When you’re coding in PHP, your code holds multiple variables more often than not. It’s not that hard to get lost among them either. To make PHP check if a variable exists, you can use PHP isset(). Its name is rather self-explanatory: by using isset in PHP, you check whether a particular variable in the script is set or not.
Contents
PHP isset: Main Tips
- By using
isset
in PHP, you can check whether a particular variable is set. - It returns a boolean value,
True
meaning a variable is set. - Just as echo and print, isset in PHP is a language construct and not a function.
- To unset a particular variable in PHP, use the
unset()
function.
How to Use and Write isset() Correctly
PHP isset()
determines whether a particular variable is set:
It returns a boolean value, which is only True
if all three conditions below are satisfied:
- The variable is declared (has a value assigned).
- This value is not Null.
- The variable hasn't been unset using the
unset()
function.
The syntax for making PHP check if a variable exists with isset()
is simple:
isset($x)
You can use PHP isset()
to check one or multiple variables, thus saving time:
isset($x, $y, $z)
Note: if you check more than one variable, PHP isset will only return True if all of them are set.
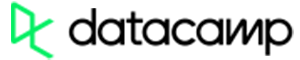
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
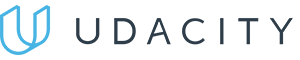
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
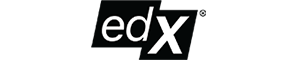
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Checking Variables in PHP: isset vs empty
To check the value of a certain PHP variable, you can also use PHP empty()
. However, you should understand how different it is to isset()
:
The purpose of isset()
and empty()
seem alike and they both return boolean values. However, as you can see in the example, they return opposite values:
isset()
returnsTrue
for set variables.empty()
returnsTrue
for unset or empty ones.
Note: one more difference is that empty() can take expressions as arguments, while isset() cannot.
PHP isset: Summary
- To check if a certain variable is set, you can use PHP
isset()
. - It is not actually a function, but a language construct, similarly as echo and print.
- If checked variable is set, it returns a boolean value of True.
- Variables can be unset with an
unset()
function.