>
<h1>Welcome!</h1>
<p>Text line.</p>
<p>Texty text line.</p>
<?php include 'footer.php';?>
</body>
</html>
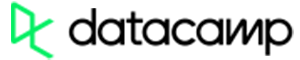
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
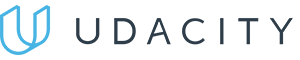
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
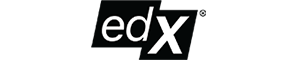
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Menu
Now, here is a file called menu.php:
<li>
<a href="home.php">Home</a>
<a href="about.php">About</a>
<a href="contacts.php">Contacts</a>
</li>
Imagine you want each page on your personal website to have a menu element. To make the code work the way we want, you should apply this code:
<html>
<body>
<div class="menu">
<?php include 'menu.php'; ?>
</div>
<h1>Welcome!</h1>
<p>Text line.</p>
<p>Texty text line.</p>
</body>
</html>
Variables
When you decide to PHP include files that hold variables, bear in mind the system treats both of your codes as one creation. Therefore, it doesn't matter if a particular variable is declared in the code you included or the one you included it to: it will apply in both.
First, we should have a file called variables.php, which contains some of the variables we need:
<?php
$color = 'red';
$car = 'bugatti';
?>
If we need our code to use the variables declared in variables.php, we will PHP include
file into our script:
<html>
<body>
<h1>Welcome!</h1>
<?php
include 'variables.php';
echo "I have a " . $color . " " . $car . ".";
?>
</body>
</html>
Differences Between PHP include and require
In the examples above, we used the include
function. You can also use the require
statement for PHP includes, though there is one difference between those two that you should keep in mind.
Using include
allows the script to continue even if the specified file cannot be accessed. It will run just like it would if there was no file included:
<html>
<body>
<h1>Welcome!</h1>
<?php
include 'no_file_exists_oh_no.php';
echo "I have a" . $color . " " . $car . ".";
?>
</body>
</html>
However, if we use require
, the system understands the included file as neccesary. Therefore, if the specified file isn't found or cannot be accessed otherwise, we will catch a fatal error, and the rest of the code will not be executed:
<html>
<body>
<h1>Welcome!</h1>
<?php
require 'no_file_exists.php';
echo "I have a " . $color . " " . $car . ".";
?>
</body>
</html>
Note: require is used when the file is absolutely crucial to the execution of the code. include is used when the presence of the file may be optional.
PHP include File: Summary
- When you use
include
andrequire
statements in your PHP scripts, code or text from an external file is placed right there in the script. - This lets you save time as you can create certain templates and reuse them multiple times.
- If you use the same block of code in different places, inserting it as a file makes it easier to modify later.