AJAX polling lets us quickly get the standpoints of everyone who takes a moment to press a button on the screen. It's that simple: they choose an option, and the results are generated immediately.
Learning to use PHP and AJAX is very efficient for anyone handling data, as the combination of these two systems lets you send information from browser to server and back. It can help you fulfill different tasks, such as create an AJAX polling system. Forms created this way are rather lightweight and easy to use.
Because of a widely-used coding language that is PHP, it's accessible to most developers with some experience. With the functionalities of AJAX, any PHP voting system can display the results without reloading the whole web page. This tutorial explains the ways of creating PHP vote system that will require users' responses.
Contents
AJAX Polling: Main Tips
- By using PHP and AJAX, you can make interactive polls that will instantly display results without slowing down your website.
- A PHP voting system infused with AJAX will help you collect data that you can later put to good use.
- Such PHP polls are often used in various news websites to find out what their readers think of a particular topic.
First Step: HTML File
Let's use an example of a simple PHP poll to make everything clearer. We will prepare an HTML with two options for answering one question. After a selection has been made, AJAX voting system with display the results without reloading the page.
This is achieved by calling get_votes()
when a selection is made. This function will be triggered by an onclick
event. Let's look at a PHP poll script to see how this works:
<html>
<head>
<script>
function get_votes(int) {
if (window.XMLHttpRequest) {
// script for browser version above IE 7 and the other popular browsers (newer browsers)
xmlhttpreq = new XMLHttpRequest();
} else {
// script for the IE 5 and 6 browsers (older versions)
xmlhttpreq = new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttpreq.onreadystatechange = function() {
//check if server response is ready
if (this.readyState == 4 && this.status==200) {
document.getElementById("ajax_poll").innerHTML=this.responseText;
}
}
xmlhttpreq.open("GET","poll_votes.php?res="+int,true);
xmlhttpreq.send();
}
</script>
</head>
<body>
<div id="ajax_poll">
<h3>Is Bitdegree Learn a good learning platform?</h3>
<form>
Yes!
<input type="radio" name="res" value="0" onclick="get_votes(this.value)">
<br>No!
<input type="radio" name="res" value="1" onclick="get_votes(this.value)">
</form>
</div>
</body>
</html>
AJAX voting system won't be able to perform without the get_vote()
function. Let's see how it's executed:
- We make an XMLHttpRequest object.
- We set up a function. It will be ready to execute once the server response is prepared.
- The request is sent to a PHP document (poll_votes.php) located on our server.
Note: Pay attention how you apply q parameter to the poll_votes.php?q="+int (Yes/No value).
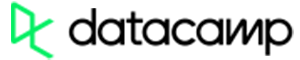
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
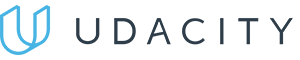
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
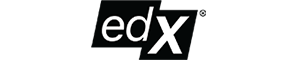
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
PHP Vote System: poll_vote.php File
In the example below, you can see how Javascript sends a request to the server. It is sent to access the poll_votes.php file, neccesary for the AJAX polling system:
<?php
$res = $_REQUEST['res'];
//get content of the text file
$file_name = 'poll_result.txt';
$content = file($file_name);
//put content in an array
$arr = explode('||', $content[0]);
$y = $arr[0];
$n = $arr[1];
if ($res == 0) {
$y = $y + 1;
}
if ($res == 1) {
$n = $n + 1;
}
//insert votes into text the file
$insert_vote = $y."||".$n;
$fp = fopen($file_name, 'w');
fputs($fp,$insert_vote);
fclose($fp);
?>
<h2>Results:</h2>
<table>
<tr>
<td>Yes!:
<img src="poll_results.png"
width='<?php echo(200*round($y/($n+$y),2)); ?>'
height='20'>
<?php echo(200*round($y/($n+$y),2)); ?>%
</td>
</tr>
<tr>
<td>No!:
<img src='poll_results.png'
width='<?php echo(200*round($n/($n+$y),2)); ?>'
height='20'>
<?php echo(200*round($n/($n+$y),2)); ?>%
</td>
</tr>
</table>
JavaScript sends out the value. What happens next?
- The content of the poll_results.txt file is received and placed in variables.
- One is added to the value of the selected variable.
- Results are written into the poll_results.txt file.
- A graphical representation of the PHP poll result is displayed.
Text File for PHP Poll Results
Results of every AJAX polling must be stored somewhere. For this example, we will store the result data for the PHP vote system we created in a text document called poll_results.txt. Here we can see how it will be stored:
0||0
The first number in the table is meant to represent voters who pressed Yes in the PHP poll. The second one represents the No votes.
Remember: Give exclusive access for the server to edit the file.
AJAX Polling: Summary
- PHP voting system is a great way to collect various data.
- AJAX will help you can make your polls interactive and dynamic.
- This kind of voting attracts more users as the results are displayed instantly.