A cookie is a small file for tracking users' behavior online and for customizing web sites according to this information. PHP setcookie() function prepares a cookie to be transferred with other HTTP headers.
Contents
PHP setcookie: Main Tips
- PHP developers set cookies to identify users by their browsing habits and usernames.
- Cookies are small documents embedded on the personal computers of users. Each time a web application loads on the same computer, it uses cookie data.
- PHP allows you to retrieve and create cookie data. Functions that you will use the most for that are PHP
setcookie()
andisset()
.
The Use of setcookie() Function
While creating a cookie, here are the most common parameters of the PHP setcookie
function:
setcookie(name, value, expire, path, domain, secure);
See how PHP sets a cookie:
<?php
setcookie('our_cookie', 'current', time() + 3600, '/');
?>
<html>
<body>
<?php
if(count($_COOKIE) > 0) {
echo "Enabled.";
} else {
echo "Disabled.";
}
?>
</body>
</html>
Tip: PHP cookies must be transferred before any other script output. This rule means that you need to invoke the PHP setcookie() function before <html> and <head> elements.
The explanation of all parameters and their purposes:
Parameter | Definitions |
---|---|
Name | The name of the PHP cookie. Required. |
Value | Value of the variable specified. Optional, but recommended to include. |
Expire | Expiration date of the cookie. Optional. If omitted, the cookie expires at the end of the session. |
Path | Directories in which the cookie works. Optional. If set to /, the cookie works in the entire domain. /foo/ sets the cookie to work in /foo/ directory and its sub-directories. If omitted, the cookie works in the directory it was sent to. |
Domain | Domain name in which the cookie works. Optional. |
Secure | Transmission type (0 if HTTP, 1 if HTTPS). Optional. |
httponly | When true, the cookie is usable only through the HTTP protocol. |
Options | An associative array that might contain any of the keys: expires, domain, path, secure, httponly, and samesite. |
Note: the cookie value is URLencoded automatically upon sending the specific cookie and decoded once received. If you wish to avoid URLencoding, use setrawcookie().
Modifying Cookies
To modify existing PHP cookies, use the same PHP setcookie()
function you used to make PHP set cookie:
<?php
$name = 'user_cookie';
$value = 'Alexander Portman';
setcookie($name, $value, time() + (86400 * 80), '/');
?>
<html>
<body>
<?php
if(!isset($_COOKIE[$name])) {
echo "Cookie called '" . $name . "' has not been set!";
} else {
echo "Cookie '" . $name . "' has been set!<br>";
echo "Value in cookie is: " . $_COOKIE[$name];
}
?>
</body>
</html>
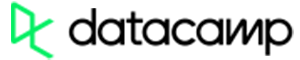
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
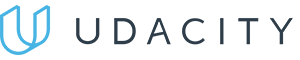
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
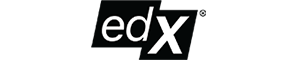
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Deleting Cookies
PHP setcookie()
function can delete cookies as well. The trick is setting the expiration date in the past. Cookies become expired:
<?php
// set the expiration date to one hour ago
setcookie('user_cookie', '', time() - 3600);
?>
<html>
<body>
<?php
echo "Cookie called 'user_cookie' has been deleted.";
?>
</body>
</html>
Method to Create and Retrieve
The code below generates a cookie called user_cookie along with a value of Johny Dawkins. The expiration date of the cookie is 80 days (86400 * 80 - 86400 is the number of seconds in one day) from now.
<?php
$name = 'user_cookie';
$value = 'Johny Dawkins';
setcookie($name, $value, time() + (86400 * 80), '/');
// 86400 = 1 day
?>
<html>
<body>
<?php
if (!isset($_COOKIE[$name])) {
echo "Cookie called '" . $name . "' has not been set!";
} else {
echo "Cookie '" . $name . "' has been set!<br>";
echo "Value in cookie is: " . $_COOKIE[$name];
}
?>
</body>
</html>
We can use a global variable called $_COOKIE
to retrieve the value stored inside user_cookie. The PHP isset()
checks whether a cookie is set.
Reminder: if you detect PHP setcookie not working, make sure it appears before the <html> element in your code, and that the set path parameter is correct.
Check If Cookies Are Enabled
isset
in PHP will tell you whether cookies are set. However, you still need to know whether they are enabled? The example below shows how you can check that in the script.
First, attempt to create a test cookie using PHP setcookie()
function. Then count the array $_COOKIE
:
<?php
setcookie('our_cookie', 'current', time() + 3600, '/');
?>
<html>
<body>
<?php
if(count($_COOKIE) > 0) {
echo "Enabled.";
} else {
echo "Disabled.";
}
?>
</body>
</html>
PHP setcookie: Summary
- The cookie is a file websites store in their users' computers.
- Cookies allow web applications to identify their users and track their activity.
- To set cookies, PHP
setcookie()
is used. - To see whether cookies are set, use PHP
isset()
function.