Developers frequently use XML files, but the manipulation of such documents is impossible without the assistance of parser. You are already familiar with DOM parser which is a great choice for small documents.
However, the tree-based structures do not excel in XML parsing huge documents. For that, you should consider an event-based parser, such as Expat XML parser.
Event-based parsers recognize the XML file as sequences of events. We will now introduce you to the lightweight Expat XML parser. It has been around for more than two decades now, and PHP has inbuilt functions to use with it.
Contents
Expat XML Parser: Main Tips
- The PHP XML Parser called Expat allows you to access and manipulate XML data.
- It is inbuilt into PHP.
- Unlike DOM, this is an event-based parser (not tree-based).
XML Format and Data Examples
To get a better understanding on how Expat XML parser works, let's first view a simple XML format example:
<from>Me</from>
As we know, Expat is event-based. That means it reads this data as a series of events:
- Starting element:
<from>
- Starting CDATA block, the value:
Me
- Closing element:
</from>
The sample XML document we are going to use is called note.xml. Let's look at the code we have below:
<?xml version="1.0" encoding="UTF-8"?>
<note>
<to>You</to>
<from>Me</from>
<heading>The Game</heading>
<body>You lost it.</body>
</note>
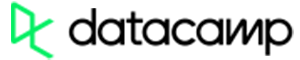
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
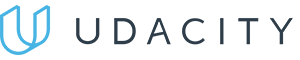
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
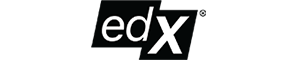
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Initializing the Parser
Now, this piece of code shows us how to initialize the XML Expat Parser using PHP:
<?php
// Initializing the XML Expat parser
$expat_parser = xml_parser_create();
// Declare the function we will use at the beginning of the element
function start($expat_parser,$_name,$_attrs) {
switch($_name) {
case "NOTE":
echo "-- Note --<br>";
break;
case "TO":
echo "Recipient: ";
break;
case "FROM":
echo "Sender: ";
break;
case "HEADING":
echo "Topic: ";
break;
case "BODY":
echo "Letter: ";
}
}
//Declare the function we will use at the ending of the element
function stop($expat_parser, $_name) {
echo "<br>";
}
//Declare the function we will use for finding character data
function char($expat_parser, $data) {
echo $data;
}
// Declare the handler for our elements
xml_set_element_handler($expat_parser, "start", "stop");
// Declare the handler for our data
xml_set_character_data_handler($expat_parser, "char");
// Open the XML file we want to read
$fp = fopen("node.xml", "r");
// Read our data
while ($data=fread($fp,4096)) {
xml_parse($expat_parser, $data, feof($fp)) or die (sprintf("XML Error: %s at line %d", xml_error_string(xml_get_error_code($expat_parser)), xml_get_current_line_number($expat_parser)));
}
// Free/stop using the XML Expat Parser
xml_parser_free($expat_parser);
?>
Expat XML Parser: Summary
- Expat XML Parser comes in handy when handling and modifying documents in XML format. If you are not sure how to read an XML file using parsers, check out our previous tutorial.
- It is an event-based parser that comes inbuilt in PHP.